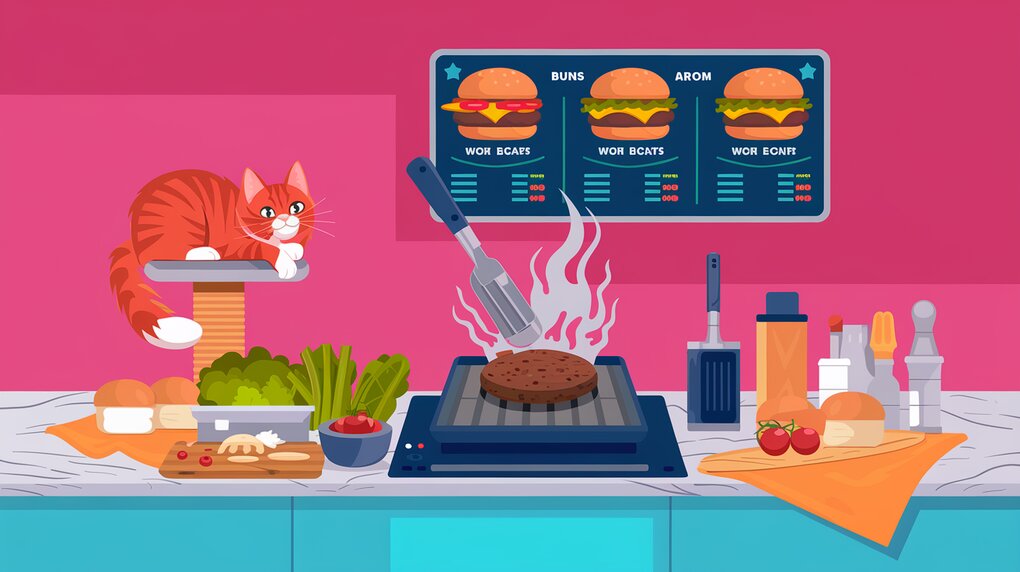
Burger Up Your React App: Sizzling Sidebars with react-burger-menu
React-burger-menu is a powerful and flexible React component that allows developers to create off-canvas sidebars with a variety of eye-catching animations. This library is perfect for adding responsive navigation menus to your web applications, providing a smooth and engaging user experience.
Features
- Multiple Animation Styles: Choose from a range of pre-built animations to suit your design needs.
- Customizable: Easily adjust the appearance and behavior of the menu to match your application’s style.
- Responsive: Works seamlessly across different screen sizes and devices.
- Accessibility: Supports keyboard navigation and screen readers for improved accessibility.
- Redux Integration: Can be easily integrated with Redux for state management (via a separate package).
Installation
To get started with react-burger-menu, you’ll need to install it in your React project. The library is available on npm and can be installed using either npm or yarn.
Using npm:
npm install react-burger-menu --save
Using yarn:
yarn add react-burger-menu
Basic Usage
Let’s dive into how you can implement a basic sidebar menu using react-burger-menu. First, you’ll need to import the component and choose an animation style.
import React from 'react';
import { slide as Menu } from 'react-burger-menu';
const Sidebar: React.FC = () => {
return (
<Menu>
<a className="menu-item" href="/">
Home
</a>
<a className="menu-item" href="/about">
About
</a>
<a className="menu-item" href="/services">
Services
</a>
<a className="menu-item" href="/contact">
Contact
</a>
</Menu>
);
};
export default Sidebar;
In this example, we’re using the ‘slide’ animation. The Menu component wraps our navigation items, which are simple anchor tags. You can replace these with your preferred navigation components, such as React Router’s Link component.
To make the menu functional, you’ll need to add some basic styles. React-burger-menu uses inline styles by default, but you can override these with your own CSS for more control.
import React from 'react';
import { slide as Menu } from 'react-burger-menu';
const styles = {
bmBurgerButton: {
position: 'fixed',
width: '36px',
height: '30px',
left: '36px',
top: '36px'
},
bmBurgerBars: {
background: '#373a47'
},
bmMenuWrap: {
position: 'fixed',
height: '100%'
},
bmMenu: {
background: '#373a47',
padding: '2.5em 1.5em 0',
fontSize: '1.15em'
},
bmItemList: {
color: '#b8b7ad',
padding: '0.8em'
},
bmItem: {
display: 'inline-block'
},
bmOverlay: {
background: 'rgba(0, 0, 0, 0.3)'
}
};
const Sidebar: React.FC = () => {
return (
<Menu styles={styles}>
{/* Menu items */}
</Menu>
);
};
export default Sidebar;
This will give you a basic styled sidebar that slides in from the left when the burger icon is clicked.
Advanced Usage
React-burger-menu offers several props to customize the behavior and appearance of your sidebar. Let’s explore some of these advanced features.
Customizing the Animation
You can easily switch between different animation styles by changing the import statement. For example, to use the ‘push’ animation:
import { push as Menu } from 'react-burger-menu';
Available animations include: slide, stack, elastic, bubble, push, pushRotate, scaleDown, scaleRotate, fallDown, and reveal.
Controlling the Menu State
You can control the open/closed state of the menu programmatically using the isOpen
prop and the onStateChange
callback.
import React, { useState } from 'react';
import { slide as Menu } from 'react-burger-menu';
const Sidebar: React.FC = () => {
const [menuOpen, setMenuOpen] = useState(false);
const handleStateChange = (state: { isOpen: boolean }) => {
setMenuOpen(state.isOpen);
};
const closeMenu = () => {
setMenuOpen(false);
};
return (
<Menu
isOpen={menuOpen}
onStateChange={handleStateChange}
>
<a className="menu-item" href="/" onClick={closeMenu}>
Home
</a>
{/* Other menu items */}
</Menu>
);
};
export default Sidebar;
This setup allows you to control the menu state from outside the component and close the menu when a link is clicked.
Customizing the Burger Icon
You can replace the default burger icon with your own custom icon:
import React from 'react';
import { slide as Menu } from 'react-burger-menu';
const Sidebar: React.FC = () => {
return (
<Menu customBurgerIcon={<img src="path/to/your/icon.png" />}>
{/* Menu items */}
</Menu>
);
};
export default Sidebar;
Right-aligned Menu
To position the menu on the right side of the screen, use the right
prop:
<Menu right>
{/* Menu items */}
</Menu>
Disabling Overlay
If you don’t want the overlay that appears behind the menu when it’s open, you can disable it:
<Menu disableOverlayClick>
{/* Menu items */}
</Menu>
Conclusion
React-burger-menu is a versatile and powerful library for creating animated sidebar menus in React applications. With its variety of animation styles and customization options, you can easily create a unique and engaging navigation experience for your users. Whether you’re building a simple blog or a complex web application, react-burger-menu provides the flexibility and functionality to enhance your project’s UI.
Remember to consider accessibility when implementing your menu, and always test your implementation across different devices and screen sizes to ensure a consistent user experience. Happy coding, and may your sidebars always be smooth and your burgers always be juicy!