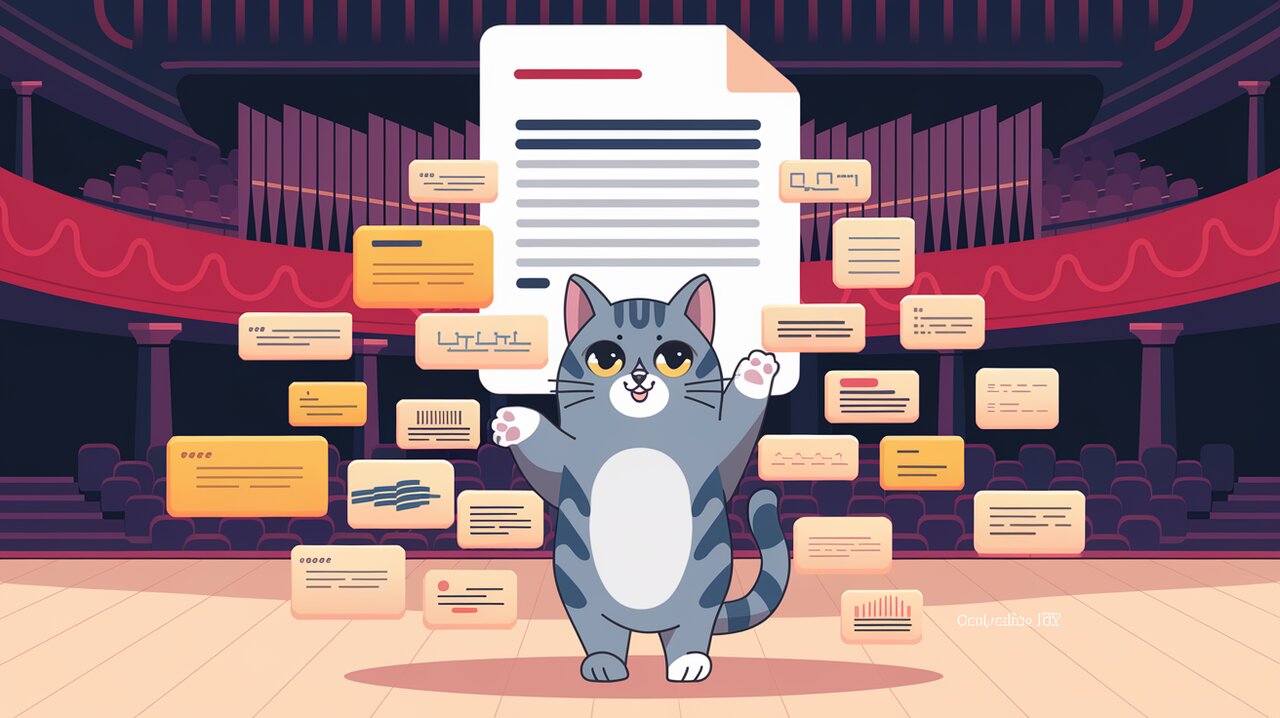
BlockNote: Orchestrating a Text Editing Symphony in React
BlockNote is a cutting-edge, block-based rich text editor designed specifically for React applications. It offers developers a powerful tool to integrate a modern text editing experience into their projects with minimal setup. By organizing documents into blocks, BlockNote provides users with an intuitive way to structure their content while giving developers granular control over document manipulation.
Composing Your Editing Masterpiece
BlockNote comes equipped with a symphony of features that elevate the text editing experience:
- Animated UI: Smooth transitions and effects for a polished user interface.
- Intelligent Placeholders: Helpful prompts guide users through content creation.
- Drag-and-Drop Functionality: Effortlessly reorganize blocks within the document.
- Nested Structures: Create hierarchical content with tab and shift+tab indentation.
- Slash (/) Command Menu: Quick access to formatting options and block types.
- Formatting Toolbar: Easily apply text styles and formatting.
- Real-Time Collaboration: Seamlessly work together on documents in real-time.
Setting the Stage: Installation
To begin your BlockNote journey, you’ll need to install the necessary packages. You can use either npm or yarn to add BlockNote to your project:
Using npm:
npm install @blocknote/react @blocknote/mantine
Using yarn:
yarn add @blocknote/react @blocknote/mantine
The Opening Act: Basic Usage
Let’s start with a simple implementation of BlockNote in your React application:
Creating Your First Editor
import { useCreateBlockNote } from "@blocknote/react";
import { BlockNoteView } from "@blocknote/mantine";
import "@blocknote/core/fonts/inter.css";
import "@blocknote/mantine/style.css";
function App() {
const editor = useCreateBlockNote();
return <BlockNoteView editor={editor} />;
}
This code snippet creates a basic BlockNote editor instance and renders it using the BlockNoteView
component. The useCreateBlockNote
hook initializes the editor, while BlockNoteView
provides the UI.
Customizing the Editor
You can easily customize the editor by passing props to the BlockNoteView
component:
function App() {
const editor = useCreateBlockNote({
initialContent: [
{
type: "paragraph",
content: "Welcome to BlockNote!"
}
]
});
return (
<BlockNoteView
editor={editor}
editable={true}
theme="light"
/>
);
}
This example sets initial content for the editor, ensures it’s editable, and applies a light theme.
The Main Performance: Advanced Usage
Let’s explore some of BlockNote’s more advanced features to truly harness its power.
Handling Editor Events
BlockNote allows you to listen for various events and react accordingly:
function App() {
const editor = useCreateBlockNote();
useEffect(() => {
if (editor) {
editor.onUpdate(() => {
console.log("Editor content updated!");
});
}
}, [editor]);
return <BlockNoteView editor={editor} />;
}
This setup logs a message whenever the editor’s content changes, allowing you to perform actions based on user input.
Custom Block Types
BlockNote supports the creation of custom block types to extend its functionality:
import { BlockNoteSchema, defaultBlockSchema } from "@blocknote/core";
const customSchema = BlockNoteSchema.create({
...defaultBlockSchema,
customBlock: {
type: "customBlock",
propSchema: {
textAlignment: {
default: "left",
},
},
content: "inline",
},
});
function App() {
const editor = useCreateBlockNote({
schema: customSchema,
});
return <BlockNoteView editor={editor} />;
}
This example adds a new custom block type to the editor’s schema, allowing for more specialized content structures.
Implementing File Uploads
BlockNote can be configured to handle file uploads, enhancing the editor’s capabilities:
function App() {
const editor = useCreateBlockNote({
uploadFile: async (file) => {
// Simulated file upload
await new Promise((resolve) => setTimeout(resolve, 1000));
return URL.createObjectURL(file);
},
});
return <BlockNoteView editor={editor} />;
}
This setup adds file upload functionality to the editor, allowing users to insert images and other file types into their documents.
The Grand Finale: Conclusion
BlockNote orchestrates a harmonious blend of powerful features and ease of use, making it an excellent choice for developers looking to implement a modern, block-based rich text editor in their React applications. From basic setups to advanced customizations, BlockNote provides the tools necessary to create a tailored editing experience.
By leveraging its extensive API, event handling capabilities, and customization options, you can compose a text editing symphony that resonates with your users’ needs. Whether you’re building a simple note-taking app or a complex document management system, BlockNote’s flexibility and feature-rich environment make it a standout performer in the world of React text editors.
As you continue to explore BlockNote’s capabilities, you’ll discover even more ways to fine-tune your editing experience, ensuring that your application hits all the right notes with its users.