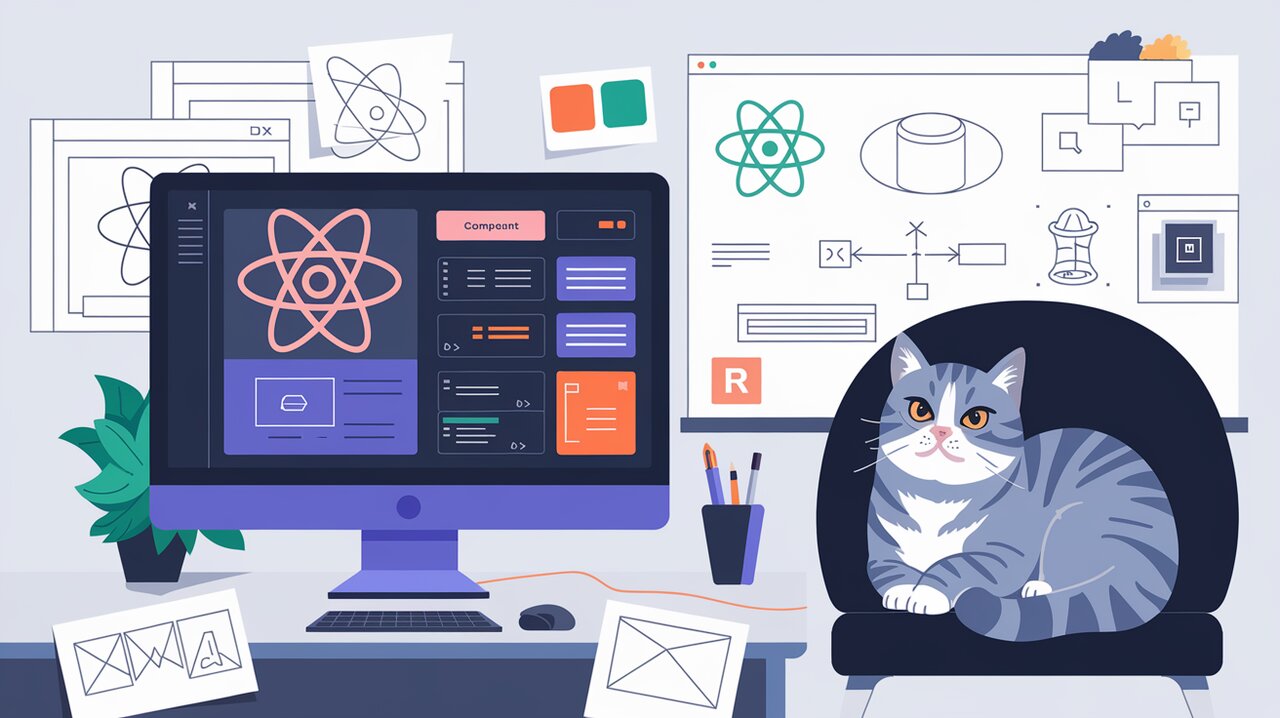
Bootstrapping Your React App: Unleashing the Power of React-Bootstrap
In the ever-evolving world of web development, creating responsive and visually appealing user interfaces has become a crucial aspect of building modern applications. React-Bootstrap emerges as a powerful solution, seamlessly blending the flexibility of React with the robust styling capabilities of Bootstrap. This library empowers developers to craft stunning, responsive web applications with ease, offering a wide array of pre-built components and utilities.
Unveiling the Magic of React-Bootstrap
React-Bootstrap stands out as a comprehensive UI toolkit that reimagines Bootstrap components as true React components. This approach eliminates the need for external dependencies like jQuery, making it a perfect fit for React-based projects. Let’s explore the key features that make React-Bootstrap a go-to choice for many developers:
-
Rich Component Library: React-Bootstrap offers a vast collection of customizable UI components, including buttons, forms, navigation bars, modals, and more.
-
Responsive Design: Built with mobile-first principles, React-Bootstrap ensures your applications look great on devices of all sizes.
-
Accessibility: Components are designed with accessibility in mind, making your applications more inclusive out of the box.
-
Customization: Easily tailor components to match your project’s branding using CSS-in-JS libraries or Sass variables.
-
Performance: By leveraging React’s virtual DOM, React-Bootstrap ensures efficient rendering and updates.
Getting Started with React-Bootstrap
Installation
To begin your journey with React-Bootstrap, you’ll need to install it in your React project. You can do this using npm or yarn:
npm install react-bootstrap bootstrap
or
yarn add react-bootstrap bootstrap
Importing Styles
To ensure your components look as intended, you’ll need to import Bootstrap’s CSS. Add the following line to your main JavaScript file (e.g., index.js
or App.js
):
import 'bootstrap/dist/css/bootstrap.min.css';
Basic Usage: Building Your First Component
Let’s start by creating a simple button component using React-Bootstrap. First, import the Button component:
import Button from 'react-bootstrap/Button';
Now, you can use the Button component in your JSX:
const MyComponent = () => {
return (
<Button variant="primary">Click me!</Button>
);
};
This code snippet creates a primary-styled button with the text “Click me!“. React-Bootstrap’s components are highly customizable, allowing you to easily modify their appearance and behavior.
Creating a Responsive Grid
One of the strengths of Bootstrap is its grid system, and React-Bootstrap makes it even easier to implement. Let’s create a simple responsive layout:
import Container from 'react-bootstrap/Container';
import Row from 'react-bootstrap/Row';
import Col from 'react-bootstrap/Col';
const ResponsiveLayout = () => {
return (
<Container>
<Row>
<Col sm={12} md={6} lg={4}>Column 1</Col>
<Col sm={12} md={6} lg={4}>Column 2</Col>
<Col sm={12} md={12} lg={4}>Column 3</Col>
</Row>
</Container>
);
};
This example creates a responsive layout that adjusts based on the screen size. On small screens, each column takes up the full width, while on larger screens, they arrange themselves side by side.
Advanced Usage: Customizing Components
React-Bootstrap allows for extensive customization of its components. Let’s explore some advanced techniques:
Theming with Sass
To create a custom theme, you can override Bootstrap’s default Sass variables. Create a custom.scss
file:
// custom.scss
$theme-colors: (
"primary": #007bff,
"secondary": #6c757d,
"success": #28a745,
// Add more custom colors here
);
@import "~bootstrap/scss/bootstrap";
Then, import this file in your main JavaScript file instead of the default CSS:
import './custom.scss';
Using the as
Prop
The as
prop allows you to change the underlying HTML element of a React-Bootstrap component. This is particularly useful for creating custom navigation components:
import Nav from 'react-bootstrap/Nav';
import { Link } from 'react-router-dom';
const CustomNav = () => {
return (
<Nav>
<Nav.Item>
<Nav.Link as={Link} to="/">Home</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link as={Link} to="/about">About</Nav.Link>
</Nav.Item>
</Nav>
);
};
In this example, we’re using the as
prop to render React Router’s Link
component instead of a regular anchor tag, allowing for seamless integration with client-side routing.
Creating a Custom Modal
Modals are a common UI pattern, and React-Bootstrap makes them easy to implement and customize:
import { useState } from 'react';
import Modal from 'react-bootstrap/Modal';
import Button from 'react-bootstrap/Button';
const CustomModal = () => {
const [show, setShow] = useState(false);
const handleClose = () => setShow(false);
const handleShow = () => setShow(true);
return (
<>
<Button variant="primary" onClick={handleShow}>
Open Modal
</Button>
<Modal show={show} onHide={handleClose}>
<Modal.Header closeButton>
<Modal.Title>Custom Modal</Modal.Title>
</Modal.Header>
<Modal.Body>This is a customizable modal body.</Modal.Body>
<Modal.Footer>
<Button variant="secondary" onClick={handleClose}>
Close
</Button>
<Button variant="primary" onClick={handleClose}>
Save Changes
</Button>
</Modal.Footer>
</Modal>
</>
);
};
This example demonstrates how to create a modal with custom content and behavior, showcasing the flexibility of React-Bootstrap components.
Conclusion
React-Bootstrap stands as a powerful ally in the quest to create beautiful, responsive web applications. By combining the flexibility of React with the robust styling of Bootstrap, it offers developers a comprehensive toolkit for building modern user interfaces. From basic components to advanced customizations, React-Bootstrap provides the tools necessary to bring your web design visions to life.
As you continue to explore React-Bootstrap, you’ll discover even more ways to enhance your applications. Whether you’re building a simple landing page or a complex web application, React-Bootstrap’s extensive component library and customization options will help you create polished, professional-looking interfaces with ease. Embrace the power of React-Bootstrap, and watch your web development projects soar to new heights of design and functionality.