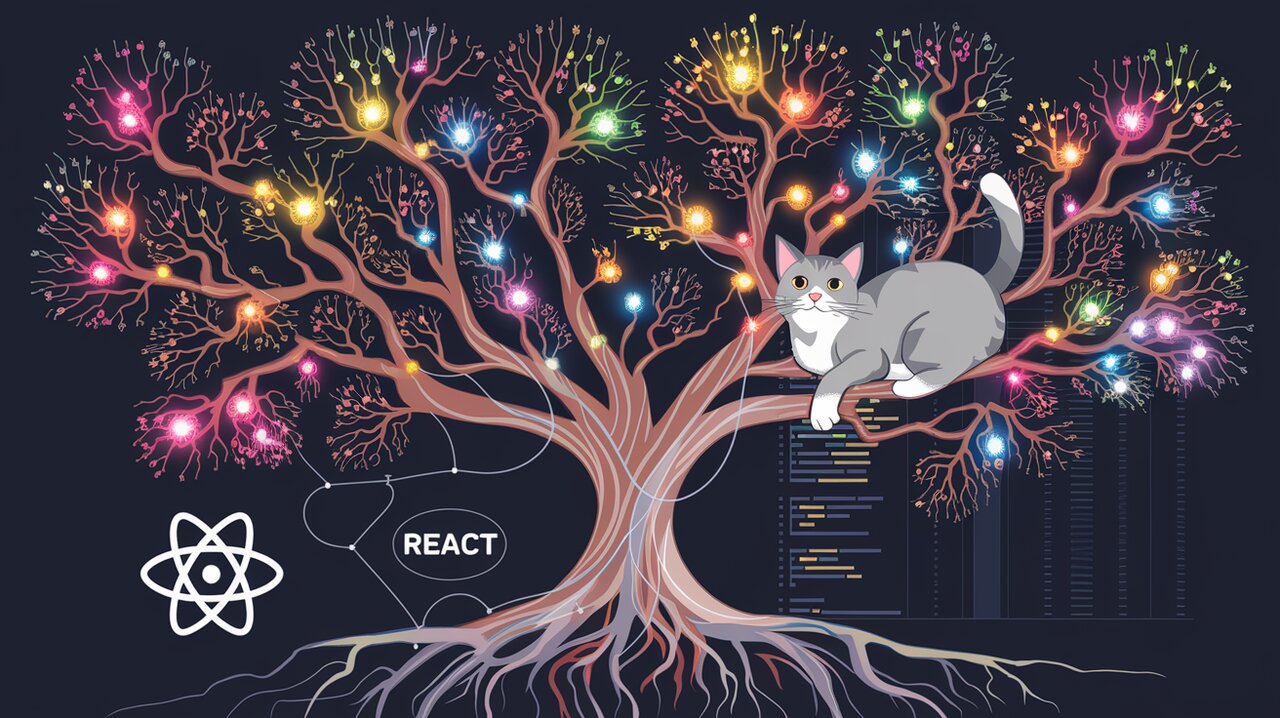
Branching Out: Visualizing Hierarchies with react-tree
React Tree is a powerful yet lightweight React component that allows developers to create beautiful tree visualizations from nested data structures. Unlike many tree visualization libraries that rely on D3.js, react-tree is a pure React implementation, making it easier to integrate into existing React projects without additional dependencies.
Features
- Pure React Implementation: No D3.js dependency required
- Flexible Orientation: Supports both vertical and horizontal tree layouts
- Customizable: Easily style nodes and connections to match your application’s design
- Performance: Efficiently renders large tree structures
- TypeScript Support: Includes TypeScript definitions for type-safe development
Installation
To get started with react-tree
, you can install it via npm or yarn:
npm install react-tree
or
yarn add react-tree
Basic Usage
Creating a Simple Tree
Let’s start by creating a basic vertical tree using react-tree
. First, we’ll define our tree data structure:
const treeData = [
{
name: "Root",
id: "root",
children: [
{
name: "Branch 1",
id: "branch1",
children: [
{ name: "Leaf 1", id: "leaf1" },
{ name: "Leaf 2", id: "leaf2" }
]
},
{
name: "Branch 2",
id: "branch2",
children: [
{ name: "Leaf 3", id: "leaf3" }
]
}
]
}
];
Now, let’s render this tree using the Tree
component from react-tree
:
import React from 'react';
import { Tree } from 'react-tree';
const MyTreeComponent: React.FC = () => {
return (
<div style={{ width: '100%', height: '400px' }}>
<Tree
data={treeData}
orientation="vertical"
nodeRadius={5}
margins={{ top: 20, bottom: 20, left: 20, right: 20 }}
/>
</div>
);
};
export default MyTreeComponent;
In this example, we’re creating a vertical tree with circular nodes of radius 5 pixels. The margins
prop ensures that the tree doesn’t touch the edges of its container.
Customizing Node Appearance
React Tree allows you to customize the appearance of nodes. Let’s modify our previous example to use different colors for different levels of the tree:
import React from 'react';
import { Tree } from 'react-tree';
const MyCustomTreeComponent: React.FC = () => {
const customNodeStyle = (node: any) => {
const level = node.depth;
const colors = ['#6b5b95', '#feb236', '#d64161'];
return {
circle: { fill: colors[level % colors.length] },
name: { fill: 'white' }
};
};
return (
<div style={{ width: '100%', height: '400px' }}>
<Tree
data={treeData}
orientation="vertical"
nodeRadius={8}
margins={{ top: 20, bottom: 20, left: 20, right: 20 }}
nodeStyle={customNodeStyle}
/>
</div>
);
};
export default MyCustomTreeComponent;
This customization applies different colors to nodes based on their depth in the tree, creating a visually appealing hierarchy.
Advanced Usage
Creating an Interactive Tree
Let’s create a more interactive tree where clicking on a node expands or collapses its children:
import React, { useState } from 'react';
import { Tree } from 'react-tree';
const InteractiveTreeComponent: React.FC = () => {
const [expandedNodes, setExpandedNodes] = useState<Set<string>>(new Set(['root']));
const toggleNode = (nodeId: string) => {
setExpandedNodes(prev => {
const newSet = new Set(prev);
if (newSet.has(nodeId)) {
newSet.delete(nodeId);
} else {
newSet.add(nodeId);
}
return newSet;
});
};
const customNodeStyle = (node: any) => ({
circle: { fill: expandedNodes.has(node.id) ? '#4CAF50' : '#2196F3' },
name: { fill: 'white' }
});
return (
<div style={{ width: '100%', height: '400px' }}>
<Tree
data={treeData}
orientation="horizontal"
nodeRadius={10}
margins={{ top: 20, bottom: 20, left: 40, right: 120 }}
nodeStyle={customNodeStyle}
onClick={(node) => toggleNode(node.id)}
expanded={Array.from(expandedNodes)}
/>
</div>
);
};
export default InteractiveTreeComponent;
This example creates a horizontal tree where nodes can be expanded or collapsed by clicking. The expanded
prop controls which nodes are expanded, and the onClick
handler updates this state.
Implementing Custom Node Content
React Tree allows you to completely customize the content of each node. Let’s create a tree with custom node content that includes an icon and additional information:
import React from 'react';
import { Tree } from 'react-tree';
const CustomNodeTreeComponent: React.FC = () => {
const renderCustomNode = (node: React.ReactNode) => (
<g>
<circle r={20} fill="#f3f3f3" stroke="#2196F3" strokeWidth={2} />
<text dy=".35em" textAnchor="middle" style={{ fontSize: 12 }}>
{node.name}
</text>
<text dy="1.2em" textAnchor="middle" style={{ fontSize: 10, fill: '#666' }}>
ID: {node.id}
</text>
</g>
);
return (
<div style={{ width: '100%', height: '600px' }}>
<Tree
data={treeData}
orientation="vertical"
margins={{ top: 40, bottom: 40, left: 120, right: 120 }}
renderCustomNode={renderCustomNode}
nodeSize={{ x: 100, y: 100 }}
/>
</div>
);
};
export default CustomNodeTreeComponent;
In this example, we’re using the renderCustomNode
prop to provide a custom rendering function for each node. This function returns SVG elements that create a circular node with the node’s name and ID.
Conclusion
React Tree offers a flexible and powerful way to visualize hierarchical data in React applications. Its pure React implementation makes it easy to integrate and customize, while its support for both vertical and horizontal layouts provides versatility for different use cases. Whether you’re building a file system explorer, an organizational chart, or any other hierarchical data visualization, react-tree
provides the tools you need to create beautiful and interactive tree structures.
By leveraging react-tree
’s customization options, you can create tree visualizations that not only represent your data accurately but also match your application’s design language. As you continue to explore react-tree, you’ll discover even more ways to enhance your tree visualizations, making your React applications more informative and engaging for users.