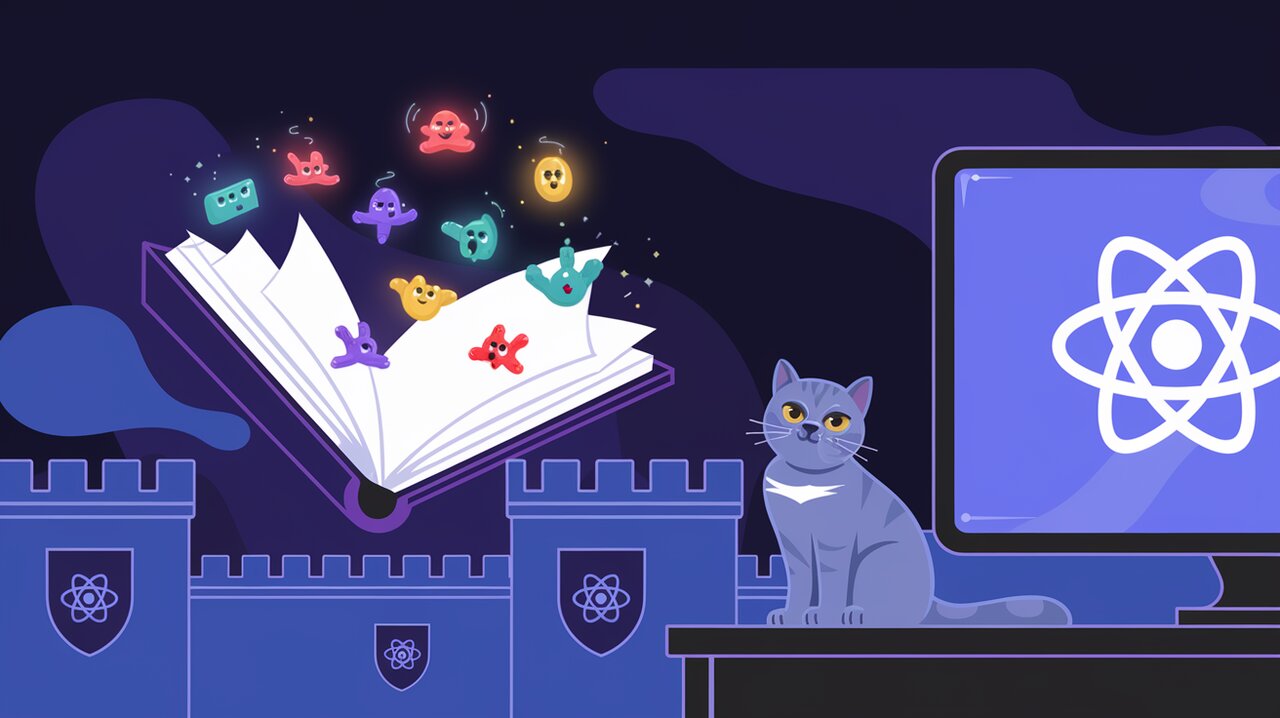
Captcha Conjuring: Unleashing the Magic of react-simple-captcha
React Simple Captcha is a powerful and highly customizable captcha solution designed specifically for React applications. It offers developers an easy way to implement robust security measures, protecting forms and sensitive actions from automated bot attacks. In this article, we’ll explore the features, installation process, and usage of react-simple-captcha, empowering you to enhance your application’s security with minimal effort.
Key Features
React Simple Captcha comes packed with a variety of features that make it stand out:
- Easy integration with React applications
- Highly customizable appearance and behavior
- Support for both reloadable and non-reloadable captchas
- Flexible validation options
- Customizable character set and length
Installation
To get started with React Simple Captcha, you’ll need to install it in your project. You can do this using either npm or yarn:
npm install react-simple-captcha
or
yarn add react-simple-captcha
Basic Usage
Let’s dive into the basic usage of React Simple Captcha. We’ll cover the essential steps to integrate the captcha into your React application.
Importing Required Functions
First, you need to import the necessary functions from the react-simple-captcha package:
import {
loadCaptchaEnginge,
LoadCanvasTemplate,
LoadCanvasTemplateNoReload,
validateCaptcha
} from 'react-simple-captcha';
Rendering the Captcha
To display the captcha in your component, you can use either LoadCanvasTemplate
or LoadCanvasTemplateNoReload
. The former includes a reload button, while the latter does not:
import React from 'react';
import { LoadCanvasTemplate } from 'react-simple-captcha';
const CaptchaComponent: React.FC = () => {
return (
<div>
<LoadCanvasTemplate />
</div>
);
};
export default CaptchaComponent;
Initializing the Captcha Engine
To generate the captcha, you need to initialize the captcha engine in the componentDidMount
lifecycle method or in a useEffect
hook:
import React, { useEffect } from 'react';
import { loadCaptchaEnginge, LoadCanvasTemplate } from 'react-simple-captcha';
const CaptchaComponent: React.FC = () => {
useEffect(() => {
loadCaptchaEnginge(6);
}, []);
return (
<div>
<LoadCanvasTemplate />
</div>
);
};
export default CaptchaComponent;
Validating User Input
To validate the user’s input against the generated captcha, you can use the validateCaptcha
function:
import React, { useEffect, useState } from 'react';
import { loadCaptchaEnginge, LoadCanvasTemplate, validateCaptcha } from 'react-simple-captcha';
const CaptchaComponent: React.FC = () => {
const [userInput, setUserInput] = useState('');
useEffect(() => {
loadCaptchaEnginge(6);
}, []);
const handleSubmit = () => {
if (validateCaptcha(userInput) === true) {
alert('Captcha Matched');
} else {
alert('Captcha Does Not Match');
}
};
return (
<div>
<LoadCanvasTemplate />
<input
type="text"
placeholder="Enter Captcha"
value={userInput}
onChange={(e) => setUserInput(e.target.value)}
/>
<button onClick={handleSubmit}>Submit</button>
</div>
);
};
export default CaptchaComponent;
Advanced Usage
React Simple Captcha offers various customization options to tailor the captcha to your specific needs.
Customizing Appearance
You can customize the appearance of the captcha by passing props to the LoadCanvasTemplate
component:
<LoadCanvasTemplate reloadText="Refresh Captcha" reloadColor="#ff0000" />
This changes the reload button text and color.
Modifying Captcha Characters
You can control the type of characters used in the captcha by passing additional parameters to the loadCaptchaEnginge
function:
// Only uppercase letters and numbers
loadCaptchaEnginge(6, '', '', 'upper');
// Only lowercase letters and numbers
loadCaptchaEnginge(6, '', '', 'lower');
// Only numbers
loadCaptchaEnginge(6, '', '', 'numbers');
// Only special characters
loadCaptchaEnginge(6, '', '', 'special_char');
Changing Background and Font Colors
You can also customize the background and font colors of the captcha:
// Custom background color (red) and font color (white)
loadCaptchaEnginge(6, 'red', 'white');
Conclusion
React Simple Captcha provides a powerful and flexible solution for implementing captchas in React applications. Its ease of use, customization options, and robust validation make it an excellent choice for developers looking to enhance their application’s security.
By following the steps outlined in this article, you can quickly integrate and customize React Simple Captcha to suit your specific needs. Remember to consider the balance between security and user experience when implementing captchas in your applications.
For more insights on enhancing your React applications, check out our articles on Mastering React Icons Library and Elevating UI with React Aria. These resources will help you further improve the visual appeal and accessibility of your React projects.