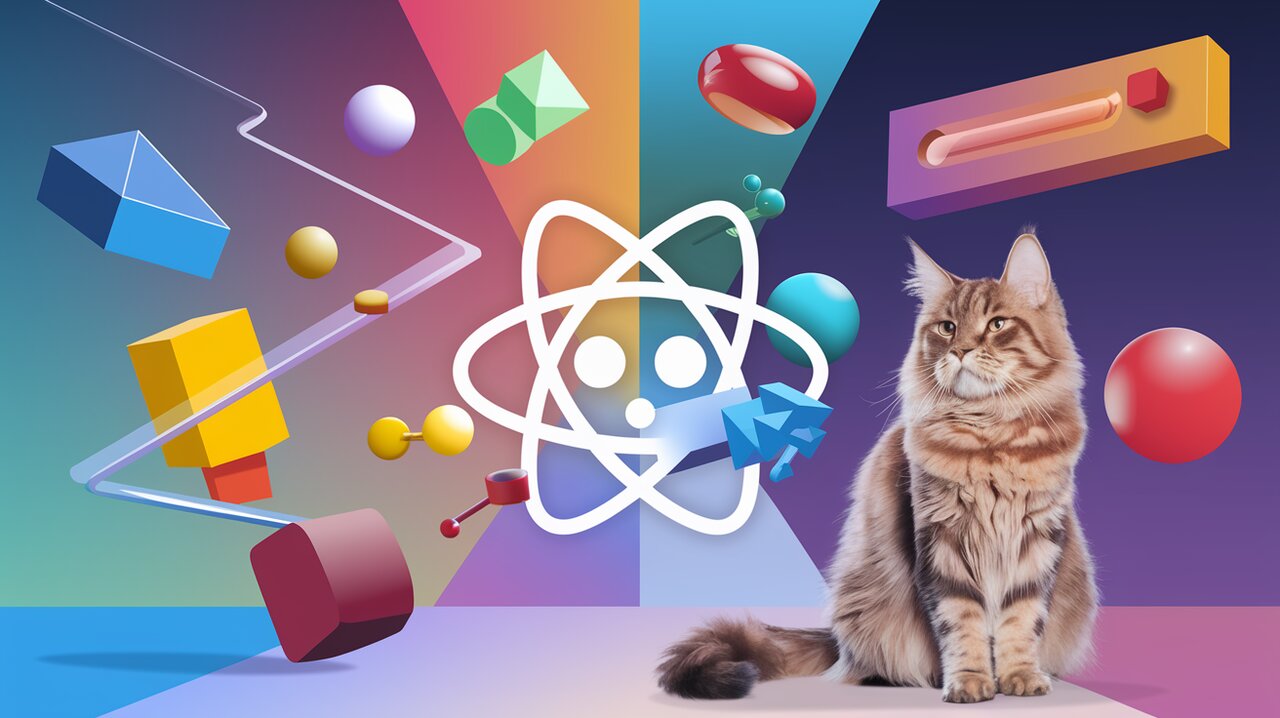
Animate with Ease: Unleashing the Power of react-animatable in React
In the ever-evolving world of web development, creating smooth and engaging animations has become crucial for crafting exceptional user experiences. Enter react-animatable, a lightweight and powerful library that brings the magic of the Web Animations API to your React applications. With its tiny footprint and impressive performance, react-animatable is set to revolutionize how developers approach animations in React.
Unveiling react-animatable’s Arsenal
react-animatable comes packed with features that make it stand out in the crowded field of animation libraries:
- Effortless Integration: With just a few lines of code, you can breathe life into your components, regardless of the UI library or CSS-in-JS solution you’re using.
- Native Performance: Leveraging the Web Animations API, react-animatable ensures your animations run smoothly, tapping into the browser’s native capabilities.
- TypeScript Support: Fully typed with TypeScript, offering a more robust development experience than the standard DOM definitions.
- Compact Size: At just ~1kB gzipped per hook and ~2kB total, it won’t bloat your bundle.
- Versatile Animation Target: Whether it’s HTML, SVG, Canvas, or any other element, react-animatable has got you covered.
Getting Started with react-animatable
Installation
To begin your journey with react-animatable, install it via npm or yarn:
npm install react-animatable
or
yarn add react-animatable
Basic Usage: Your First Animation
Let’s dive into creating a simple rotation animation using react-animatable:
import { useAnimation } from "react-animatable";
const RotatingButton = () => {
const animate = useAnimation(
[
{ transform: "rotate(0deg)" },
{ transform: "rotate(720deg)" }
],
{
duration: 1000,
easing: "ease-in-out",
}
);
return (
<button ref={animate} onClick={() => animate.play()}>
Spin Me!
</button>
);
};
In this example, we define a button that rotates 720 degrees when clicked. The useAnimation
hook sets up our animation keyframes and options, while the ref
attribute and play()
method bring it to life.
Advanced Techniques
Dynamic Keyframes
react-animatable shines when it comes to creating dynamic animations. Here’s how you can animate an element to follow the mouse cursor:
import { useEffect } from "react";
import { useAnimation } from "react-animatable";
const FollowCursor = () => {
const animate = useAnimation<{ x: number; y: number }>(
(prev, args) => [
{ transform: prev.transform },
{ transform: `translate(${args.x}px, ${args.y}px)` },
],
{
duration: 400,
easing: "ease-in-out",
}
);
useEffect(() => {
const onMouseMove = (e: MouseEvent) => {
animate.play({ args: { x: e.clientX, y: e.clientY } });
};
window.addEventListener("mousemove", onMouseMove);
return () => window.removeEventListener("mousemove", onMouseMove);
}, []);
return (
<div
ref={animate}
style={{
position: "fixed",
width: "20px",
height: "20px",
borderRadius: "50%",
backgroundColor: "red",
}}
/>
);
};
This code creates a red dot that smoothly follows your mouse cursor across the screen, demonstrating the power of dynamic keyframe generation.
JavaScript-Only Animations
For scenarios where CSS animations won’t cut it, react-animatable offers the useAnimationFunction
hook:
import { useState, useEffect } from "react";
import { useAnimationFunction } from "react-animatable";
const ProgressBar = () => {
const [progress, setProgress] = useState(0);
const animate = useAnimationFunction<number>(
({ progress }, targetValue) => {
setProgress(progress * targetValue);
},
{
duration: 2000,
easing: "ease-in-out",
}
);
useEffect(() => {
animate.play({ args: 100 });
}, []);
return (
<div style={{ width: "300px", backgroundColor: "#e0e0e0" }}>
<div
style={{
width: `${progress}%`,
height: "20px",
backgroundColor: "#4caf50",
transition: "width 0.3s",
}}
/>
</div>
);
};
This example creates a progress bar that animates from 0 to 100% over 2 seconds, showcasing how useAnimationFunction
can drive non-CSS animations.
Embracing the Future of Web Animations
react-animatable doesn’t just stop at basic animations. It also supports cutting-edge features like ScrollTimeline and ViewTimeline, opening up new possibilities for scroll-driven and view-based animations:
import { useAnimation } from "react-animatable";
const ScrollAnimatedElement = () => {
const animate = useAnimation(
[
{ opacity: 0, transform: "translateY(50px)" },
{ opacity: 1, transform: "translateY(0)" }
],
{
duration: 1000,
easing: "ease-out",
timeline: new ScrollTimeline({
source: document.documentElement,
axis: "block",
}),
}
);
return (
<div ref={animate} style={{ height: "100vh" }}>
Scroll to reveal me!
</div>
);
};
This code creates an element that fades in and slides up as the user scrolls down the page, demonstrating the power of ScrollTimeline integration.
Conclusion
react-animatable brings a fresh approach to animations in React applications. By harnessing the power of the Web Animations API, it offers a performant, lightweight, and easy-to-use solution for creating engaging user interfaces. Whether you’re building simple hover effects or complex, scroll-driven animations, react-animatable provides the tools you need to bring your ideas to life.
As web technologies continue to evolve, libraries like react-animatable will play a crucial role in pushing the boundaries of what’s possible in web animation. By embracing these tools, developers can create more immersive, interactive, and delightful experiences for their users, all while maintaining performance and code simplicity.
So why wait? Dive into react-animatable today and start creating animations that will captivate your users and elevate your React applications to new heights!