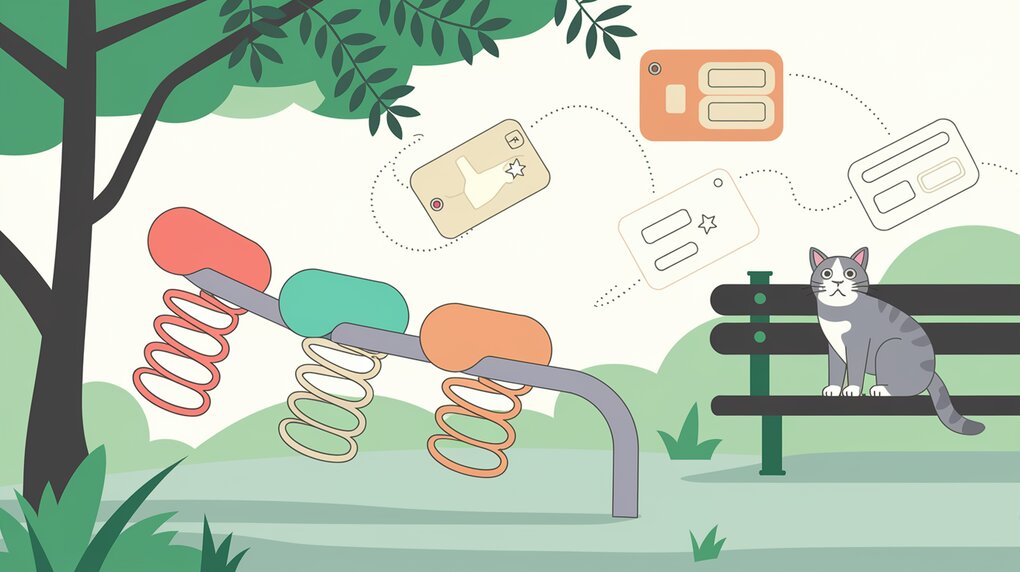
React-spring is a powerful animation library for React applications that leverages spring physics to create fluid, natural-looking animations. Unlike traditional animation libraries that rely on duration and easing functions, react-spring uses a physics-based approach, resulting in more realistic and responsive animations.
Features
React-spring offers a range of features that make it a versatile choice for animating React components:
- Spring Physics: Utilizes real-world physics principles for natural-feeling animations.
- Performance Optimized: Built with performance in mind, ensuring smooth animations even in complex scenarios.
- Declarative API: Allows you to describe animations in a declarative manner, making them easier to understand and maintain.
- Cross-Platform: Works seamlessly across web, React Native, and other React-powered platforms.
- Hooks-based API: Provides a modern, hooks-based approach for creating animations.
- Interpolations: Supports powerful interpolations for transforming values during animations.
- Gesture Support: Integrates well with gesture libraries for interactive animations.
Installation
To get started with react-spring, you can install it using npm or yarn:
Using npm:
npm install react-spring
Using yarn:
yarn add react-spring
Basic Usage
Let’s dive into some basic examples to demonstrate how react-spring works.
Simple Animation
Here’s a simple example of how to create a basic animation using the useSpring
hook:
import React from 'react';
import { useSpring, animated } from 'react-spring';
const AnimatedComponent: React.FC = () => {
const props = useSpring({ opacity: 1, from: { opacity: 0 } });
return <animated.div style={props}>Hello, I'm fading in!</animated.div>;
};
In this example, we’re animating the opacity of a div from 0 to 1, creating a fade-in effect.
Animated Button
Let’s create a button that scales up when hovered:
import React from 'react';
import { useSpring, animated } from 'react-spring';
const AnimatedButton: React.FC = () => {
const [props, set] = useSpring(() => ({ scale: 1 }));
return (
<animated.button
style={{
transform: props.scale.to(s => `scale(${s})`),
}}
onMouseEnter={() => set({ scale: 1.1 })}
onMouseLeave={() => set({ scale: 1 })}
>
Hover me!
</animated.button>
);
};
This button will smoothly scale up to 1.1 times its original size when hovered, and back to normal when the mouse leaves.
Advanced Usage
React-spring’s power truly shines in more complex scenarios. Let’s explore some advanced use cases.
Chained Animations
You can chain multiple animations together using the useChain
hook:
import React from 'react';
import { useSpring, useChain, animated, useSpringRef } from 'react-spring';
const ChainedAnimation: React.FC = () => {
const springRef1 = useSpringRef();
const springRef2 = useSpringRef();
const props1 = useSpring({
ref: springRef1,
from: { opacity: 0 },
to: { opacity: 1 },
});
const props2 = useSpring({
ref: springRef2,
from: { transform: 'scale(0)' },
to: { transform: 'scale(1)' },
});
useChain([springRef1, springRef2]);
return (
<animated.div style={props1}>
<animated.div style={props2}>I fade in, then scale up!</animated.div>
</animated.div>
);
};
This example demonstrates how to chain two animations: first, the outer div fades in, then the inner div scales up.
Gesture-based Animations
React-spring integrates well with gesture libraries like react-use-gesture
. Here’s an example of a draggable component:
import React from 'react';
import { useSpring, animated } from 'react-spring';
import { useDrag } from 'react-use-gesture';
const DraggableComponent: React.FC = () => {
const [{ x, y }, set] = useSpring(() => ({ x: 0, y: 0 }));
const bind = useDrag(({ down, movement: [mx, my] }) => {
set({ x: down ? mx : 0, y: down ? my : 0, immediate: down });
});
return (
<animated.div
{...bind()}
style={{
transform: x.to(x => `translate3d(${x}px, ${y.get()}px, 0)`),
}}
>
Drag me!
</animated.div>
);
};
This component can be dragged around the screen, with the movement being smoothly animated by react-spring.
Interpolation
React-spring allows for powerful interpolations, enabling you to map animated values to different ranges or even to strings:
import React from 'react';
import { useSpring, animated } from 'react-spring';
const InterpolatedAnimation: React.FC = () => {
const { number } = useSpring({
from: { number: 0 },
to: { number: 1 },
config: { duration: 2000 },
});
return (
<animated.div>
{number.to(n => (
<>
<div>Number: {n.toFixed(2)}</div>
<div>Percentage: {(n * 100).toFixed(0)}%</div>
<div style={{ color: n.to(n => `rgb(${n * 255}, 0, 0)`) }}>
Color interpolation
</div>
</>
))}
</animated.div>
);
};
This example shows how a single animated value can be used to drive multiple visual changes, including text content and color.
Conclusion
React-spring offers a powerful and flexible approach to creating animations in React applications. By leveraging spring physics, it provides a more natural and responsive feel to your UI interactions. Whether you’re creating simple fade effects, complex gesture-based animations, or anything in between, react-spring’s declarative API and performance optimizations make it an excellent choice for bringing your React components to life.
As you continue to explore react-spring, you’ll discover even more ways to enhance your user interfaces with fluid, physics-based animations. The library’s extensive documentation and active community provide a wealth of resources for mastering advanced techniques and solving complex animation challenges. Happy animating!