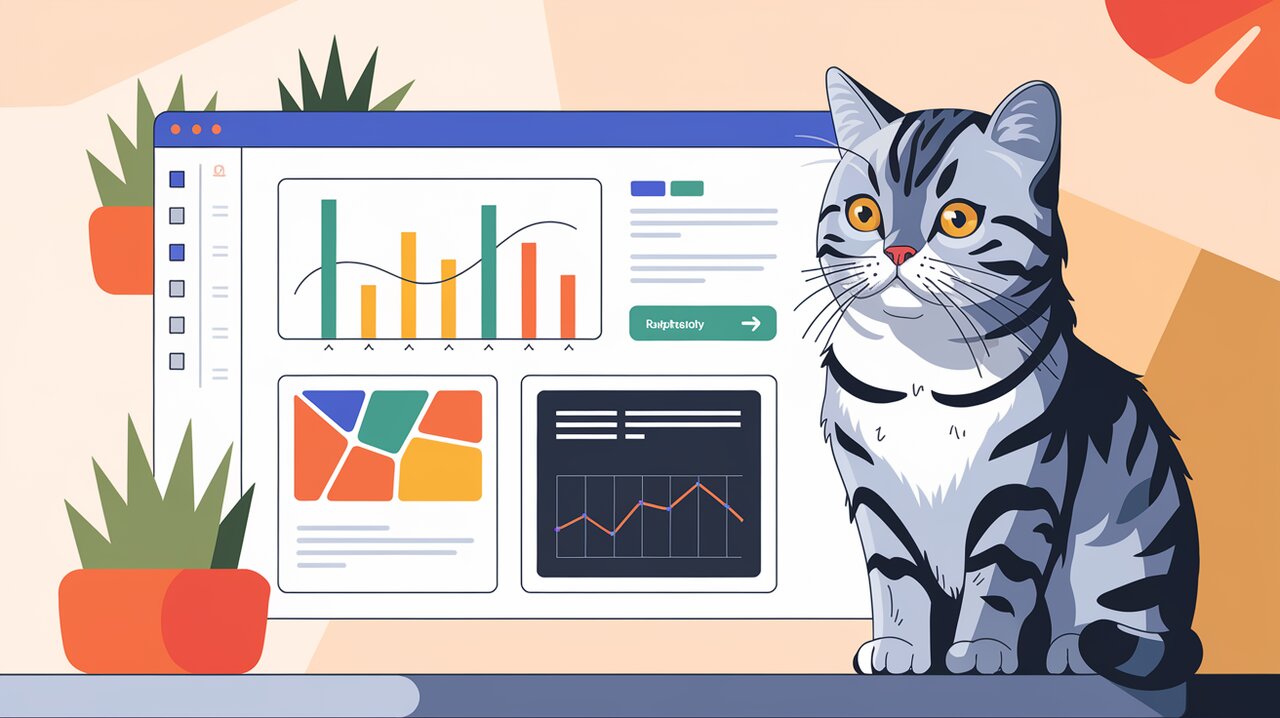
Orchestrating UI Harmony with CDBreact: A React Bootstrap Symphony
CDBreact is a powerful UI kit that brings together the best of React and Bootstrap, offering developers an elegant solution for building responsive and visually appealing web applications. This library provides a comprehensive set of reusable components that adhere to modern design principles, making it easier than ever to create stunning user interfaces with minimal effort.
Features That Sing
CDBreact comes packed with a symphony of features that will delight developers and end-users alike:
- Extensive Component Library: From basic elements like buttons and alerts to complex widgets like carousels and data tables, CDBreact offers a wide array of components to suit various UI needs.
- Responsive Design: Built on Bootstrap’s grid system, CDBreact ensures your applications look great on devices of all sizes.
- Customizable Themes: Easily adapt the look and feel of components to match your brand’s aesthetic.
- Accessibility: Components are designed with accessibility in mind, ensuring your applications are usable by everyone.
- TypeScript Support: Enjoy the benefits of type checking and enhanced IDE support with TypeScript definitions.
Installation: Setting the Stage
Getting started with CDBreact is as simple as adding an instrument to your orchestra. You can install the library using npm or yarn:
npm install --save cdbreact
Or if you prefer yarn:
yarn add cdbreact
Basic Usage: Your First Composition
Once installed, you can start using CDBreact components in your React application. Here’s a simple example to get you started:
import React from 'react';
import { CDBAlert } from 'cdbreact';
const MyComponent = () => {
return (
<CDBAlert color="primary">
Welcome to the world of CDBreact!
</CDBAlert>
);
};
export default MyComponent;
This code snippet creates a primary-colored alert component, showcasing the simplicity and elegance of CDBreact’s API.
Advanced Harmonies: Exploring Complex Components
CDBreact truly shines when you start combining its components to create more complex UI elements. Let’s look at a more advanced example using a card component with some interactive elements:
import React from 'react';
import { CDBCard, CDBCardBody, CDBCardTitle, CDBCardText, CDBBtn } from 'cdbreact';
const AdvancedCard = () => {
return (
<CDBCard style={{ width: '18rem' }}>
<CDBCardBody>
<CDBCardTitle>CDBreact Magic</CDBCardTitle>
<CDBCardText>
Discover the power of CDBreact with this interactive card component.
</CDBCardText>
<CDBBtn color="primary">Learn More</CDBBtn>
</CDBCardBody>
</CDBCard>
);
};
export default AdvancedCard;
This example demonstrates how to create a card with a title, text, and a button, all using CDBreact components. The result is a cohesive and visually appealing UI element that’s both responsive and interactive.
Responsive Design: Adapting to Every Stage
One of CDBreact’s strongest suits is its built-in responsiveness. Let’s create a responsive grid layout to showcase this feature:
import React from 'react';
import { CDBContainer, CDBRow, CDBCol } from 'cdbreact';
const ResponsiveGrid = () => {
return (
<CDBContainer>
<CDBRow>
<CDBCol sm="4">
<h3>Column 1</h3>
<p>This column takes up 4 units on small screens and above.</p>
</CDBCol>
<CDBCol sm="4">
<h3>Column 2</h3>
<p>This column also takes up 4 units on small screens and above.</p>
</CDBCol>
<CDBCol sm="4">
<h3>Column 3</h3>
<p>And this one completes the row with 4 units.</p>
</CDBCol>
</CDBRow>
</CDBContainer>
);
};
export default ResponsiveGrid;
This grid will automatically adjust its layout based on the screen size, ensuring your content looks great on everything from mobile phones to large desktop monitors.
Customization: Tuning Your Instruments
While CDBreact components look great out of the box, you might want to customize them to match your project’s specific needs. Most components accept custom props for styling and behavior modification. Here’s an example of customizing a button:
import React from 'react';
import { CDBBtn } from 'cdbreact';
const CustomButton = () => {
return (
<CDBBtn
color="info"
size="large"
style={{ borderRadius: '25px' }}
onClick={() => console.log('Button clicked!')}
>
Custom Action
</CDBBtn>
);
};
export default CustomButton;
This button has a custom color, size, border-radius, and click handler, demonstrating the flexibility of CDBreact components.
Conclusion: The Final Crescendo
CDBreact offers a harmonious blend of React’s component-based architecture and Bootstrap’s responsive design principles. With its extensive library of components, ease of use, and customization options, it’s an excellent choice for developers looking to create beautiful and functional user interfaces quickly and efficiently.
Whether you’re building a simple landing page or a complex web application, CDBreact provides the tools you need to orchestrate a stunning UI symphony. As you continue to explore this library, you’ll discover even more ways to enhance your React projects and delight your users with smooth, responsive, and visually appealing interfaces.
For more insights into React libraries and UI components, check out our articles on Bootstrapping Your React App with React Bootstrap and Chakra UI React Symphony. Happy coding, and may your CDBreact compositions always hit the right notes!