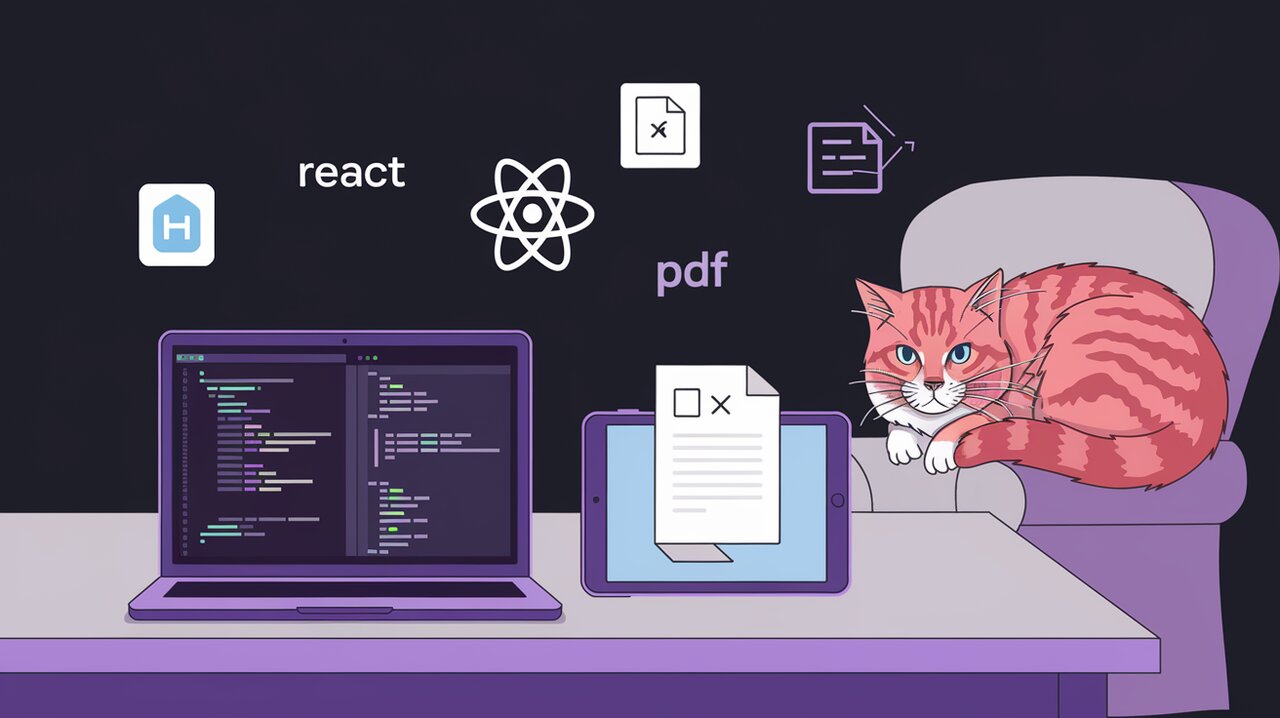
Crafting PDFs with React: Unleashing the Power of @react-pdf/renderer
Introduction to @react-pdf/renderer
@react-pdf/renderer
is a powerful React library that enables developers to create PDF documents programmatically using familiar React components and concepts. Whether you need to generate reports, invoices, or any other type of document, this library provides a flexible and intuitive way to craft PDFs directly within your React applications.
Features
@react-pdf/renderer
offers a range of features that make PDF generation in React a breeze:
- React-based syntax: Utilize JSX to describe your PDF layout, making it feel natural for React developers.
- Cross-platform compatibility: Generate PDFs on both the client-side (browser) and server-side (Node.js).
- Customizable styling: Apply styles to your PDF elements using a subset of CSS properties.
- Dynamic content: Create PDFs with dynamic data and conditional rendering.
- Reusable components: Build and use custom components for consistent document structures.
- Text wrapping and pagination: Automatically handle text overflow and page breaks.
- Image support: Embed images from various sources, including URLs and base64 data.
Installation
To get started with @react-pdf/renderer, you’ll need to install it in your React project. You can do this using npm or yarn:
npm install @react-pdf/renderer
or
yarn add @react-pdf/renderer
Basic Usage
Let’s dive into a simple example to demonstrate how to create a PDF document using @react-pdf/renderer.
Creating a Simple Document
import React from 'react';
import { Document, Page, Text, View, StyleSheet } from '@react-pdf/renderer';
// Define styles
const styles = StyleSheet.create({
page: {
flexDirection: 'row',
backgroundColor: '#E4E4E4'
},
section: {
margin: 10,
padding: 10,
flexGrow: 1
}
});
// Create Document Component
const MyDocument = () => (
<Document>
<Page size="A4" style={styles.page}>
<View style={styles.section}>
<Text>Section 1</Text>
</View>
<View style={styles.section}>
<Text>Section 2</Text>
</View>
</Page>
</Document>
);
This example creates a simple PDF with two sections on a single page. The StyleSheet.create
method is used to define styles, similar to how you would style components in React Native.
Rendering the PDF
To render the PDF in a browser, you can use the PDFViewer
component:
import React from 'react';
import ReactDOM from 'react-dom';
import { PDFViewer } from '@react-pdf/renderer';
import MyDocument from './MyDocument';
const App = () => (
<PDFViewer>
<MyDocument />
</PDFViewer>
);
ReactDOM.render(<App />, document.getElementById('root'));
For server-side rendering or generating a PDF file, you can use the ReactPDF.render
method:
import ReactPDF from '@react-pdf/renderer';
import MyDocument from './MyDocument';
ReactPDF.render(<MyDocument />, `${__dirname}/example.pdf`);
Advanced Usage
Now that we’ve covered the basics, let’s explore some more advanced features of @react-pdf/renderer.
Dynamic Content
One of the powerful features of @react-pdf/renderer
is the ability to generate dynamic content. Here’s an example of how you can create a list of items dynamically:
import React from 'react';
import { Document, Page, Text, View, StyleSheet } from '@react-pdf/renderer';
const styles = StyleSheet.create({
page: { padding: 30 },
item: { marginBottom: 10 }
});
const ItemList = ({ items }) => (
<Document>
<Page size="A4" style={styles.page}>
{items.map((item, index) => (
<View key={index} style={styles.item}>
<Text>{item}</Text>
</View>
))}
</Page>
</Document>
);
// Usage
const items = ['Apple', 'Banana', 'Orange', 'Mango'];
<ItemList items={items} />
This example demonstrates how you can use props and mapping to create a dynamic list of items in your PDF.
Custom Fonts
@react-pdf/renderer
allows you to use custom fonts in your PDFs. Here’s how you can register and use a custom font:
import React from 'react';
import { Document, Page, Text, Font, StyleSheet } from '@react-pdf/renderer';
// Register font
Font.register({
family: 'Oswald',
src: 'https://fonts.gstatic.com/s/oswald/v13/Y_TKV6o8WovbUd3m_X9aAA.ttf'
});
// Create styles
const styles = StyleSheet.create({
title: {
fontSize: 24,
fontFamily: 'Oswald'
}
});
// Create Document Component
const MyDocument = () => (
<Document>
<Page>
<Text style={styles.title}>Custom Font Document</Text>
</Page>
</Document>
);
Page Numbers
Adding page numbers to your PDF is straightforward with @react-pdf/renderer
:
import React from 'react';
import { Document, Page, Text, View, StyleSheet } from '@react-pdf/renderer';
const styles = StyleSheet.create({
page: { padding: 30 },
pageNumber: {
position: 'absolute',
fontSize: 12,
bottom: 30,
left: 0,
right: 0,
textAlign: 'center',
color: 'grey',
},
});
const MyDocument = () => (
<Document>
<Page style={styles.page}>
<Text>Page Content</Text>
<Text style={styles.pageNumber} render={({ pageNumber, totalPages }) => (
`${pageNumber} / ${totalPages}`
)} fixed />
</Page>
</Document>
);
This example adds a page number at the bottom of each page, showing the current page number and total pages.
Conclusion
@react-pdf/renderer
is a powerful tool for generating PDFs in React applications. Its React-based approach makes it intuitive for developers familiar with React, while its extensive feature set allows for the creation of complex, dynamic documents.
Whether you’re creating invoices, reports, or any other type of document, @react-pdf/renderer
provides the flexibility and ease of use to streamline your PDF generation process. By leveraging React components and styles, you can create beautiful, consistent PDFs that integrate seamlessly with your React applications.
As you continue to explore @react-pdf/renderer, you’ll discover even more advanced features and optimizations that can help you create sophisticated PDF documents tailored to your specific needs. Happy PDF crafting!