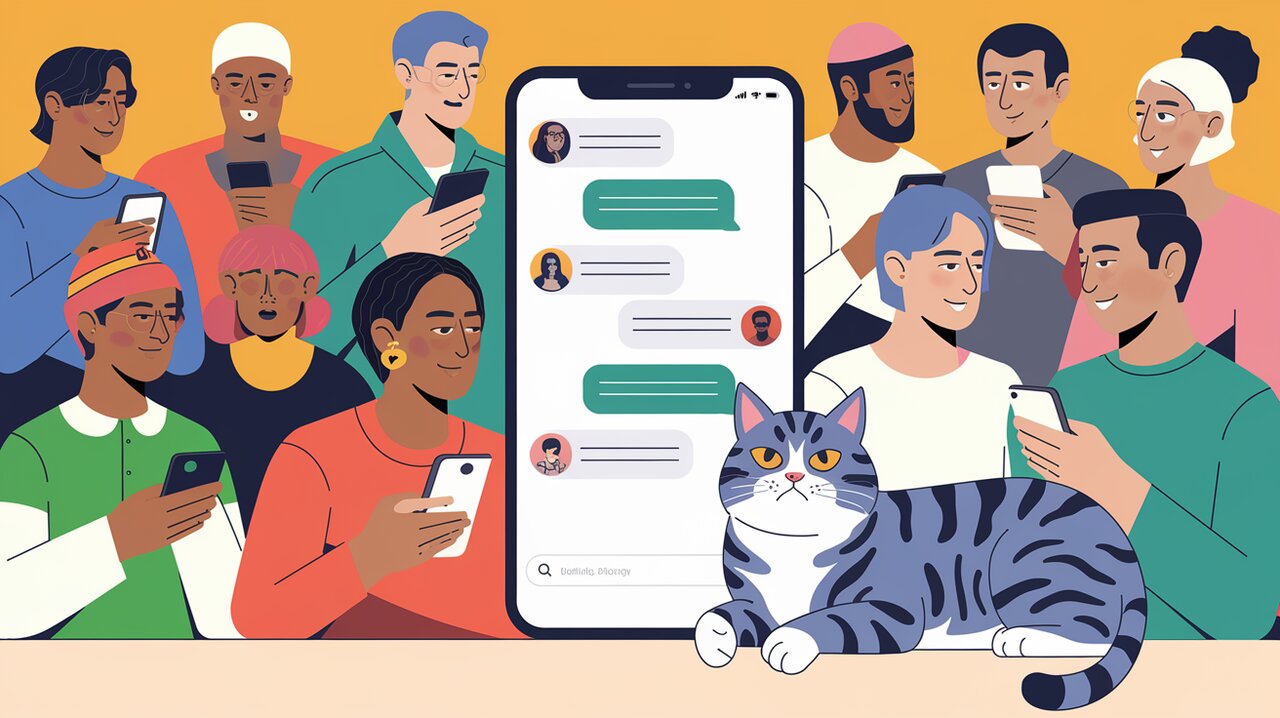
The Stream Chat library for React is an official SDK designed to help developers create robust chat applications effortlessly. It provides a comprehensive set of components that allow for seamless integration of messaging capabilities into any React application. Whether you’re building a customer support platform, an in-game chat feature, or a social messaging app, this library has you covered.
With Stream Chat, you can create various use cases including:
- Livestream chats similar to those on Twitch or YouTube.
- In-game communication tools like those found in Overwatch or Fortnite.
- Team collaboration spaces akin to Slack.
- Messaging applications reminiscent of WhatsApp or Facebook Messenger.
- Customer support interfaces similar to Drift or Intercom.
Key Features
-
Real-time Messaging: Enjoy real-time communication with instant message delivery and updates.
-
Customizable UI Components: Tailor the appearance and behavior of chat components to match your application’s design.
Getting Started
To begin using stream-chat-react
, you’ll need to install it along with its dependencies. Here’s how you can do that:
Installation via NPM
To install the necessary packages using npm, run:
npm install react react-dom stream-chat stream-chat-react
Installation via Yarn
If you prefer using Yarn, execute:
yarn add react react-dom stream-chat stream-chat-react
CDN Installation
For quick setups without package managers, you can include the library via CDN:
<script src="https://cdn.jsdelivr.net/npm/react@16.13.1/umd/react.production.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/react-dom@16/umd/react-dom.production.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/stream-chat"></script>
<script src="https://cdn.jsdelivr.net/npm/stream-chat-react"></script>
Basic Implementation
Setting Up Your Chat Client
To start using stream-chat-react
, you first need to create a client instance and set up the provider. Here’s how you can do that:
import React from 'react';
import { Chat } from 'stream-chat-react';
import { StreamChat } from 'stream-chat';
const client = StreamChat.getInstance('YOUR_API_KEY');
const App = () => {
return (
<Chat client={client} theme="messaging light">
{/* Your chat components will go here */}
</Chat>
);
};
export default App;
In this code snippet, replace 'YOUR_API_KEY'
with your actual API key from Stream. This sets up the basic structure for your chat application.
Creating Channels and Sending Messages
Once your client is set up, you can create channels and send messages. Here’s an example of how to create a channel and send a message:
const channel = client.channel('messaging', 'general', {
name: 'General Chat',
});
await channel.create();
await channel.sendMessage({
text: 'Hello World!',
});
This code creates a new channel called ‘General Chat’ and sends an initial message.
Advanced Customizations
Customizing Message Components
You can customize how messages are displayed by creating your own message component. Here’s an example:
import { MessageSimple } from 'stream-chat-react';
const CustomMessage = (props) => {
return (
<div style={{ padding: '10px', backgroundColor: '#f0f0f0' }}>
<MessageSimple {...props} />
</div>
);
};
// Usage within Channel component
<Channel Message={CustomMessage}>
{/* Other components */}
</Channel>
This customization allows you to style messages according to your application’s design requirements.
Handling User Presence
The library also allows you to manage user presence easily. You can display online/offline status with minimal effort:
<Channel>
<Window>
<ChannelHeader />
<MessageList />
<MessageInput />
</Window>
<Thread />
</Channel>
By incorporating presence indicators, users can see who is online, enhancing engagement within your application.
Wrapping Up
The Stream Chat library for React offers powerful tools and features that simplify the process of adding real-time messaging capabilities to your applications. With its customizable components and straightforward API, developers at any level can create engaging chat experiences tailored to their needs.
For more insights into enhancing your applications with React libraries, check out similar articles such as Elevate Your Chat with Assistant UI or Mastering React Modals with React Modal.