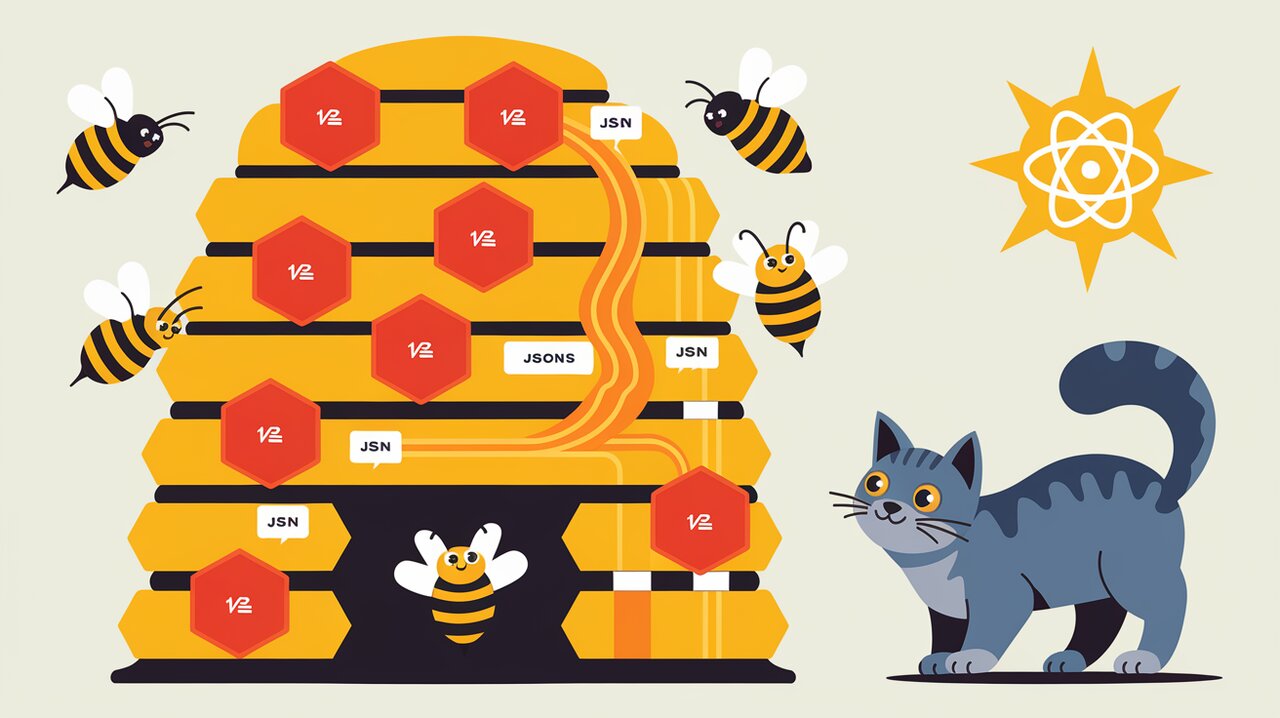
Buzzing with Redux-Bees: A Sweet Solution for JSON API Calls in Redux
In the world of React and Redux applications, managing API calls can often become a complex and tedious task. Enter Redux-Bees, a library that aims to simplify this process by providing a declarative way to handle JSON API calls within your Redux ecosystem. By offering a structured approach to API integration, Redux-Bees helps developers create more maintainable and efficient React applications.
The Buzz About Redux-Bees
Redux-Bees brings several key features to the table that make it stand out in the crowded field of API management libraries:
- Declarative API Definitions: Define your API endpoints in a clear, structured manner.
- Automatic Request Deduplication: Avoid redundant API calls by automatically combining identical requests.
- Redux Integration: Seamlessly integrate with your existing Redux store.
- Normalized State Management: Efficiently manage API responses in a normalized Redux state.
- React Integration: Easily connect your React components to API data with higher-order components.
- Conditional Fetching: Control when and how data is fetched based on component props.
- Server-Side Rendering Support: Facilitate data loading in server-side rendered applications.
Setting Up Your Hive
To get started with Redux-Bees, you’ll need to install it in your project. You can do this using npm or yarn:
npm install redux-bees --save
Or if you prefer yarn:
yarn add redux-bees
Building Your API Honeycomb
Defining API Endpoints
The first step in using Redux-Bees is to define your API endpoints. This is done in a declarative manner, making it easy to understand and maintain your API structure:
import { buildApi, get, post, patch, destroy } from 'redux-bees';
const apiEndpoints = {
getPosts: { method: get, path: '/posts' },
getPost: { method: get, path: '/posts/:id' },
createPost: { method: post, path: '/posts' },
updatePost: { method: patch, path: '/posts/:id' },
destroyPost: { method: destroy, path: '/posts/:id' },
getCategory: { method: get, path: '/categories/:id' },
getComments: { method: get, path: '/posts/:postId/relationships/comments' },
createComment: { method: post, path: '/posts/:postId/relationships/comments' },
};
const config = {
baseUrl: 'https://api.yourservice.com'
};
const api = buildApi(apiEndpoints, config);
This setup creates a structured representation of your API, making it easy to use throughout your application. The buildApi
function takes your endpoint definitions and configuration, returning an object with methods corresponding to each endpoint.
Integrating with Redux
To integrate Redux-Bees with your Redux store, you need to add its reducer and middleware:
import { createStore, applyMiddleware, compose, combineReducers } from 'redux';
import { reducer as beesReducer, middleware as beesMiddleware } from 'redux-bees';
const reducer = combineReducers({
// ...your other reducers
bees: beesReducer,
});
const store = createStore(
reducer,
applyMiddleware(beesMiddleware())
);
This setup ensures that Redux-Bees can manage API-related state within your Redux store.
Harvesting Data with Redux-Bees
Making API Calls
With your API defined and Redux integrated, you can now make API calls using the methods provided by Redux-Bees:
api.getPosts()
.then((result) => {
console.log(result.body.data);
})
.catch((error) => {
console.error(error);
});
Redux-Bees handles the API call and automatically updates your Redux store with the results.
Accessing API Data in Components
Redux-Bees provides a query
higher-order component to easily connect your React components to API data:
import React from 'react';
import { query } from 'redux-bees';
interface PostsProps {
posts: any[];
status: {
posts: {
isLoading: boolean;
hasFailed: boolean;
error: any;
}
};
}
@query('posts', api.getPosts)
class PostsList extends React.Component<PostsProps> {
render() {
const { posts, status } = this.props;
if (status.posts.isLoading) {
return <div>Loading posts...</div>;
}
if (status.posts.hasFailed) {
return <div>Error: {status.posts.error}</div>;
}
return (
<ul>
{posts.map(post => (
<li key={post.id}>{post.attributes.title}</li>
))}
</ul>
);
}
}
This component will automatically fetch posts when mounted and re-render as the data becomes available.
Advanced Beekeeping Techniques
Conditional Fetching
Sometimes you only want to fetch data under certain conditions. Redux-Bees allows for this with its query
HOC:
@query('post', api.getPost, (perform, props) => (
perform({ id: props.match.params.id })
))
class PostDetail extends React.Component {
// Component implementation
}
In this example, the post is only fetched when the id
parameter is available in the component’s props.
Cache Invalidation
After modifying data, you often need to invalidate previously fetched data. Redux-Bees provides an invalidateRequests
action for this purpose:
import { invalidateRequests } from 'redux-bees';
// After creating a new post
dispatch(api.createPost({ /* post data */ }))
.then(() => {
dispatch(invalidateRequests(api.getPosts));
});
This ensures that the next time getPosts
is called, it will fetch fresh data from the server.
Server-Side Rendering
Redux-Bees supports server-side rendering by exposing a loadData
static method on components wrapped with the query
HOC:
import { matchRoutes } from 'react-router-config';
const routes = [
{ component: PostsList, path: '/posts' },
// other routes...
];
server.get('*', (req, res) => {
const store = createStore(/* your store configuration */);
const branch = matchRoutes(routes, req.url);
const promises = branch.map(({ route }) => {
return route.component.loadData ?
route.component.loadData(store.dispatch) :
Promise.resolve(null);
});
Promise.all(promises).then(() => {
// Render your app with the pre-loaded state
});
});
This approach allows you to pre-fetch necessary data on the server before rendering your React application.
Wrapping Up the Hive
Redux-Bees offers a structured and efficient approach to managing API calls in React applications using Redux. By providing a declarative API definition, automatic request deduplication, and easy integration with React components, it simplifies the often complex task of data fetching and state management.
Whether you’re building a small application or a large-scale project, Redux-Bees can help you maintain a clean and organized codebase while efficiently managing your API interactions. Its support for advanced features like conditional fetching and server-side rendering makes it a versatile tool for a wide range of React applications.
As you continue to explore the capabilities of Redux-Bees, you might also find it helpful to look into related topics such as state management with Jotai or data fetching with React Query. These complementary approaches can further enhance your React development toolkit, allowing you to build even more powerful and efficient applications.