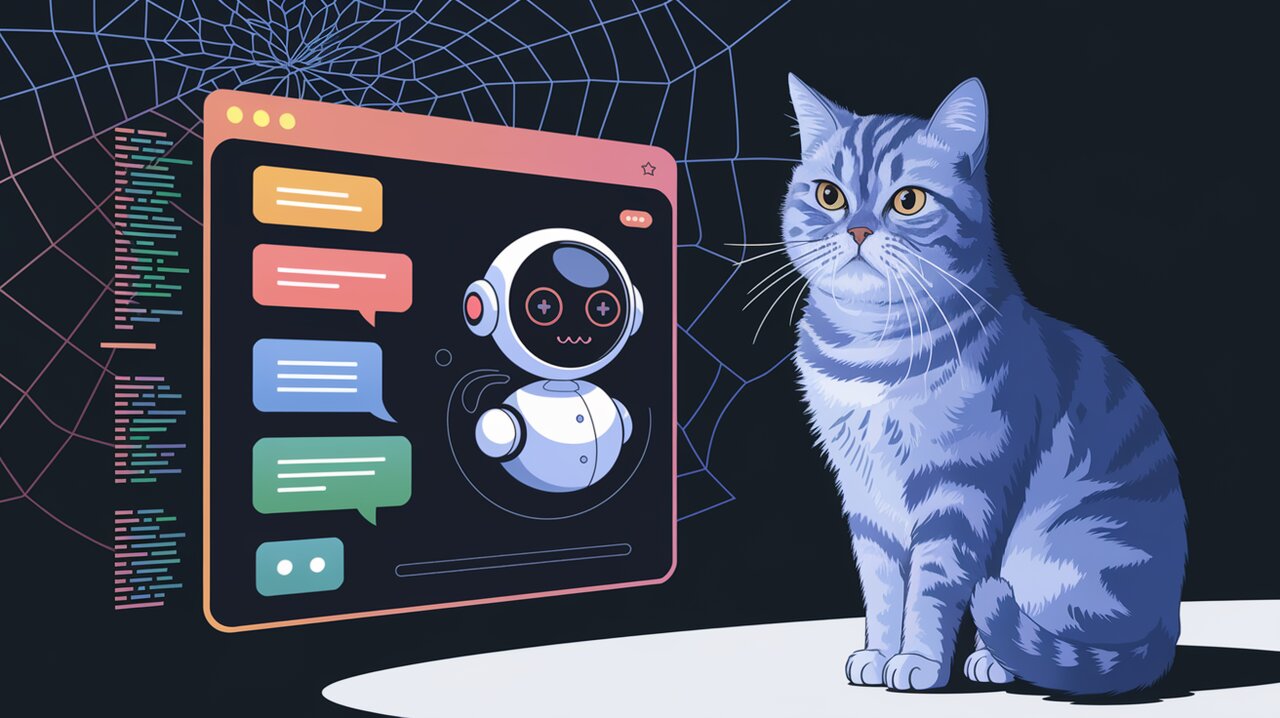
Chatbot Chatter: Unleashing the Power of React Simple Chatbot
React Simple Chatbot is a powerful library that enables developers to create interactive and customizable chatbot interfaces with ease. This component-based solution offers a straightforward approach to building conversation chats, making it an excellent choice for enhancing user engagement in React applications.
Features of React Simple Chatbot
React Simple Chatbot comes packed with a variety of features that make it stand out:
- Easy integration with React applications
- Customizable chat flow and user interactions
- Support for various types of user inputs
- Ability to include custom components within chat messages
- Theming options for personalizing the chatbot’s appearance
Installation
Getting started with React Simple Chatbot is a breeze. You can install it using npm or yarn:
npm install react-simple-chatbot --save
Or if you prefer yarn:
yarn add react-simple-chatbot
Basic Usage
Let’s dive into a simple example to demonstrate how to use React Simple Chatbot:
import React from 'react';
import ChatBot from 'react-simple-chatbot';
const steps = [
{
id: '0',
message: 'Welcome to React Simple Chatbot!',
trigger: '1',
},
{
id: '1',
message: 'Please type your name',
trigger: 'name',
},
{
id: 'name',
user: true,
trigger: '3',
},
{
id: '3',
message: 'Hi {previousValue}! How can I help you today?',
end: true,
},
];
const SimpleChatbot = () => (
<ChatBot steps={steps} />
);
export default SimpleChatbot;
In this example, we define a series of steps that guide the conversation flow. The chatbot welcomes the user, asks for their name, and then greets them personally. The trigger
property determines the next step in the conversation, while the user: true
property indicates that the chatbot is expecting user input.
Advanced Usage: Custom Components
React Simple Chatbot allows you to incorporate custom components into your chat flow, enabling more complex interactions:
import React from 'react';
import ChatBot from 'react-simple-chatbot';
const CustomComponent = ({ steps }) => {
return (
<div>
<h1>Custom Component</h1>
<p>You can add any React component or HTML here.</p>
</div>
);
};
const steps = [
{
id: '1',
message: 'Here\'s a custom component:',
trigger: '2',
},
{
id: '2',
component: <CustomComponent />,
trigger: '3',
},
{
id: '3',
message: 'What do you think about it?',
end: true,
},
];
const AdvancedChatbot = () => (
<ChatBot steps={steps} />
);
export default AdvancedChatbot;
This example demonstrates how to include a custom React component within the chat flow, allowing for more dynamic and interactive conversations.
Styling and Theming
React Simple Chatbot offers theming options to customize the appearance of your chatbot. You can adjust colors, fonts, and other visual elements to match your application’s design:
import React from 'react';
import ChatBot from 'react-simple-chatbot';
import { ThemeProvider } from 'styled-components';
const theme = {
background: '#f5f8fb',
fontFamily: 'Helvetica Neue',
headerBgColor: '#EF6C00',
headerFontColor: '#fff',
headerFontSize: '15px',
botBubbleColor: '#EF6C00',
botFontColor: '#fff',
userBubbleColor: '#fff',
userFontColor: '#4a4a4a',
};
const ThemedChatbot = () => (
<ThemeProvider theme={theme}>
<ChatBot
steps={[
{
id: '1',
message: 'Hello! This chatbot has a custom theme.',
end: true,
},
]}
/>
</ThemeProvider>
);
export default ThemedChatbot;
By wrapping your ChatBot component with a ThemeProvider, you can easily apply custom styles to create a unique look for your chatbot.
Conclusion
React Simple Chatbot provides an intuitive and flexible solution for integrating conversational interfaces into React applications. Its ease of use, customization options, and support for complex interactions make it a valuable tool for developers looking to enhance user engagement through chatbots.
While exploring React Simple Chatbot, you might also be interested in other React libraries that can complement your chatbot development. For instance, the React Toastify library can be used to display notifications alongside your chatbot, enhancing the overall user experience. Additionally, for more advanced state management in complex chatbot applications, you might want to check out the Redux Saga library, which can help manage side effects and asynchronous flows in your React and Redux applications.
By leveraging React Simple Chatbot and these complementary libraries, you can create sophisticated, interactive, and user-friendly chatbot experiences that will set your application apart.