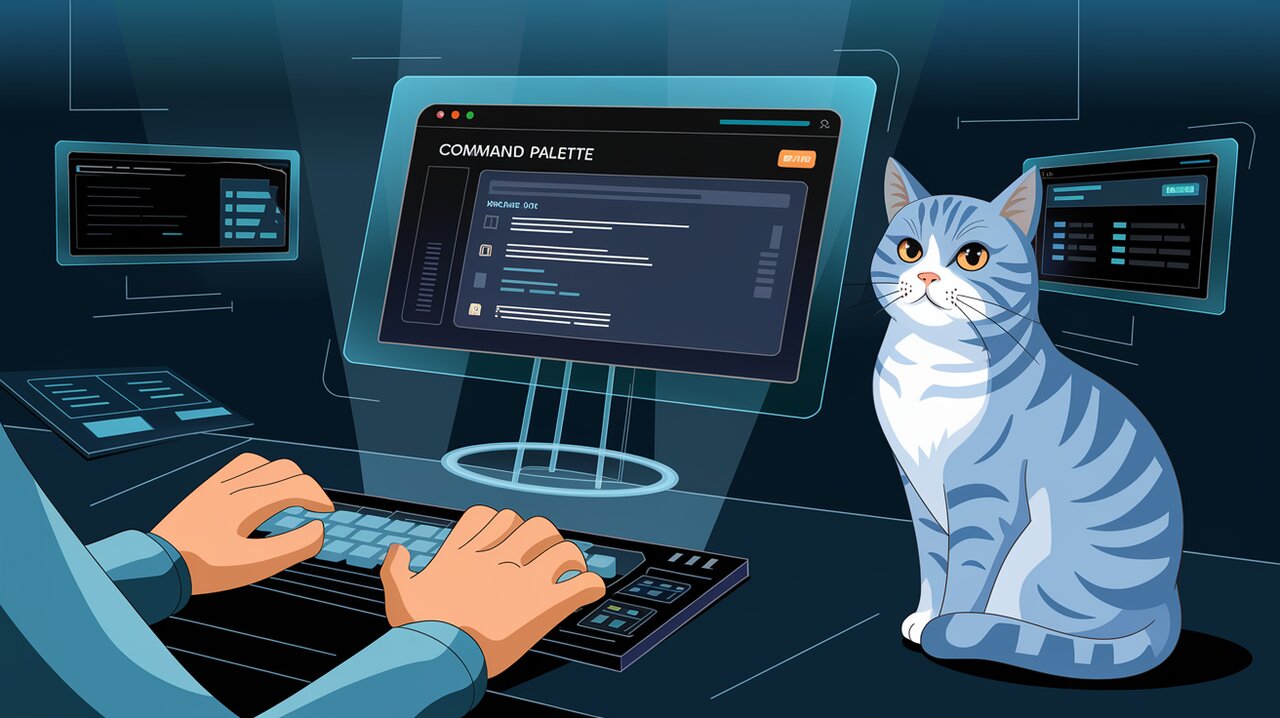
Command-K Mastery: Unleashing the Power of CMDK in React
In the ever-evolving landscape of web development, efficiency and user experience reign supreme. Enter CMDK, a React library that’s revolutionizing the way we think about command palettes. If you’ve ever marveled at the seamless navigation in tools like Slack or Notion, you’ve experienced the power of a well-designed command palette. Now, it’s time to bring that magic to your own React applications.
Unveiling CMDK: Your Command Palette Companion
CMDK is more than just a library; it’s a toolkit for creating lightning-fast, accessible command menus in React. At its core, CMDK offers a composable API that allows developers to build intuitive interfaces that users can navigate with ease. Whether you’re looking to enhance productivity, streamline navigation, or simply add a touch of sophistication to your app, CMDK has got you covered.
Key Features That Set CMDK Apart
- Blazing Fast Performance: CMDK is optimized for speed, ensuring that even with thousands of items, your command palette remains snappy and responsive.
- Accessibility First: Built with a focus on accessibility, CMDK ensures that your command palette is usable by everyone, regardless of their abilities.
- Flexible and Customizable: From simple lists to nested pages, CMDK’s composable API allows you to create the perfect command palette for your needs.
- Unstyled Components: CMDK provides unstyled components, giving you complete control over the look and feel of your command palette.
- React 18 Compatible: Leveraging the latest React features, CMDK is built for the future of web development.
Getting Started with CMDK
Installation: Your First Step to Command Palette Mastery
To begin your journey with CMDK, you’ll need to have Node.js installed on your system. Once you’re set up, installing CMDK is as simple as running a single command in your project directory:
npm install cmdk
Or if you prefer using Yarn:
yarn add cmdk
Basic Usage: Crafting Your First Command Palette
Let’s dive into creating a basic command palette using CMDK. Here’s a simple example to get you started:
import { Command } from 'cmdk'
const CommandMenu = () => {
return (
<Command label="Command Menu">
<Command.Input />
<Command.List>
<Command.Empty>No results found.</Command.Empty>
<Command.Group heading="Actions">
<Command.Item>Create New File</Command.Item>
<Command.Item>Open Project</Command.Item>
<Command.Separator />
<Command.Item>Settings</Command.Item>
</Command.Group>
</Command.List>
</Command>
)
}
This code snippet creates a basic command palette with a search input, a list of items grouped under “Actions”, and a message for when no results are found. The Command.Separator
adds a visual break between related items, enhancing the palette’s readability.
Advanced Techniques: Elevating Your Command Palette
Nested Navigation: Creating a Multi-Page Experience
One of CMDK’s powerful features is the ability to create nested navigation within your command palette. This is particularly useful for organizing complex sets of actions or information. Here’s how you can implement a multi-page command palette:
const [pages, setPages] = useState([])
const page = pages[pages.length - 1]
return (
<Command>
<Command.Input />
<Command.List>
{!page && (
<>
<Command.Item onSelect={() => setPages([...pages, 'projects'])}>
Browse Projects
</Command.Item>
<Command.Item onSelect={() => setPages([...pages, 'teams'])}>
Manage Teams
</Command.Item>
</>
)}
{page === 'projects' && (
<>
<Command.Item>Project Alpha</Command.Item>
<Command.Item>Project Beta</Command.Item>
</>
)}
{page === 'teams' && (
<>
<Command.Item>Engineering Team</Command.Item>
<Command.Item>Design Team</Command.Item>
</>
)}
</Command.List>
</Command>
)
This setup allows users to navigate through different “pages” within the command palette, providing a hierarchical structure to your actions or data.
Asynchronous Data Loading: Keeping Things Smooth
In real-world applications, you often need to load data asynchronously. CMDK makes it easy to handle this scenario gracefully:
const [loading, setLoading] = useState(false)
const [items, setItems] = useState([])
useEffect(() => {
const fetchItems = async () => {
setLoading(true)
const data = await api.fetchItems()
setItems(data)
setLoading(false)
}
fetchItems()
}, [])
return (
<Command>
<Command.Input />
<Command.List>
{loading && <Command.Loading>Fetching items...</Command.Loading>}
{items.map((item) => (
<Command.Item key={item.id}>{item.name}</Command.Item>
))}
</Command.List>
</Command>
)
This approach ensures that users see a loading indicator while data is being fetched, providing a smooth and responsive experience.
Customization: Making CMDK Your Own
While CMDK provides a solid foundation, the real magic happens when you customize it to fit your application’s unique needs. Here are some ways to tailor CMDK to your preferences:
Styling: Crafting the Perfect Look
CMDK components come unstyled, giving you complete control over their appearance. You can use CSS-in-JS solutions, CSS modules, or traditional stylesheets to define your palette’s look. Here’s a simple example using CSS:
[cmdk-root] {
max-width: 640px;
margin: 0 auto;
background: #ffffff;
border-radius: 8px;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
}
[cmdk-input] {
width: 100%;
padding: 12px 16px;
font-size: 16px;
border: none;
border-bottom: 1px solid #e2e8f0;
}
[cmdk-item] {
padding: 8px 16px;
cursor: pointer;
}
[cmdk-item][data-selected="true"] {
background: #e2e8f0;
}
Custom Filtering: Tailoring Search Results
CMDK allows you to define custom filtering logic to better match your application’s needs:
<Command
filter={(value, search) => {
if (value.toLowerCase().includes(search.toLowerCase())) return 1
return 0
}}
>
{/* Command palette content */}
</Command>
This custom filter function performs a case-insensitive search, but you could extend it to include fuzzy matching or other advanced search techniques.
Integrating CMDK with Your React Ecosystem
CMDK plays well with other React libraries and patterns. Here are a couple of integration scenarios to consider:
Using CMDK with React Router
If your application uses React Router, you can integrate CMDK to navigate between routes:
import { useNavigate } from 'react-router-dom'
const CommandPalette = () => {
const navigate = useNavigate()
return (
<Command>
<Command.Input />
<Command.List>
<Command.Item onSelect={() => navigate('/dashboard')}>
Go to Dashboard
</Command.Item>
<Command.Item onSelect={() => navigate('/profile')}>
View Profile
</Command.Item>
</Command.List>
</Command>
)
}
This integration allows users to quickly navigate your application using the command palette, enhancing the overall user experience.
Combining CMDK with State Management
For applications using state management solutions like Redux or MobX, you can connect your command palette to your global state:
import { useSelector, useDispatch } from 'react-redux'
const CommandPalette = () => {
const dispatch = useDispatch()
const todos = useSelector(state => state.todos)
return (
<Command>
<Command.Input />
<Command.List>
{todos.map(todo => (
<Command.Item
key={todo.id}
onSelect={() => dispatch({ type: 'TOGGLE_TODO', id: todo.id })}
>
{todo.text}
</Command.Item>
))}
</Command.List>
</Command>
)
}
This example demonstrates how you can use CMDK to interact with your application’s state, allowing users to perform actions directly from the command palette.
Performance Considerations
While CMDK is designed to be fast out of the box, there are a few things to keep in mind when working with large datasets:
-
Virtualization: For lists with thousands of items, consider implementing virtualization to render only the visible items. Libraries like
react-window
can be helpful here. -
Debouncing Search: If your command palette triggers API calls on search, implement debouncing to reduce unnecessary network requests.
-
Memoization: Use React’s
useMemo
anduseCallback
hooks to optimize rendering performance, especially for complex filtering or item rendering logic.
Accessibility: Ensuring Universal Usability
CMDK is built with accessibility in mind, but it’s important to ensure your implementation maintains these standards:
- Provide clear, descriptive labels for all interactive elements.
- Ensure keyboard navigation works smoothly throughout the command palette.
- Test your command palette with screen readers to verify it’s usable for visually impaired users.
Conclusion: Empowering Users with CMDK
Implementing a command palette with CMDK can significantly enhance the user experience of your React application. By providing a fast, accessible, and customizable interface for navigation and actions, you empower users to interact with your app more efficiently.
As you continue to explore CMDK, remember that the key to a great command palette lies in understanding your users’ needs and tailoring the experience accordingly. Whether you’re building a complex web application or a simple tool, CMDK offers the flexibility and power to create intuitive, keyboard-centric interfaces that users will love.
By mastering CMDK, you’re not just adding a feature to your application; you’re revolutionizing how users interact with your software. So go ahead, dive deeper into CMDK’s capabilities, experiment with different configurations, and create command palettes that truly set your React applications apart.
For more insights on enhancing your React applications, check out our articles on mastering React modals with react-modal and elevating UI with React Aria. These complementary techniques can further boost your application’s usability and accessibility, working in harmony with your CMDK-powered command palette.