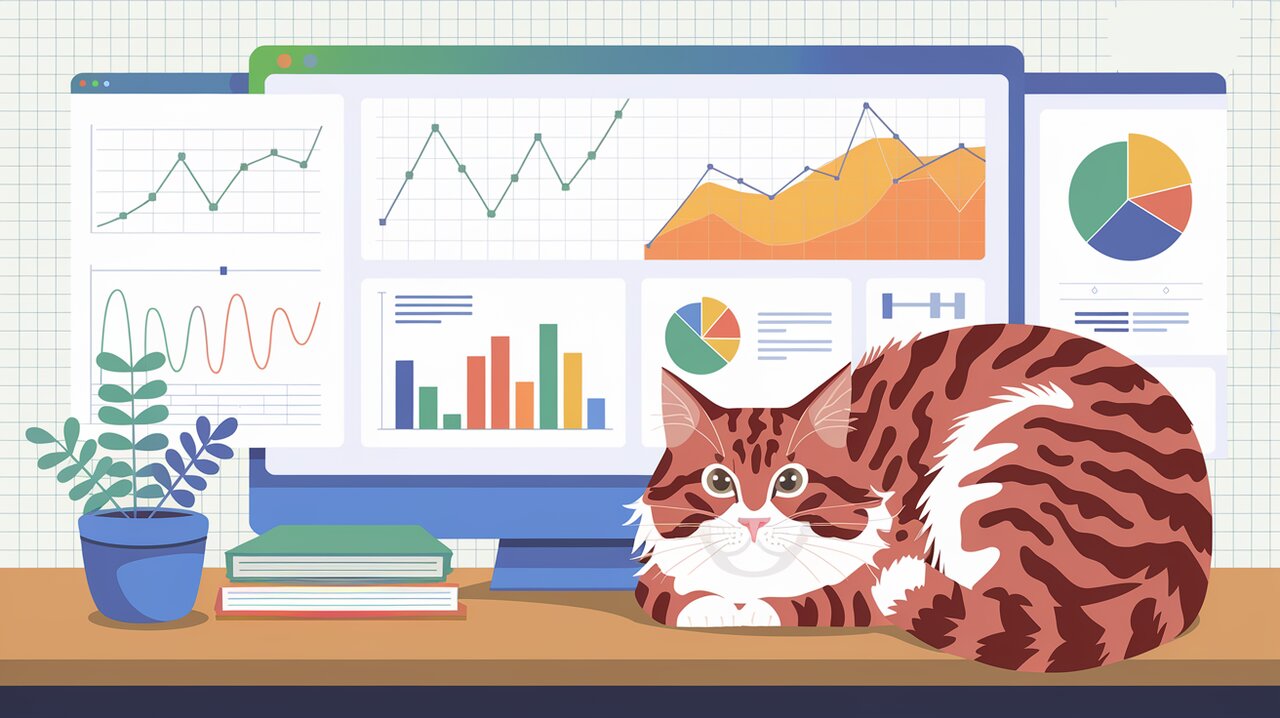
Chart Your Course with Recharts: Navigating Data Visualization in React
Recharts is a powerful and flexible charting library for React that allows developers to create beautiful, responsive charts with ease. Built on top of SVG and leveraging the power of D3, Recharts provides a declarative approach to chart creation, making it simple to integrate data visualization into your React applications.
Why Choose Recharts?
Recharts stands out from other charting libraries for several reasons:
- React-centric: Designed specifically for React applications, Recharts seamlessly integrates with your existing React components.
- Declarative API: Create complex charts using simple, declarative components that are easy to understand and maintain.
- Customizable: Offers extensive customization options to tailor charts to your specific needs.
- Responsive: Charts automatically adjust to different screen sizes, ensuring a great user experience across devices.
- Performance: Utilizes SVG for rendering, providing smooth animations and interactions.
Getting Started with Recharts
Let’s dive into how you can start using Recharts in your React project.
Installation
You can install Recharts using npm or yarn:
npm install recharts
# or
yarn add recharts
Basic Usage
Here’s a simple example of how to create a line chart using Recharts:
import React from 'react';
import { LineChart, Line, XAxis, YAxis, CartesianGrid, Tooltip, Legend } from 'recharts';
const data = [
{ name: 'Page A', uv: 4000, pv: 2400, amt: 2400 },
{ name: 'Page B', uv: 3000, pv: 1398, amt: 2210 },
{ name: 'Page C', uv: 2000, pv: 9800, amt: 2290 },
{ name: 'Page D', uv: 2780, pv: 3908, amt: 2000 },
{ name: 'Page E', uv: 1890, pv: 4800, amt: 2181 },
{ name: 'Page F', uv: 2390, pv: 3800, amt: 2500 },
{ name: 'Page G', uv: 3490, pv: 4300, amt: 2100 },
];
const SimpleLineChart = () => {
return (
<LineChart width={600} height={300} data={data} margin={{ top: 5, right: 30, left: 20, bottom: 5 }}>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="name" />
<YAxis />
<Tooltip />
<Legend />
<Line type="monotone" dataKey="pv" stroke="#8884d8" />
<Line type="monotone" dataKey="uv" stroke="#82ca9d" />
</LineChart>
);
};
export default SimpleLineChart;
This code creates a simple line chart with two data series, complete with axes, grid lines, tooltips, and a legend.
Advanced Features
Recharts offers a wide range of chart types and customization options. Let’s explore some advanced features.
Customizing Chart Appearance
You can easily customize the appearance of your charts by adjusting properties of the chart components:
<LineChart width={600} height={300} data={data}>
<Line type="monotone" dataKey="pv" stroke="#8884d8" strokeWidth={2} dot={{ r: 8 }} />
<Line type="monotone" dataKey="uv" stroke="#82ca9d" strokeWidth={2} dot={{ r: 8 }} />
</LineChart>
This code increases the line thickness and dot size for better visibility.
Adding Interactivity
Recharts supports interactive features like zooming and cursor tracking:
import { LineChart, Line, XAxis, YAxis, CartesianGrid, Tooltip, Legend, ReferenceArea } from 'recharts';
const InteractiveChart = () => {
const [left, setLeft] = useState('dataMin');
const [right, setRight] = useState('dataMax');
const [refAreaLeft, setRefAreaLeft] = useState('');
const [refAreaRight, setRefAreaRight] = useState('');
const zoom = () => {
if (refAreaLeft === refAreaRight || refAreaRight === '') {
setRefAreaLeft('');
setRefAreaRight('');
return;
}
if (refAreaLeft > refAreaRight) {
[refAreaLeft, refAreaRight] = [refAreaRight, refAreaLeft];
}
setLeft(refAreaLeft);
setRight(refAreaRight);
setRefAreaLeft('');
setRefAreaRight('');
};
return (
<LineChart
width={600}
height={300}
data={data}
onMouseDown={(e) => setRefAreaLeft(e.activeLabel)}
onMouseMove={(e) => refAreaLeft && setRefAreaRight(e.activeLabel)}
onMouseUp={zoom}
>
{/* Chart components */}
{refAreaLeft && refAreaRight ? (
<ReferenceArea x1={refAreaLeft} x2={refAreaRight} strokeOpacity={0.3} />
) : null}
</LineChart>
);
};
This example demonstrates how to implement a zoomable chart with Recharts.
Best Practices
When working with Recharts, keep these best practices in mind:
- Responsiveness: Use percentage values for width and height to ensure charts are responsive.
- Performance: For large datasets, consider using the
ResponsiveContainer
component to optimize rendering. - Accessibility: Provide meaningful labels and use contrasting colors to make your charts accessible to all users.
- Data Formatting: Preprocess your data to match Recharts’ expected format for optimal performance.
Conclusion
Recharts offers a powerful and flexible solution for creating beautiful, interactive charts in React applications. Its declarative API and extensive customization options make it an excellent choice for developers looking to add data visualization to their projects.
By mastering Recharts, you can create stunning visualizations that bring your data to life, enhancing user engagement and understanding. Whether you’re building a complex dashboard or simply need to display some basic metrics, Recharts provides the tools you need to chart your course through the sea of data.
For more insights on React libraries and data visualization, check out our articles on Chart.js Symphony with react-chartjs-2 and Visualizing Data: react-vis Symphony. These complementary libraries can further expand your data visualization toolkit in React.