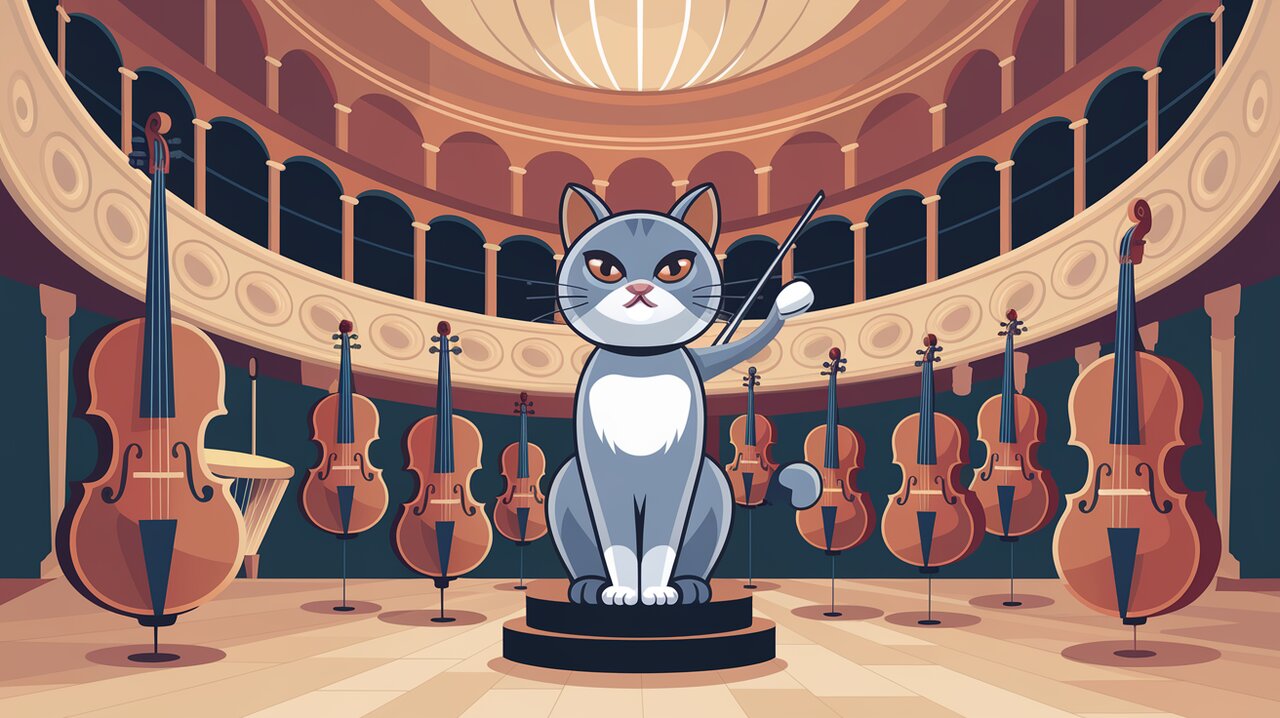
Ant Design: Orchestrating Enterprise-Grade React UI Symphony
Ant Design, affectionately known as antd, is a treasure trove for React developers seeking to build sophisticated, enterprise-grade user interfaces. This powerful UI library offers a harmonious blend of aesthetics and functionality, providing a comprehensive suite of components that work in concert to create stunning web applications.
A Symphony of Features
Ant Design’s repertoire is impressive, offering developers a rich selection of tools and capabilities:
- Enterprise-Class Design: Crafted with large-scale applications in mind, antd provides a consistent and professional look across all components.
- Rich Component Library: From basic buttons to complex data tables, antd offers a wide array of ready-to-use components.
- TypeScript Support: With full TypeScript definitions, developers can enjoy enhanced code intelligence and type safety.
- Customization: The library leverages CSS-in-JS for powerful theme customization, allowing you to tailor the look and feel to your brand.
- Internationalization: Built-in support for dozens of languages makes it easy to create multilingual applications.
- Accessibility: Many components are designed with accessibility in mind, ensuring your application can reach a wider audience.
Setting the Stage
Before we dive into the world of Ant Design, let’s set up our development environment. You can easily add antd to your React project using npm or yarn:
npm install antd
Or if you prefer yarn:
yarn add antd
Basic Usage: The Opening Notes
Let’s start with a simple example to get a feel for how Ant Design components work in harmony:
import React from 'react';
import { Button, DatePicker, Space } from 'antd';
const App: React.FC = () => (
<Space direction="vertical">
<Button type="primary">Click me!</Button>
<DatePicker placeholder="Select a date" />
</Space>
);
export default App;
In this snippet, we’re using the Button
and DatePicker
components, wrapped in a Space
component for easy layout management. The type="primary"
prop on the Button gives it a distinct, eye-catching style, while the DatePicker offers a sleek interface for date selection.
Advanced Compositions: Creating Complex Melodies
Now that we’ve warmed up, let’s explore some more advanced uses of Ant Design components. We’ll create a simple form that demonstrates the power and flexibility of antd:
import React from 'react';
import { Form, Input, Select, Button, message } from 'antd';
const { Option } = Select;
const RegistrationForm: React.FC = () => {
const [form] = Form.useForm();
const onFinish = (values: any) => {
console.log('Received values:', values);
message.success('Registration successful!');
};
return (
<Form form={form} onFinish={onFinish} layout="vertical">
<Form.Item name="name" label="Name" rules={[{ required: true, message: 'Please input your name!' }]}>
<Input />
</Form.Item>
<Form.Item name="email" label="Email" rules={[{ required: true, type: 'email', message: 'Please input a valid email!' }]}>
<Input />
</Form.Item>
<Form.Item name="role" label="Role" rules={[{ required: true, message: 'Please select a role!' }]}>
<Select placeholder="Select a role">
<Option value="developer">Developer</Option>
<Option value="designer">Designer</Option>
<Option value="manager">Manager</Option>
</Select>
</Form.Item>
<Form.Item>
<Button type="primary" htmlType="submit">
Register
</Button>
</Form.Item>
</Form>
);
};
export default RegistrationForm;
This form demonstrates several key features of Ant Design:
- Form Validation: The
rules
prop on Form.Item components allows for easy form validation. - Layout Control: The
layout="vertical"
prop on the Form component controls the overall form layout. - Component Composition: We’re using Input, Select, and Button components within the form, showcasing how different antd components work together seamlessly.
- Feedback: The
message
component provides a simple way to give feedback to the user after form submission.
Customization: Tailoring the Performance
One of Ant Design’s strengths is its customizability. You can easily modify the theme to match your brand’s look and feel. Here’s how you can customize the primary color:
import { ConfigProvider } from 'antd';
const App = () => (
<ConfigProvider
theme={{
token: {
colorPrimary: '#00b96b',
},
}}
>
<YourApp />
</ConfigProvider>
);
This simple configuration changes the primary color across all components, demonstrating the power of Ant Design’s theming system.
Responsive Design: Adapting to Different Stages
Ant Design components are built with responsiveness in mind. Let’s look at how we can create a responsive layout using the Grid system:
import React from 'react';
import { Row, Col, Card } from 'antd';
const ResponsiveLayout: React.FC = () => (
<Row gutter={[16, 16]}>
<Col xs={24} sm={12} md={8} lg={6}>
<Card title="Card 1" extra={<a href="#">More</a>}>
Content for card 1
</Card>
</Col>
<Col xs={24} sm={12} md={8} lg={6}>
<Card title="Card 2" extra={<a href="#">More</a>}>
Content for card 2
</Card>
</Col>
<Col xs={24} sm={12} md={8} lg={6}>
<Card title="Card 3" extra={<a href="#">More</a>}>
Content for card 3
</Card>
</Col>
<Col xs={24} sm={12} md={8} lg={6}>
<Card title="Card 4" extra={<a href="#">More</a>}>
Content for card 4
</Card>
</Col>
</Row>
);
export default ResponsiveLayout;
This layout adjusts gracefully across different screen sizes, showcasing Ant Design’s built-in responsive capabilities.
The Grand Finale
Ant Design offers a comprehensive suite of tools for building sophisticated React applications. Its extensive component library, powerful customization options, and focus on developer experience make it an excellent choice for projects of any scale.
As you continue your journey with Ant Design, you might find these related articles helpful:
These articles explore other popular UI libraries and components that can complement or provide alternatives to Ant Design in your React projects.
With its robust feature set and active community, Ant Design empowers developers to create harmonious, efficient, and visually appealing user interfaces. Whether you’re composing a simple form or orchestrating a complex enterprise application, Ant Design provides the instruments you need to create a true UI symphony.