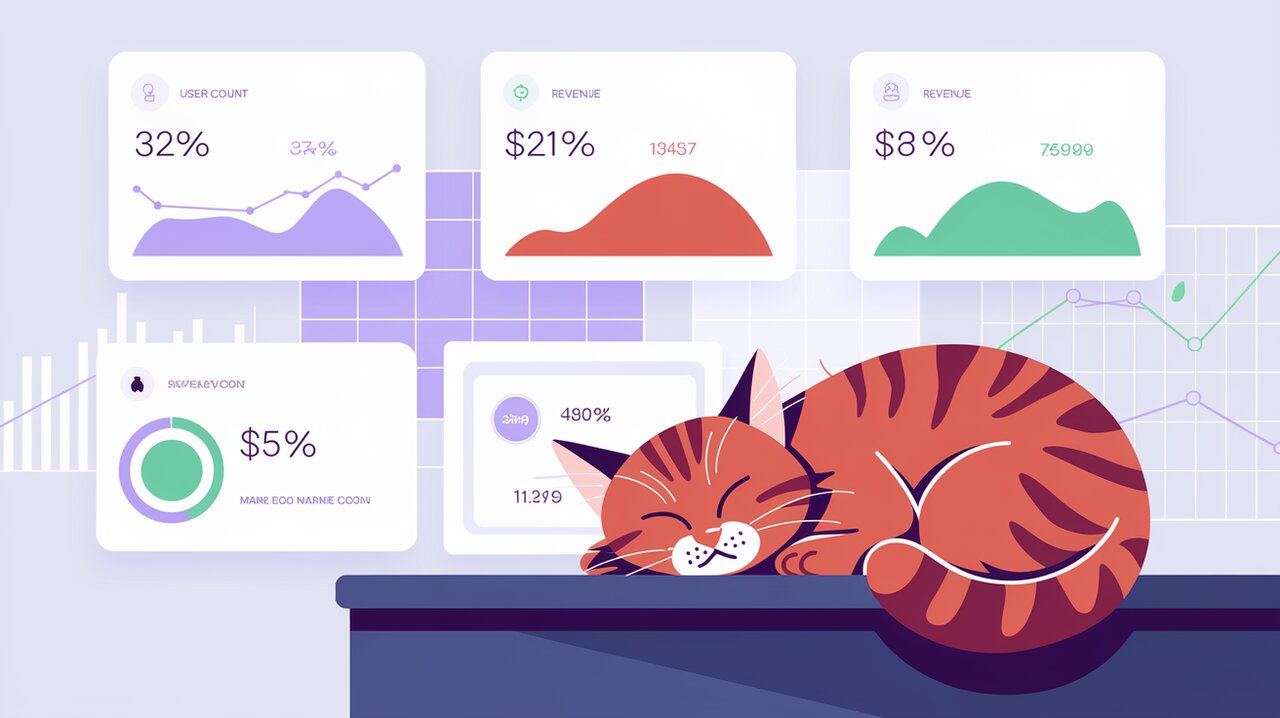
React CountUp is a powerful and flexible library that allows you to easily add number counting animations to your React applications. Whether you’re building a dashboard, showcasing statistics, or simply want to add some visual flair to your numbers, react-countup
provides an elegant solution. In this article, we’ll explore how to use this library effectively, from basic implementations to more advanced use cases.
Features
react-countup
offers a range of features that make it a versatile tool for developers:
- Smooth animations with customizable duration and easing functions
- Support for decimals, prefixes, and suffixes
- Ability to format numbers with separators
- Start, pause, resume, and reset controls
- Render prop API for maximum flexibility
- Scroll-triggered animations
- TypeScript support
Installation
To get started with react-countup
, you’ll need to install it in your React project. You can do this using npm or yarn:
npm install react-countup
or
yarn add react-countup
Basic Usage
Simple Counter
Let’s start with a basic example of how to use react-countup
:
import React from 'react';
import CountUp from 'react-countup';
const SimpleCounter = () => {
return <CountUp end={1000} />;
};
This will create a counter that animates from 0 to 1000. By default, the animation starts as soon as the component mounts and lasts for 2 seconds.
Customizing the Counter
You can easily customize the behavior of the counter by passing additional props:
import React from 'react';
import CountUp from 'react-countup';
const CustomCounter = () => {
return (
<CountUp
start={0}
end={5000}
duration={2.5}
separator=","
decimals={2}
decimal="."
prefix="$"
suffix=" USD"
/>
);
};
This example creates a more complex counter that:
- Starts at 0 and ends at 5000
- Takes 2.5 seconds to complete
- Uses commas as thousand separators
- Shows 2 decimal places
- Uses a dot as the decimal separator
- Adds a dollar sign prefix and “USD” suffix
Advanced Usage
Using the Render Prop API
For more control over the counter, you can use the render prop API:
import React from 'react';
import CountUp from 'react-countup';
const ControlledCounter = () => {
return (
<CountUp start={0} end={1000} duration={3}>
{({ countUpRef, start }) => (
<div>
<span ref={countUpRef} />
<button onClick={start}>Start</button>
</div>
)}
</CountUp>
);
};
This approach gives you access to the counter’s internal methods, allowing you to control when the animation starts and providing a reference to the element being updated.
Scroll-Triggered Animation
react-countup
can also start the animation when the element comes into view:
import React from 'react';
import CountUp from 'react-countup';
const ScrollTriggeredCounter = () => {
return (
<CountUp end={1000} enableScrollSpy scrollSpyOnce>
{({ countUpRef }) => (
<div>
<span ref={countUpRef} />
</div>
)}
</CountUp>
);
};
This counter will start animating when it becomes visible in the viewport and will only trigger once.
Using the Hook API
For even more flexibility, you can use the useCountUp
hook:
import React from 'react';
import { useCountUp } from 'react-countup';
const HookCounter = () => {
const countUpRef = React.useRef(null);
const { start, pauseResume, reset, update } = useCountUp({
ref: countUpRef,
start: 0,
end: 1000,
duration: 5,
onReset: () => console.log('Counter Reset'),
onUpdate: () => console.log('Counter Updated'),
onPauseResume: () => console.log('Counter Paused or Resumed'),
onStart: ({ pauseResume }) => console.log(pauseResume),
onEnd: ({ pauseResume }) => console.log(pauseResume),
});
return (
<div>
<div ref={countUpRef} />
<button onClick={start}>Start</button>
<button onClick={pauseResume}>Pause/Resume</button>
<button onClick={reset}>Reset</button>
<button onClick={() => update(2000)}>Update to 2000</button>
</div>
);
};
This example demonstrates how to use the hook to gain full control over the counter, including starting, pausing, resuming, resetting, and updating the end value.
Best Practices
When using react-countup
, keep these tips in mind:
-
Performance: For large numbers of counters, consider using the
useCountUp
hook to avoid unnecessary re-renders. -
Accessibility: Use ARIA attributes to ensure your counters are accessible. The library provides a
containerProps
prop for this purpose. -
Responsive Design: Adjust the duration and end values based on screen size for a better mobile experience.
-
Testing: When writing tests, you may need to mock the animation or use a library like
jest-matchmedia-mock
to simulate scroll events.
Conclusion
react-countup
is a versatile and easy-to-use library that can add a touch of dynamism to your React applications. Whether you’re creating a simple counter or a complex, interactive dashboard, this library provides the tools you need to bring your numbers to life. By leveraging its various APIs and customization options, you can create engaging user experiences that make data presentation more exciting and impactful.
Remember to consider performance and accessibility when implementing counters, especially in larger applications. With react-countup
, you have the flexibility to balance aesthetics and functionality, ensuring that your animated numbers not only look great but also contribute to a smooth and inclusive user experience.