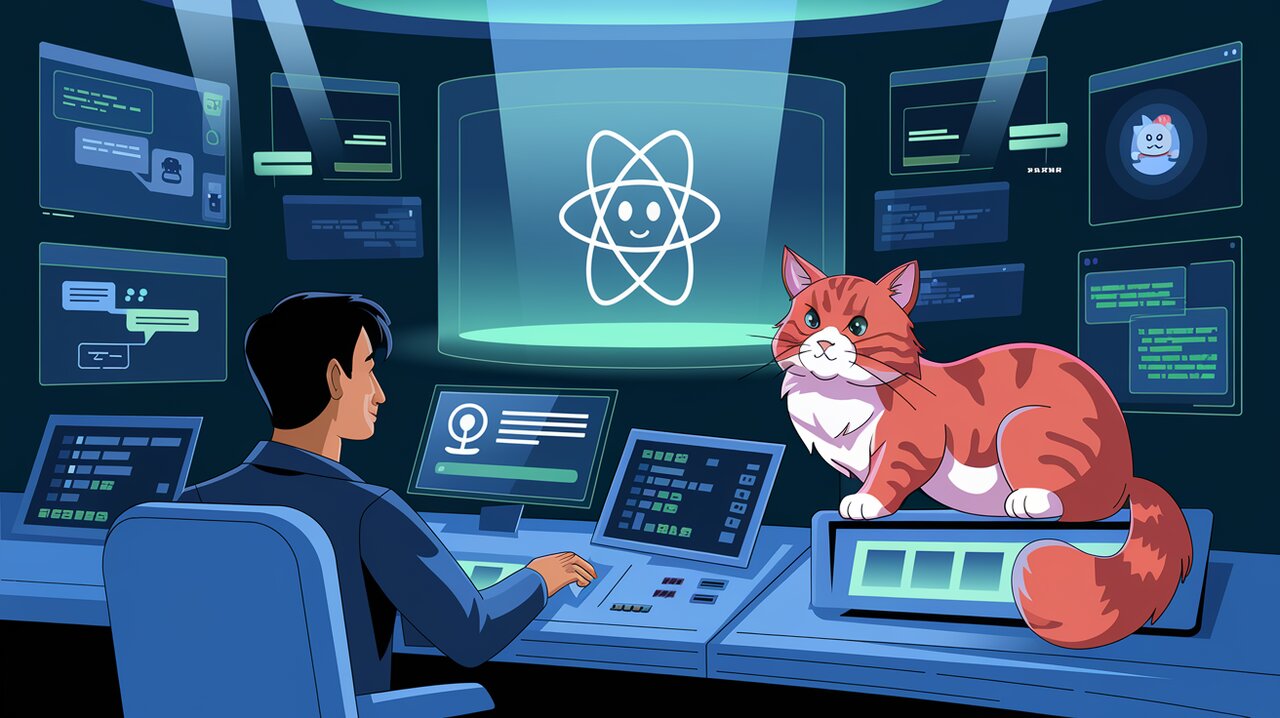
Chatbot Chatter: Unleashing the Power of react-chatbot-kit
React-chatbot-kit is a powerful library that enables developers to create interactive and customizable chatbots for React applications. With its intuitive API and flexible architecture, you can quickly build conversational interfaces that engage users and enhance their experience. Whether you’re looking to create a simple FAQ bot or a complex AI-driven assistant, react-chatbot-kit provides the tools you need to bring your chatbot vision to life.
Chatbot Capabilities
React-chatbot-kit comes packed with features that make it a versatile choice for chatbot development:
- Easy setup and configuration
- Customizable UI components
- Widget support for rich interactions
- Flexible message parsing and action handling
- State management for complex conversations
- Support for custom styling
Setting Up Your Chatbot
To get started with react-chatbot-kit, you’ll need to install the package in your React project. Open your terminal and run one of the following commands:
npm install react-chatbot-kit
# or
yarn add react-chatbot-kit
Once installed, you can import the necessary components and styles in your React application:
import Chatbot from 'react-chatbot-kit';
import 'react-chatbot-kit/build/main.css';
Crafting Your First Chatbot
To create a functional chatbot, you’ll need to set up three key components: the configuration, the message parser, and the action provider.
Configuring Your Bot
Start by creating a config.ts
file to define your chatbot’s initial settings:
import { createChatBotMessage } from 'react-chatbot-kit';
const config = {
initialMessages: [createChatBotMessage('Hello! How can I assist you today?')],
botName: 'FriendlyBot',
};
export default config;
This configuration sets up the initial greeting message and gives your bot a name.
Parsing User Messages
Next, create a MessageParser.tsx
file to handle incoming user messages:
import React from 'react';
const MessageParser: React.FC<any> = ({ children, actions }) => {
const parse = (message: string) => {
if (message.toLowerCase().includes('hello')) {
actions.handleHello();
}
};
return (
<div>
{React.Children.map(children, (child) => {
return React.cloneElement(child, {
parse: parse,
actions: {},
});
})}
</div>
);
};
export default MessageParser;
This parser checks for the word “hello” in user messages and triggers a corresponding action.
Providing Bot Actions
Create an ActionProvider.tsx
file to define how your bot responds to user inputs:
import React from 'react';
const ActionProvider: React.FC<any> = ({ createChatBotMessage, setState, children }) => {
const handleHello = () => {
const botMessage = createChatBotMessage('Hello! Nice to meet you.');
setState((prev: any) => ({
...prev,
messages: [...prev.messages, botMessage],
}));
};
return (
<div>
{React.Children.map(children, (child) => {
return React.cloneElement(child, {
actions: {
handleHello,
},
});
})}
</div>
);
};
export default ActionProvider;
This action provider defines a handleHello
function that creates a bot response when triggered.
Bringing It All Together
Now, you can use these components to render your chatbot in a React component:
import React from 'react';
import Chatbot from 'react-chatbot-kit';
import 'react-chatbot-kit/build/main.css';
import config from './config';
import MessageParser from './MessageParser';
import ActionProvider from './ActionProvider';
const MyChatbot: React.FC = () => {
return (
<div>
<Chatbot
config={config}
messageParser={MessageParser}
actionProvider={ActionProvider}
/>
</div>
);
};
export default MyChatbot;
Enhancing Your Chatbot
With the basic setup complete, let’s explore some advanced features of react-chatbot-kit.
Custom Widgets
Widgets allow you to create rich, interactive elements within your chatbot conversations. Here’s how you can create and use a custom widget:
import React from 'react';
const WeatherWidget: React.FC = () => {
return (
<div>
<h3>Today's Weather</h3>
<p>Sunny, 72°F</p>
</div>
);
};
export default WeatherWidget;
To use this widget, update your config.ts
:
import WeatherWidget from './WeatherWidget';
const config = {
// ... other config options
widgets: [
{
widgetName: 'weatherWidget',
widgetFunc: (props) => <WeatherWidget {...props} />,
},
],
};
Now you can trigger this widget in your ActionProvider
:
const showWeather = () => {
const botMessage = createChatBotMessage(
"Here's today's weather forecast:",
{
widget: 'weatherWidget',
}
);
setState((prev: any) => ({
...prev,
messages: [...prev.messages, botMessage],
}));
};
Styling Your Chatbot
React-chatbot-kit allows for easy customization of your chatbot’s appearance. Add custom styles to your config.ts
:
const config = {
// ... other config options
customStyles: {
botMessageBox: {
backgroundColor: '#376B7E',
},
chatButton: {
backgroundColor: '#5ccc9d',
},
},
};
This will change the color of the bot’s message boxes and the chat button.
Handling Complex Conversations
For more complex interactions, you can use state management within your ActionProvider
:
const ActionProvider: React.FC<any> = ({ createChatBotMessage, setState, children }) => {
const [userPreferences, setUserPreferences] = React.useState({});
const handlePreferences = (preference: string) => {
setUserPreferences((prev) => ({ ...prev, [preference]: true }));
const botMessage = createChatBotMessage(`I've noted your preference for ${preference}.`);
setState((prev: any) => ({
...prev,
messages: [...prev.messages, botMessage],
}));
};
// ... other action handlers
return (
<div>
{React.Children.map(children, (child) => {
return React.cloneElement(child, {
actions: {
handlePreferences,
// ... other actions
},
});
})}
</div>
);
};
This example demonstrates how to maintain user preferences across the conversation.
Wrapping Up
React-chatbot-kit provides a robust foundation for building interactive chatbots in React applications. By leveraging its configuration options, message parsing capabilities, and action providers, you can create chatbots that range from simple to highly complex. The ability to incorporate custom widgets and styling ensures that your chatbot can be tailored to fit seamlessly into any application design.
As you continue to explore react-chatbot-kit, you’ll discover even more ways to enhance your chatbot’s functionality. From integrating with backend services to implementing natural language processing, the possibilities are vast. Remember to consult the official documentation for the most up-to-date information and advanced usage scenarios.
With the knowledge gained from this guide, you’re well-equipped to start building engaging chatbot experiences that will delight your users and add a new dimension of interactivity to your React applications. Happy chatbot building!