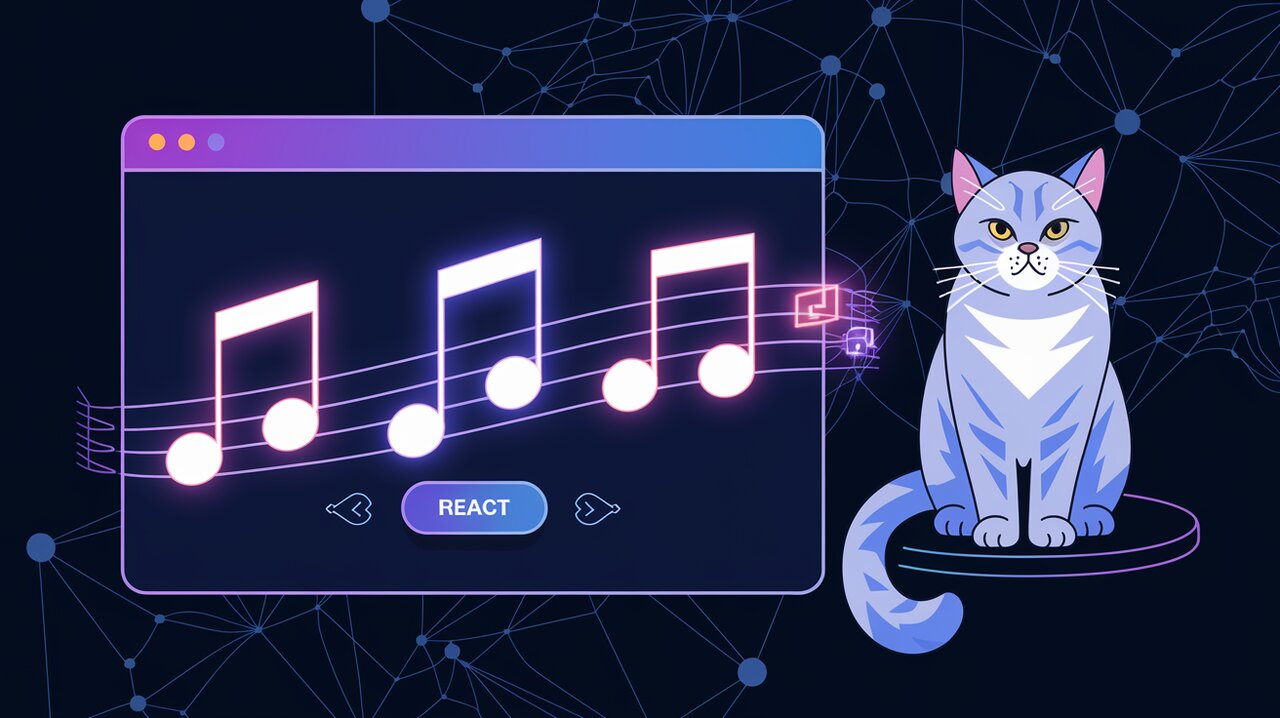
Orchestrating Bluesky Symphonies with react-bluesky-embed
In the ever-evolving landscape of social media integration, developers are constantly seeking elegant solutions to incorporate diverse content into their applications. Enter react-bluesky-embed, a sophisticated React library that orchestrates a seamless symphony of Bluesky posts, profiles, and comments within your React projects. This powerful tool empowers developers to enrich their applications with dynamic social content, fostering user engagement and creating immersive experiences.
Harmonizing Features
react-bluesky-embed comes with a repertoire of features that make it a standout choice for Bluesky integration:
- Effortless embedding of Bluesky post threads
- Support for user profiles and comments (coming soon)
- Customizable themes to match your application’s aesthetic
- Configurable depth for displaying nested replies
- Flexible options to show or hide original posts
Setting the Stage: Installation
Before we dive into the melodious world of Bluesky embedding, let’s set up our development environment. You can install react-bluesky-embed using your preferred package manager:
npm install react-bluesky-embed
# or
yarn add react-bluesky-embed
Composing Your First Bluesky Embed
The Overture: Basic Post Thread Embedding
Let’s start with a simple example of embedding a Bluesky post thread:
import { PostThread } from 'react-bluesky-embed';
const BlueskyEmbed = () => {
return (
<div className="max-w-[672px]">
<PostThread
params={{
did: "did:plc:gru662w3omynujkgwebgeeof",
rkey: "3lbirib5xnc2u",
}}
theme="light"
/>
</div>
);
};
In this example, we’re using the PostThread
component to embed a specific Bluesky post. The params
prop specifies the post’s unique identifiers, while the theme
prop sets the visual style to “light”.
The Crescendo: Customizing Thread Depth
To create a more engaging experience, you might want to display nested replies. Here’s how you can adjust the thread depth:
<PostThread
params={{
did: "did:plc:gru662w3omynujkgwebgeeof",
rkey: "3lbirib5xnc2u",
}}
theme="dark"
config={{
depth: 3,
}}
/>
By setting the depth
in the config
prop, you’re instructing the component to display replies up to three levels deep. This creates a richer conversation view for your users.
Advanced Compositions
The Aria: Focusing on Replies
Sometimes, you might want to highlight the discussion around a post rather than the post itself. react-bluesky-embed allows you to do just that:
<PostThread
params={{
did: "did:plc:gru662w3omynujkgwebgeeof",
rkey: "3lbirib5xnc2u",
}}
theme="dark"
config={{
depth: 6,
}}
hidePost={true}
/>
By setting hidePost
to true
, you’re instructing the component to display only the replies, creating a focused view of the conversation.
The Ensemble: Combining Multiple Threads
For a more complex composition, you might want to display multiple threads side by side:
const BlueskyThreads = () => {
const threads = [
{ did: "did:plc:gru662w3omynujkgwebgeeof", rkey: "3lbirib5xnc2u" },
{ did: "did:plc:another-example-did", rkey: "another-example-rkey" },
];
return (
<div className="flex gap-4">
{threads.map((thread, index) => (
<div key={index} className="w-1/2">
<PostThread
params={thread}
theme={index % 2 === 0 ? "light" : "dark"}
config={{ depth: 2 }}
/>
</div>
))}
</div>
);
};
This example creates a responsive layout with two Bluesky threads side by side, alternating between light and dark themes for visual contrast.
The Finale: Wrapping Up
react-bluesky-embed offers a harmonious solution for integrating Bluesky content into your React applications. Its intuitive API and flexible configuration options allow developers to create rich, interactive social media experiences with minimal effort.
As the library continues to evolve, with upcoming support for profiles and comments, it promises to become an even more powerful tool in the React developer’s repertoire. Whether you’re building a social media dashboard, a content aggregator, or simply want to showcase Bluesky discussions on your website, react-bluesky-embed provides the perfect orchestration of components to bring your vision to life.
By leveraging this library, you’re not just embedding posts; you’re creating a symphony of social interaction within your React applications. As you explore its capabilities, you’ll discover new ways to engage your users and keep them in tune with the latest Bluesky conversations.
For those looking to dive deeper into React component libraries, you might find our articles on Unleashing React Admin Power and Elevating UI React Native Elements to be valuable companions in your development journey.