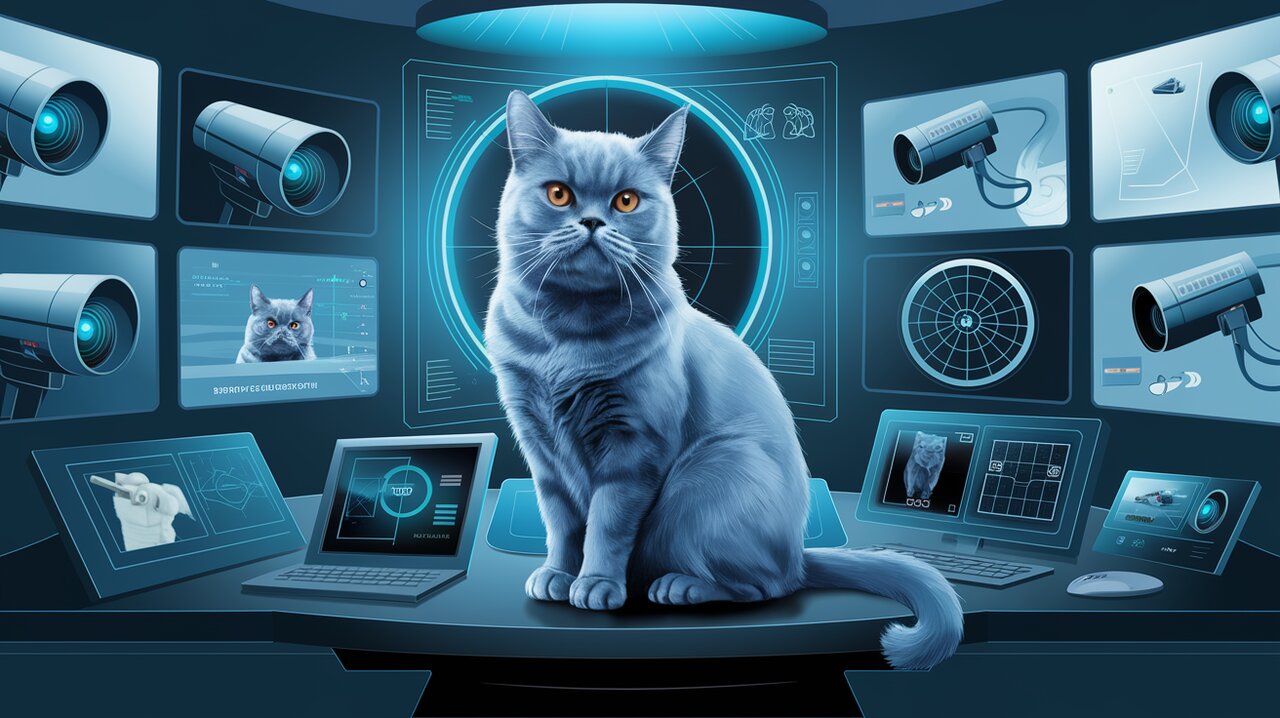
Capturing Reality: Unleash the Power of react-vision-camera in Your React Apps
In today’s web development landscape, the ability to integrate camera functionality into applications has become increasingly important. Whether you’re building a video chat application, implementing barcode scanning, or diving into the realm of augmented reality, having a reliable and flexible camera component is crucial. Enter React Vision Camera, a powerful library that brings advanced camera capabilities to your React applications with ease and efficiency.
Illuminating Features of React Vision Camera
React Vision Camera comes packed with a set of features that make it stand out in the crowded field of camera libraries:
- Seamless integration with React applications
- Support for both front and back cameras
- Customizable resolution settings
- Pause and resume functionality
- Device selection capabilities
- Event-driven architecture for easy control and monitoring
Setting Up Your Camera Rig
Before we dive into the exciting world of camera manipulation, let’s get React Vision Camera installed in your project. You can use either npm or yarn to add this powerful tool to your development arsenal.
Using npm:
npm install react-vision-camera
Or if you prefer yarn:
yarn add react-vision-camera
Lights, Camera, Action: Basic Usage
Now that we have our camera equipment ready, let’s set up a basic scene to capture some footage. Here’s how you can create a simple camera component in your React application:
import React, { useState } from 'react';
import { VisionCamera } from 'react-vision-camera';
const CameraComponent: React.FC = () => {
const [isActive, setIsActive] = useState(true);
const [isPaused, setIsPaused] = useState(false);
const handleCameraOpen = (cam: HTMLVideoElement, camLabel: string) => {
console.log(`Camera opened: ${camLabel}`);
};
return (
<VisionCamera
isActive={isActive}
isPause={isPaused}
desiredCamera="back"
desiredResolution={{ width: 1280, height: 720 }}
onOpened={handleCameraOpen}
/>
);
};
export default CameraComponent;
In this basic setup, we’re creating a camera component that starts active and uses the back camera (if available) with a resolution of 1280x720. The onOpened
callback allows us to perform actions when the camera is successfully initialized.
Controlling the Camera State
The isActive
and isPause
props give you fine-grained control over the camera’s state. You can use these to implement features like turning the camera on/off or pausing the video feed:
const CameraControls: React.FC = () => {
const [isActive, setIsActive] = useState(true);
const [isPaused, setIsPaused] = useState(false);
return (
<div>
<VisionCamera
isActive={isActive}
isPause={isPaused}
// ... other props
/>
<button onClick={() => setIsActive(!isActive)}>
{isActive ? 'Turn Off Camera' : 'Turn On Camera'}
</button>
<button onClick={() => setIsPaused(!isPaused)}>
{isPaused ? 'Resume' : 'Pause'}
</button>
</div>
);
};
This example demonstrates how you can create simple controls to toggle the camera’s active state and pause/resume the video feed.
Advanced Cinematography: Exploring Advanced Features
Now that we’ve covered the basics, let’s delve into some of the more advanced features that React Vision Camera offers. These capabilities allow you to create more sophisticated and interactive camera experiences.
Selecting the Perfect Shot: Camera Device Management
One of the powerful features of React Vision Camera is its ability to work with multiple camera devices. Here’s how you can implement device selection in your application:
import React, { useState } from 'react';
import { VisionCamera } from 'react-vision-camera';
const AdvancedCameraComponent: React.FC = () => {
const [devices, setDevices] = useState<MediaDeviceInfo[]>([]);
const [selectedDevice, setSelectedDevice] = useState<string | null>(null);
const handleDeviceListLoaded = (deviceList: MediaDeviceInfo[]) => {
setDevices(deviceList);
if (deviceList.length > 0) {
setSelectedDevice(deviceList[0].deviceId);
}
};
return (
<div>
<VisionCamera
isActive={true}
desiredCamera={selectedDevice || undefined}
onDeviceListLoaded={handleDeviceListLoaded}
/>
<select
value={selectedDevice || ''}
onChange={(e) => setSelectedDevice(e.target.value)}
>
{devices.map((device) => (
<option key={device.deviceId} value={device.deviceId}>
{device.label}
</option>
))}
</select>
</div>
);
};
export default AdvancedCameraComponent;
This component uses the onDeviceListLoaded
callback to populate a list of available camera devices. Users can then select which camera they want to use through a dropdown menu.
Framing the Perfect Shot: Resolution Control
For applications that require specific video quality, React Vision Camera allows you to set desired resolutions. Here’s how you can implement dynamic resolution control:
import React, { useState } from 'react';
import { VisionCamera } from 'react-vision-camera';
const ResolutionControl: React.FC = () => {
const [resolution, setResolution] = useState({ width: 1280, height: 720 });
const resolutionOptions = [
{ label: '720p', width: 1280, height: 720 },
{ label: '1080p', width: 1920, height: 1080 },
{ label: '4K', width: 3840, height: 2160 },
];
return (
<div>
<VisionCamera
isActive={true}
desiredResolution={resolution}
/>
<select
value={`${resolution.width}x${resolution.height}`}
onChange={(e) => {
const [width, height] = e.target.value.split('x').map(Number);
setResolution({ width, height });
}}
>
{resolutionOptions.map((option) => (
<option key={option.label} value={`${option.width}x${option.height}`}>
{option.label}
</option>
))}
</select>
</div>
);
};
export default ResolutionControl;
This component allows users to switch between different predefined resolutions, demonstrating how you can dynamically adjust the camera’s output quality.
The Final Cut: Wrapping Up
React Vision Camera provides a robust foundation for integrating camera functionality into your React applications. From basic video capture to advanced device and resolution control, this library offers the tools you need to create engaging and interactive camera-based features.
As you continue to explore the capabilities of React Vision Camera, remember that it opens up possibilities for more advanced use cases such as real-time image processing, computer vision tasks, and augmented reality applications. By combining this library with other React ecosystem tools, you can create truly innovative and immersive user experiences.
Whether you’re building a simple photo booth app or a complex computer vision system, React Vision Camera equips you with the necessary tools to bring your ideas to life. So grab your virtual camera, start experimenting, and let your creativity shine through the lens of React development!