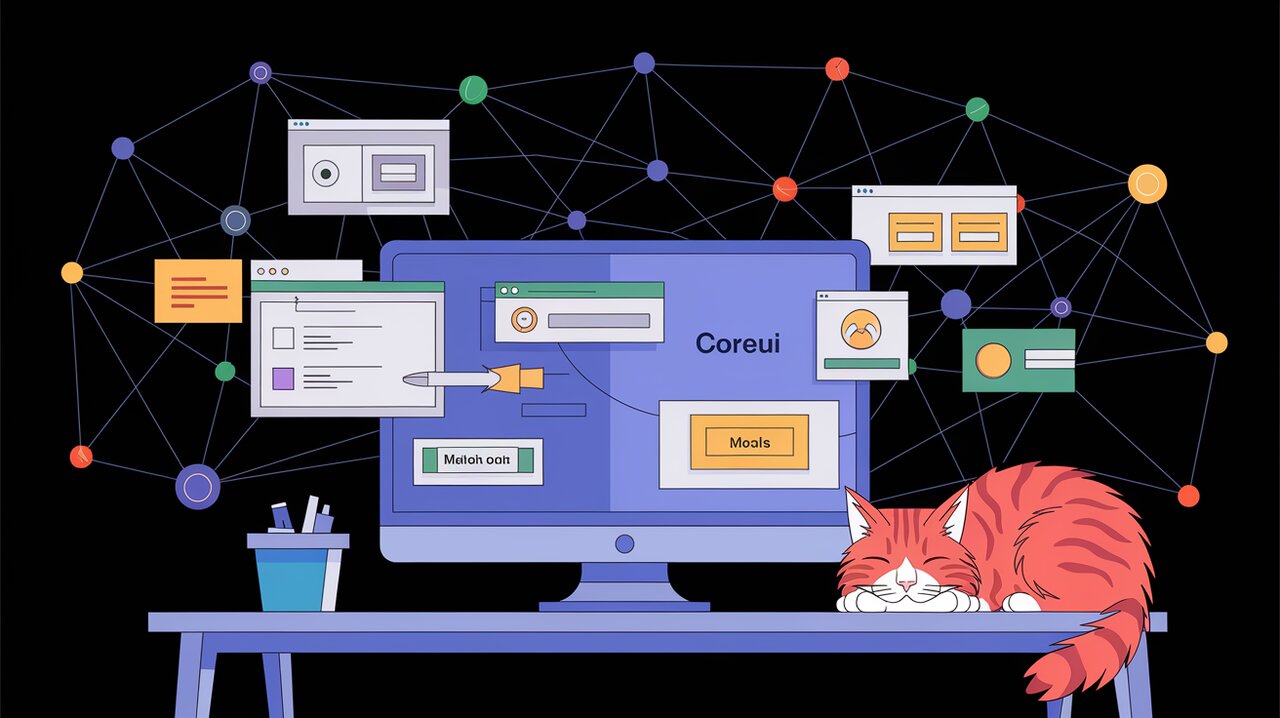
CoreUI for React: Orchestrating UI Symphonies with Ease
CoreUI for React is a powerful and versatile UI library that brings together the best of Bootstrap 5 and React to create stunning, responsive web applications. This comprehensive toolkit offers developers a wide array of pre-built components and utilities, streamlining the process of building modern, user-friendly interfaces.
Harmonizing Your UI with CoreUI
CoreUI for React provides a symphony of components that work seamlessly together to create cohesive and visually appealing user interfaces. From basic elements like buttons and forms to more complex structures like navbars and modals, CoreUI offers everything you need to orchestrate a beautiful frontend.
Key Features
- Bootstrap 5 Foundation: Built on the latest version of Bootstrap, ensuring responsive and mobile-first designs.
- React-Specific Implementation: Optimized for React applications, allowing for seamless integration with your existing projects.
- Extensive Component Library: A wide range of customizable components to suit various UI needs.
- TypeScript Support: Fully typed components for enhanced development experience and type safety.
- Accessibility: Components are designed with accessibility in mind, adhering to WCAG guidelines.
Setting the Stage: Installation and Setup
Getting started with CoreUI for React is a straightforward process. You can quickly add it to your project using npm or yarn.
Installation
To install CoreUI for React, run one of the following commands in your project directory:
npm install @coreui/react
or if you prefer yarn:
yarn add @coreui/react
Styling Your Application
CoreUI components are styled using the @coreui/coreui
CSS library. To include the styles in your project, you’ll need to import the CSS file:
import '@coreui/coreui/dist/css/coreui.min.css'
Alternatively, if you’re using Bootstrap styles, you can import those instead:
import "bootstrap/dist/css/bootstrap.min.css";
Composing Your UI: Basic Usage
Let’s explore how to use some of CoreUI’s components to create a simple interface.
Creating a Button
The CButton
component allows you to easily create stylish, customizable buttons:
import React from 'react';
import { CButton } from '@coreui/react';
const MyComponent = () => {
return (
<CButton color="primary" size="lg">
Click me!
</CButton>
);
};
This code snippet creates a large, primary-colored button with the text “Click me!“.
Building a Card
The CCard
component is perfect for displaying content in a boxed format:
import React from 'react';
import { CCard, CCardBody, CCardTitle, CCardText, CButton } from '@coreui/react';
const MyCard = () => {
return (
<CCard style={{ width: '18rem' }}>
<CCardBody>
<CCardTitle>Card Title</CCardTitle>
<CCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CCardText>
<CButton href="#">Go somewhere</CButton>
</CCardBody>
</CCard>
);
};
This example creates a card with a title, some text, and a button, demonstrating how CoreUI components can be nested to create more complex UI elements.
Advanced Compositions: Creating Dynamic UIs
CoreUI for React shines when it comes to building more complex, interactive interfaces. Let’s look at a few advanced examples.
Responsive Grid System
CoreUI’s grid system, based on Bootstrap’s flexbox grid, allows for easy creation of responsive layouts:
import React from 'react';
import { CContainer, CRow, CCol } from '@coreui/react';
const ResponsiveLayout = () => {
return (
<CContainer>
<CRow>
<CCol sm={8}>Main Content</CCol>
<CCol sm={4}>Sidebar</CCol>
</CRow>
</CContainer>
);
};
This layout will automatically adjust based on screen size, with the main content taking up 2/3 of the width on small screens and larger.
Interactive Modal
Creating an interactive modal is straightforward with CoreUI:
import React, { useState } from 'react';
import { CButton, CModal, CModalHeader, CModalBody, CModalFooter } from '@coreui/react';
const InteractiveModal = () => {
const [visible, setVisible] = useState(false);
return (
<>
<CButton onClick={() => setVisible(!visible)}>Launch Modal</CButton>
<CModal visible={visible} onClose={() => setVisible(false)}>
<CModalHeader closeButton>Modal Title</CModalHeader>
<CModalBody>
This is the modal body where you can put your content.
</CModalBody>
<CModalFooter>
<CButton color="secondary" onClick={() => setVisible(false)}>
Close
</CButton>
<CButton color="primary">Save changes</CButton>
</CModalFooter>
</CModal>
</>
);
};
This code creates a button that, when clicked, opens a modal with a header, body, and footer, complete with close functionality.
Conclusion: The Grand Finale
CoreUI for React provides a robust set of tools for creating beautiful, responsive user interfaces with ease. Its integration of Bootstrap 5 with React-specific optimizations makes it an excellent choice for developers looking to build modern web applications quickly and efficiently.
By leveraging CoreUI’s extensive component library and customization options, you can orchestrate stunning UIs that are both functional and visually appealing. Whether you’re building a simple landing page or a complex dashboard, CoreUI for React offers the flexibility and power to bring your vision to life.
As you continue to explore CoreUI, you might find it helpful to delve into related topics. For instance, our article on Bootstrapping Your React App with React-Bootstrap offers insights into another popular UI library for React. Additionally, if you’re interested in enhancing your forms, check out our guide on Taming Forms with Simple Redux Form.
With CoreUI for React, you have all the instruments you need to compose a symphony of UI elements that will delight your users and streamline your development process. Start building your masterpiece today!