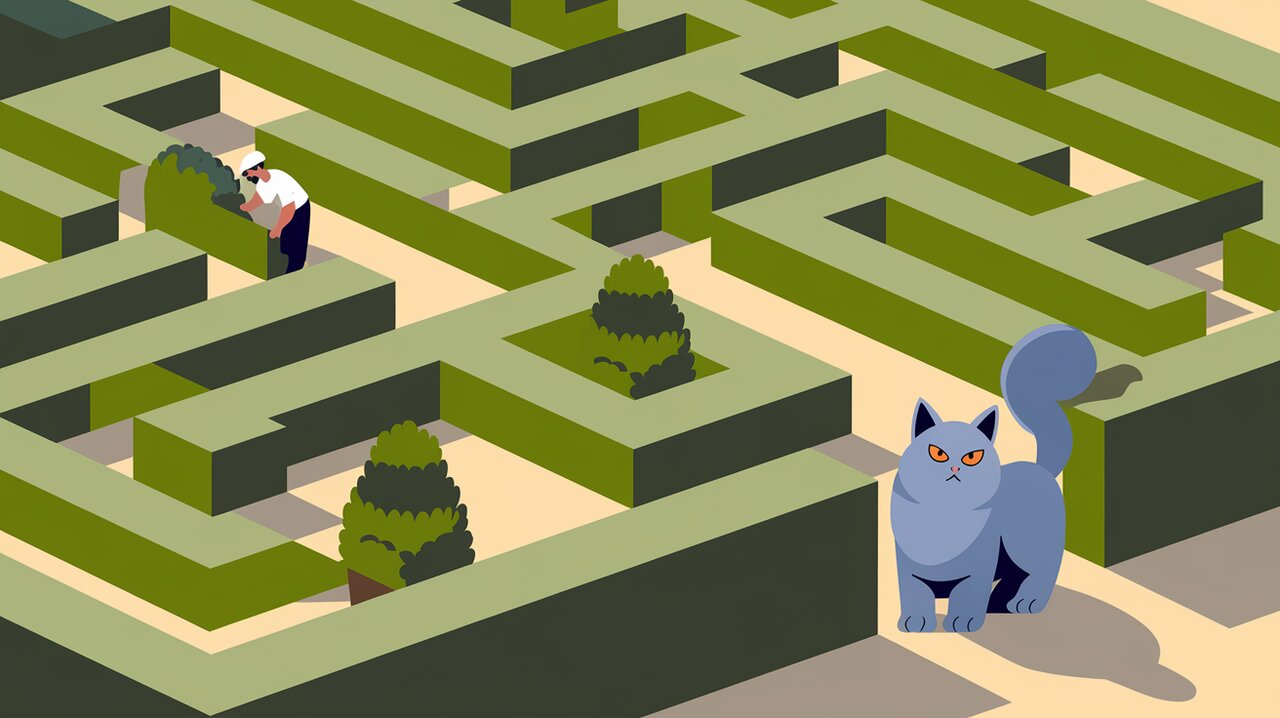
Branching Out: Mastering Dynamic Hierarchies with React DnD TreeView
React DnD TreeView is a powerful and flexible library that brings the concept of interactive, draggable tree structures to life in React applications. This component is designed to handle complex hierarchical data with ease, allowing users to visualize and manipulate tree-like structures through an intuitive drag-and-drop interface. Whether you’re building a file explorer, a category management system, or any application that deals with nested data, React DnD TreeView provides the tools to create a seamless and user-friendly experience.
Branching Out: Key Features of React DnD TreeView
React DnD TreeView comes packed with a variety of features that make it stand out in the forest of React components:
- Drag and Drop Functionality: Effortlessly reorganize tree nodes with smooth drag-and-drop operations.
- Customizable Node Rendering: Tailor the appearance of each node to fit your application’s design.
- Flexible Data Structure: Work with a wide range of hierarchical data formats.
- Performance Optimized: Efficiently handles large trees without compromising on speed.
- Keyboard Accessibility: Navigate and manipulate the tree using keyboard controls.
- Expandable/Collapsible Nodes: Easily manage complex trees by expanding or collapsing parent nodes.
- Multi-select Capability: Select multiple nodes for batch operations.
Planting the Seed: Installation
To start using React DnD TreeView in your project, you’ll need to install it along with its peer dependencies. Open your terminal and run one of the following commands:
Using npm:
npm install @minoru/react-dnd-treeview react-dnd react-dnd-html5-backend
Using yarn:
yarn add @minoru/react-dnd-treeview react-dnd react-dnd-html5-backend
Nurturing Growth: Basic Usage
Let’s start by creating a simple tree structure using React DnD TreeView. First, we’ll set up the basic component and render a tree with some sample data.
Sprouting Your First Tree
import React, { useState } from 'react';
import { Tree } from "@minoru/react-dnd-treeview";
const initialData = [
{
id: 1,
parent: 0,
droppable: true,
text: "Folder 1"
},
{
id: 2,
parent: 1,
text: "File 1-1"
},
{
id: 3,
parent: 1,
text: "File 1-2"
},
{
id: 4,
parent: 0,
droppable: true,
text: "Folder 2"
}
];
const App: React.FC = () => {
const [treeData, setTreeData] = useState(initialData);
const handleDrop = (newTree: any) => setTreeData(newTree);
return (
<Tree
tree={treeData}
rootId={0}
render={(node, { depth, isOpen, onToggle }) => (
<div style={{ marginLeft: depth * 10 }}>
{node.droppable && (
<span onClick={onToggle}>{isOpen ? "[-]" : "[+]"}</span>
)}
{node.text}
</div>
)}
onDrop={handleDrop}
/>
);
};
export default App;
In this example, we’ve created a basic tree structure with folders and files. The Tree
component takes our treeData
state, a rootId
to define the top-level parent, a render
function to customize node appearance, and an onDrop
handler to update the tree when nodes are moved.
Cultivating Custom Nodes
React DnD TreeView allows you to customize the appearance of each node. Let’s enhance our tree by adding icons and styles to differentiate between folders and files.
import React, { useState } from 'react';
import { Tree } from "@minoru/react-dnd-treeview";
import FolderIcon from '@material-ui/icons/Folder';
import InsertDriveFileIcon from '@material-ui/icons/InsertDriveFile';
const CustomNode: React.FC<any> = (props) => {
const { droppable, text } = props.node;
return (
<div style={{ display: 'flex', alignItems: 'center' }}>
{droppable ? <FolderIcon /> : <InsertDriveFileIcon />}
<span style={{ marginLeft: 5 }}>{text}</span>
</div>
);
};
const App: React.FC = () => {
// ... (previous state and handleDrop logic)
return (
<Tree
tree={treeData}
rootId={0}
render={(node, options) => (
<CustomNode node={node} {...options} />
)}
onDrop={handleDrop}
/>
);
};
export default App;
This customization adds visual cues to distinguish between folders and files, enhancing the user experience and making the tree structure more intuitive.
Advanced Techniques: Pruning and Grafting
As your tree grows more complex, you might need to implement more advanced features. Let’s explore some sophisticated uses of React DnD TreeView.
Implementing Multi-select
Enable users to select multiple nodes for batch operations with this enhanced version:
import React, { useState } from 'react';
import { Tree } from "@minoru/react-dnd-treeview";
import Checkbox from '@material-ui/core/Checkbox';
const MultiSelectNode: React.FC<any> = ({ node, depth, isOpen, onToggle, onSelect }) => {
return (
<div style={{ display: 'flex', alignItems: 'center', marginLeft: depth * 10 }}>
<Checkbox
checked={node.selected}
onChange={(e) => onSelect(node.id, e.target.checked)}
/>
{node.droppable && (
<span onClick={onToggle}>{isOpen ? "[-]" : "[+]"}</span>
)}
{node.text}
</div>
);
};
const App: React.FC = () => {
const [treeData, setTreeData] = useState(initialData);
const handleDrop = (newTree: any) => setTreeData(newTree);
const handleSelect = (id: number, selected: boolean) => {
setTreeData(prevData =>
prevData.map(node =>
node.id === id ? { ...node, selected } : node
)
);
};
return (
<Tree
tree={treeData}
rootId={0}
render={(node, options) => (
<MultiSelectNode
node={node}
{...options}
onSelect={handleSelect}
/>
)}
onDrop={handleDrop}
/>
);
};
export default App;
This implementation adds checkboxes to each node, allowing users to select multiple items simultaneously.
Conditional Dropping
Sometimes, you may want to restrict where items can be dropped. Here’s how to implement conditional dropping:
import React, { useState } from 'react';
import { Tree } from "@minoru/react-dnd-treeview";
const App: React.FC = () => {
const [treeData, setTreeData] = useState(initialData);
const handleDrop = (newTree: any, options: any) => {
const { dragSource, dropTarget } = options;
// Only allow dropping files into folders
if (dragSource.droppable || !dropTarget.droppable) {
return;
}
setTreeData(newTree);
};
const canDrop = (tree: any, options: any) => {
const { dragSource, dropTarget } = options;
return !dragSource.droppable && dropTarget.droppable;
};
return (
<Tree
tree={treeData}
rootId={0}
render={(node, options) => (
<div>{node.text}</div>
)}
onDrop={handleDrop}
canDrop={canDrop}
/>
);
};
export default App;
This example demonstrates how to use the canDrop
prop to define rules for where nodes can be dropped, ensuring that only files can be moved into folders.
Harvesting the Fruits: Conclusion
React DnD TreeView offers a robust solution for creating interactive and flexible tree structures in React applications. From basic implementations to advanced features like multi-select and conditional dropping, this library provides the tools needed to build sophisticated hierarchical interfaces.
By leveraging the power of React DnD TreeView, developers can create intuitive, drag-and-drop enabled tree components that enhance user interaction with complex data structures. Whether you’re building a file system explorer, a category management tool, or any application that deals with nested data, React DnD TreeView proves to be an invaluable asset in your React toolkit.
As you continue to explore and implement React DnD TreeView in your projects, remember that the key to creating effective tree structures lies in understanding your data and user needs. Experiment with different rendering styles, implement custom logic for node operations, and always prioritize user experience in your designs.
For more insights on working with complex UI components in React, you might find our articles on Branching Out: React TreeView and Cultivating Dynamic UIs with React Arborist helpful in expanding your knowledge of tree-based interfaces.