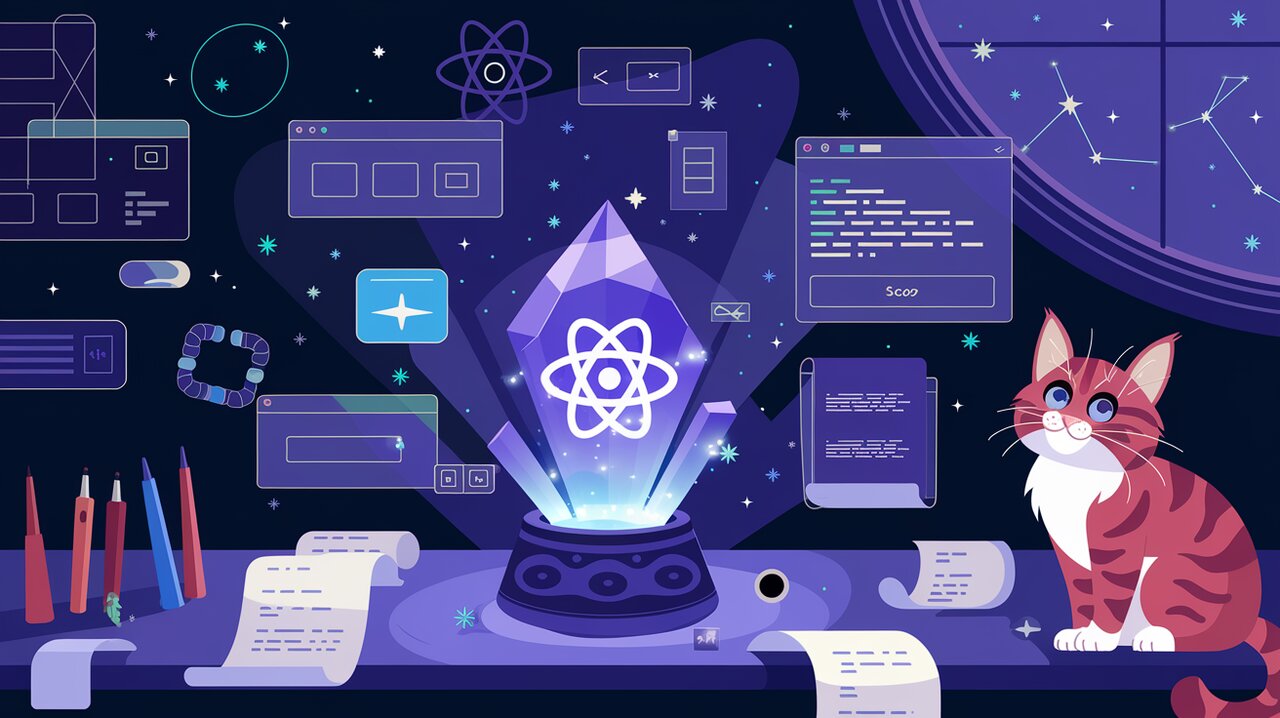
AgnosticUI React: Unleashing UI Wizardry Across Frameworks
AgnosticUI React is a powerful UI component library that brings the concept of framework-agnostic design to the React ecosystem. It offers a set of carefully crafted, accessible, and customizable UI primitives that can be seamlessly integrated into your React projects. With AgnosticUI, you can create consistent and beautiful user interfaces while maintaining the flexibility to use the same components across different frameworks.
Key Features of AgnosticUI React
AgnosticUI React comes packed with features that make it stand out from other UI libraries:
- Framework Flexibility: While we’re focusing on React, the core components are designed to work across multiple frameworks, including Vue 3, Svelte, Astro, and Angular.
- Accessibility First: Built with a strong emphasis on semantic HTML and ARIA attributes, ensuring your applications are usable by everyone.
- Customizable Theming: Leverage CSS custom properties for easy and flexible theming across your entire application.
- Clean and Semantic HTML: The library promotes the use of proper HTML structure, improving SEO and maintainability.
- No Preprocessor Dependencies: Pure CSS is used, eliminating the need for additional build steps or preprocessor configurations.
Getting Started with AgnosticUI React
Let’s dive into how you can start using AgnosticUI React in your projects.
Installation
To begin your journey with AgnosticUI React, you’ll need to install the package and its peer dependencies. Open your terminal and run:
npm install agnostic-react uuid react react-dom
# or if you prefer yarn
yarn add agnostic-react uuid react react-dom
This command installs the agnostic-react
package along with its required peer dependencies[1].
Basic Usage
Once installed, you can start using AgnosticUI React components in your project. Here’s a quick example to get you started:
import React from 'react';
import ReactDOM from 'react-dom';
import 'agnostic-react/dist/common.min.css';
import 'agnostic-react/dist/esm/index.css';
import { Button } from 'agnostic-react';
const App = () => (
<Button mode="primary">Let's get started!</Button>
);
ReactDOM.render(<App />, document.getElementById('root'));
In this example, we import the necessary CSS files and the Button
component from AgnosticUI React. We then create a simple App
component that renders a primary button[2].
Importing Components and Styles
To use AgnosticUI React effectively, you need to import both the common CSS and the component-specific CSS. Here’s how you do it:
import 'agnostic-react/dist/common.min.css';
import 'agnostic-react/dist/esm/index.css';
import { Alert, Button } from 'agnostic-react';
By importing these CSS files, you ensure that your components have the necessary styles to function correctly and look great out of the box[1].
Advanced Usage and Customization
AgnosticUI React offers various ways to customize and extend its components to fit your specific needs.
Theming with CSS Custom Properties
One of the powerful features of AgnosticUI is its use of CSS custom properties for theming. You can easily customize the look and feel of your components by overriding these properties:
:root {
--agnostic-primary: #007bff;
--agnostic-secondary: #6c757d;
--agnostic-font-family: 'Roboto', sans-serif;
}
By setting these custom properties, you can change the primary and secondary colors, as well as the font family used across all AgnosticUI components.
Creating Complex Layouts
AgnosticUI React provides components that can be combined to create complex layouts. Here’s an example using the Grid
and Column
components:
import React from 'react';
import { Grid, Column, Card } from 'agnostic-react';
const ComplexLayout = () => (
<Grid>
<Column xs={12} md={6}>
<Card>
<h2>Left Column</h2>
<p>This content takes up half the width on medium screens and larger.</p>
</Card>
</Column>
<Column xs={12} md={6}>
<Card>
<h2>Right Column</h2>
<p>This content also takes up half the width on medium screens and larger.</p>
</Card>
</Column>
</Grid>
);
This example demonstrates how to create a responsive two-column layout using AgnosticUI components. The Grid
and Column
components work together to create a flexible and responsive structure.
Implementing Forms with Validation
AgnosticUI React can be used to create complex forms with validation. Here’s an example using the Input
and Button
components along with a custom validation hook:
import React, { useState } from 'react';
import { Input, Button } from 'agnostic-react';
const useValidation = (value) => {
const [error, setError] = useState('');
const validate = () => {
if (!value) {
setError('This field is required');
return false;
}
setError('');
return true;
};
return { error, validate };
};
const LoginForm = () => {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const usernameValidation = useValidation(username);
const passwordValidation = useValidation(password);
const handleSubmit = (e) => {
e.preventDefault();
const isUsernameValid = usernameValidation.validate();
const isPasswordValid = passwordValidation.validate();
if (isUsernameValid && isPasswordValid) {
console.log('Form submitted');
}
};
return (
<form onSubmit={handleSubmit}>
<Input
type="text"
value={username}
onChange={(e) => setUsername(e.target.value)}
error={usernameValidation.error}
placeholder="Username"
/>
<Input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
error={passwordValidation.error}
placeholder="Password"
/>
<Button type="submit" mode="primary">Login</Button>
</form>
);
};
This example showcases how to create a login form with validation using AgnosticUI components. The Input
component is used for form fields, while the Button
component is used for submission. Custom validation logic is implemented to provide a smooth user experience.
Conclusion
AgnosticUI React offers a powerful and flexible solution for building user interfaces in React applications. Its framework-agnostic approach, accessibility focus, and customization options make it an excellent choice for developers looking to create consistent and beautiful UIs across different projects and frameworks.
By leveraging AgnosticUI React, you can streamline your development process, ensure accessibility compliance, and maintain a consistent look and feel across your applications. Whether you’re building a simple website or a complex web application, AgnosticUI React provides the tools you need to create stunning user interfaces with ease.
Start exploring the possibilities of AgnosticUI React today and experience the magic of framework-agnostic UI development in your React projects!