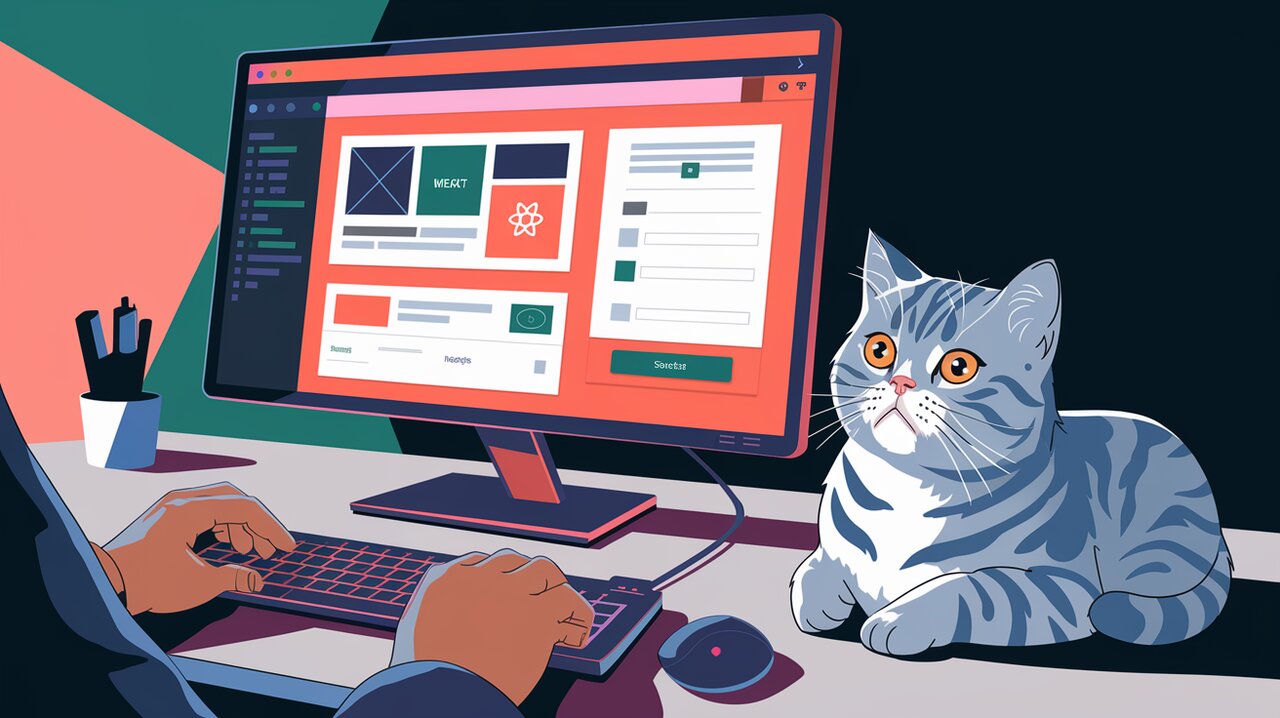
Base Web: Orchestrating React UI with Uber's Design Symphony
Base Web is a powerful React component library that brings Uber’s design system to life. It offers a comprehensive set of modern, responsive, and customizable components that can help developers create stunning user interfaces with ease. Whether you’re building a simple form or a complex dashboard, Base Web provides the tools you need to craft beautiful and functional React applications.
Harmonizing Your UI with Base Web’s Features
Base Web comes packed with a symphony of features that make it stand out in the crowded world of React UI libraries:
- Extensive Component Library: From basic buttons to complex data tables, Base Web offers a wide range of pre-built components that cover most UI needs.
- Customizable Theming: Easily adapt the look and feel of your application with Base Web’s robust theming system.
- Accessibility-First: All components are designed with accessibility in mind, ensuring your app is usable by everyone.
- Responsive Design: Create layouts that adapt seamlessly to different screen sizes and devices.
- TypeScript Support: Enjoy full TypeScript definitions for enhanced development experience and type safety.
- Styletron Integration: Leverage the power of Styletron for efficient CSS-in-JS styling.
Setting the Stage: Installation
To begin your journey with Base Web, you’ll need to install the library and its peer dependencies. You can use either npm or yarn for this purpose:
# Using npm
npm install baseui@next styletron-react styletron-engine-monolithic
# Using yarn
yarn add baseui@next styletron-react styletron-engine-monolithic
Composing Your First Base Web Melody
Let’s start by creating a simple application that showcases how to set up Base Web and use one of its components.
Overture: Basic Setup
First, we need to set up the Base Web provider and Styletron engine:
import React from 'react';
import { Client as Styletron } from 'styletron-engine-monolithic';
import { Provider as StyletronProvider } from 'styletron-react';
import { LightTheme, BaseProvider, styled } from 'baseui';
import { Button } from 'baseui/button';
const engine = new Styletron();
const Centered = styled('div', {
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
height: '100vh',
});
function App() {
return (
<StyletronProvider value={engine}>
<BaseProvider theme={LightTheme}>
<Centered>
<Button>Hello, Base Web!</Button>
</Centered>
</BaseProvider>
</StyletronProvider>
);
}
export default App;
In this example, we’ve set up the necessary providers and created a simple centered layout with a Base Web Button component. The StyletronProvider
and BaseProvider
ensure that our components are properly styled and themed.
Verse: Using Form Components
Base Web excels in form creation. Let’s explore how to use the Input
and FormControl
components to create a simple login form:
import React from 'react';
import { Input } from 'baseui/input';
import { FormControl } from 'baseui/form-control';
import { Button } from 'baseui/button';
function LoginForm() {
return (
<form>
<FormControl label="Username">
<Input placeholder="Enter your username" />
</FormControl>
<FormControl label="Password">
<Input type="password" placeholder="Enter your password" />
</FormControl>
<Button>Log In</Button>
</form>
);
}
This code snippet demonstrates how easy it is to create a structured form using Base Web components. The FormControl
component provides a consistent layout for form fields, including labels and error messages.
Advanced Compositions with Base Web
Now that we’ve covered the basics, let’s explore some more advanced usage of Base Web components.
Chorus: Responsive Layouts with Block
Base Web’s Block
component is a powerful tool for creating responsive layouts. Let’s create a simple responsive grid:
import React from 'react';
import { Block } from 'baseui/block';
import { Card, StyledBody } from 'baseui/card';
function ResponsiveGrid() {
return (
<Block
display="grid"
gridTemplateColumns={['1fr', '1fr 1fr', '1fr 1fr 1fr']}
gridGap="16px"
>
{[1, 2, 3].map((item) => (
<Card key={item}>
<StyledBody>Item {item}</StyledBody>
</Card>
))}
</Block>
);
}
This example creates a grid that adapts from one column on small screens to three columns on larger screens, showcasing the power of Base Web’s responsive design capabilities.
Bridge: Customizing Themes
Base Web allows for deep customization of your application’s look and feel through theming. Here’s how you can create a custom theme:
import { createTheme, lightThemePrimitives } from 'baseui';
const customTheme = createTheme(
{
...lightThemePrimitives,
primary: '#007bff',
primary400: '#4dabf7',
primary500: '#339af0',
},
{
name: 'custom-theme',
}
);
function App() {
return (
<StyletronProvider value={engine}>
<BaseProvider theme={customTheme}>
{/* Your app content */}
</BaseProvider>
</StyletronProvider>
);
}
This example demonstrates how to create a custom theme by overriding specific color values. You can customize various aspects of your theme, including typography, spacing, and component-specific styles.
Finale: Wrapping Up Our Base Web Symphony
Base Web offers a comprehensive suite of React components that can significantly speed up your UI development process. With its focus on accessibility, customization, and responsive design, it’s an excellent choice for building modern web applications.
By leveraging Base Web’s extensive component library, powerful theming system, and integration with Styletron, you can create beautiful, performant, and accessible React applications with ease. Whether you’re building a simple landing page or a complex enterprise application, Base Web provides the tools and flexibility you need to bring your vision to life.
As you continue to explore Base Web, you’ll discover even more advanced features and components that can help you create truly outstanding user interfaces. So dive in, experiment, and let Base Web help you orchestrate your perfect React UI symphony!