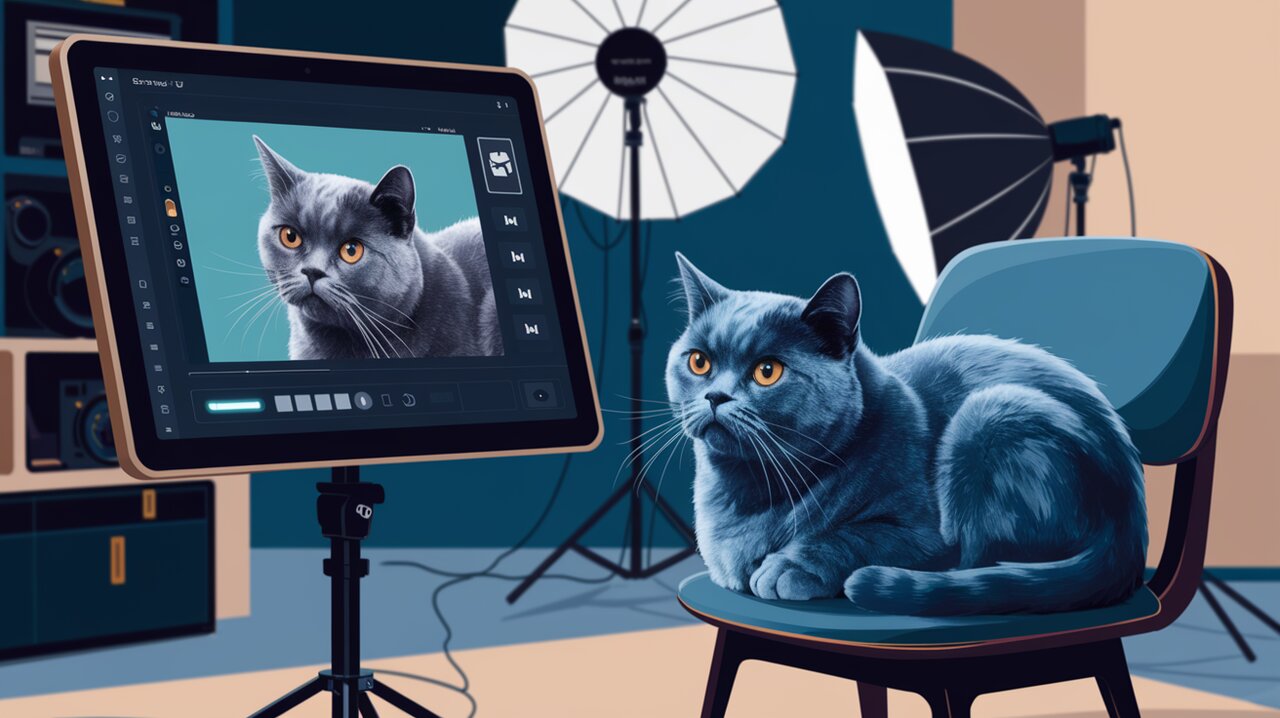
Crop Like a Pro: Mastering Image Manipulation with react-image-crop
React Image Crop is a powerful and flexible library that enables developers to add image cropping functionality to their React applications with ease. This lightweight, dependency-free solution provides a user-friendly interface for selecting and manipulating crop areas on images, making it an excellent choice for projects that require image editing capabilities.
Features
React Image Crop comes packed with a variety of features that make it stand out:
- Responsive Design: The library supports both pixel and percentage-based dimensions, ensuring your cropping interface adapts seamlessly to different screen sizes.
- Touch-Enabled: Users can interact with the cropping tool on touch devices, providing a smooth experience across platforms.
- Flexible Crop Shapes: Developers can implement free-form or fixed aspect ratio crops, catering to various use cases.
- Accessibility: The library is fully keyboard accessible, adhering to a11y standards and making it usable for all users.
- Lightweight: With a small footprint of less than 5KB gzipped, it won’t bloat your application.
- Customizable Crop Sizes: Set minimum and maximum crop sizes to control user input.
- Versatile Application: While primarily used for images, React Image Crop can be applied to crop any content within a container.
Installation
To get started with React Image Crop, you can install it using your preferred package manager:
npm install react-image-crop
# or
yarn add react-image-crop
# or
pnpm add react-image-crop
Basic Usage
Let’s dive into the basics of using React Image Crop in your project.
Setting Up the Component
First, import the necessary modules and styles:
import React, { useState } from 'react'
import ReactCrop, { type Crop } from 'react-image-crop'
import 'react-image-crop/dist/ReactCrop.css'
Next, create a basic component that uses React Image Crop:
const ImageCropper: React.FC = () => {
const [crop, setCrop] = useState<Crop>()
return (
<ReactCrop crop={crop} onChange={(c) => setCrop(c)}>
<img src="/path/to/your/image.jpg" alt="Crop me" />
</ReactCrop>
)
}
This simple setup creates a cropping interface around your image. The crop
state holds the current crop selection, which is updated via the onChange
callback.
Handling Crop Changes
To make use of the crop data, you can add a function to handle the crop completion:
const ImageCropper: React.FC = () => {
const [crop, setCrop] = useState<Crop>()
const handleCompletedCrop = (c: Crop) => {
console.log('Completed crop:', c)
// Here you can send the crop data to a server or process it further
}
return (
<ReactCrop crop={crop} onChange={(c) => setCrop(c)} onComplete={handleCompletedCrop}>
<img src="/path/to/your/image.jpg" alt="Crop me" />
</ReactCrop>
)
}
The onComplete
prop is called when the user finishes dragging the crop selection, providing you with the final crop data.
Advanced Usage
Now that we’ve covered the basics, let’s explore some more advanced features of React Image Crop.
Fixed Aspect Ratio
To enforce a specific aspect ratio for the crop selection, you can use the aspect
prop:
const ImageCropper: React.FC = () => {
const [crop, setCrop] = useState<Crop>({
unit: '%',
width: 50,
aspect: 16 / 9,
})
return (
<ReactCrop crop={crop} onChange={(c) => setCrop(c)} aspect={16 / 9}>
<img src="/path/to/your/image.jpg" alt="Crop me" />
</ReactCrop>
)
}
This will constrain the crop selection to a 16:9 aspect ratio, which is common for video thumbnails or widescreen images.
Minimum and Maximum Crop Sizes
You can set limits on the crop size to ensure users select an appropriate area:
const ImageCropper: React.FC = () => {
const [crop, setCrop] = useState<Crop>()
return (
<ReactCrop
crop={crop}
onChange={(c) => setCrop(c)}
minWidth={100}
minHeight={100}
maxWidth={300}
maxHeight={300}
>
<img src="/path/to/your/image.jpg" alt="Crop me" />
</ReactCrop>
)
}
This configuration ensures that the crop selection is between 100x100 and 300x300 pixels.
Circular Crops
For profile pictures or circular UI elements, you can create a circular crop:
const ImageCropper: React.FC = () => {
const [crop, setCrop] = useState<Crop>({
unit: '%',
width: 50,
aspect: 1,
})
return (
<ReactCrop
crop={crop}
onChange={(c) => setCrop(c)}
aspect={1}
circularCrop
>
<img src="/path/to/your/image.jpg" alt="Crop me" />
</ReactCrop>
)
}
The circularCrop
prop, combined with an aspect ratio of 1, creates a perfect circle selection.
Generating a Crop Preview
To show users a preview of their crop selection, you can use a canvas element:
const ImageCropper: React.FC = () => {
const [crop, setCrop] = useState<Crop>()
const [completedCrop, setCompletedCrop] = useState<Crop>()
const imgRef = useRef<HTMLImageElement>(null)
const previewCanvasRef = useRef<HTMLCanvasElement>(null)
useEffect(() => {
if (!completedCrop || !imgRef.current || !previewCanvasRef.current) {
return
}
const image = imgRef.current
const canvas = previewCanvasRef.current
const crop = completedCrop
const scaleX = image.naturalWidth / image.width
const scaleY = image.naturalHeight / image.height
const ctx = canvas.getContext('2d')
if (!ctx) {
return
}
canvas.width = crop.width
canvas.height = crop.height
ctx.drawImage(
image,
crop.x * scaleX,
crop.y * scaleY,
crop.width * scaleX,
crop.height * scaleY,
0,
0,
crop.width,
crop.height
)
}, [completedCrop])
return (
<div>
<ReactCrop
crop={crop}
onChange={(c) => setCrop(c)}
onComplete={(c) => setCompletedCrop(c)}
>
<img ref={imgRef} src="/path/to/your/image.jpg" alt="Crop me" />
</ReactCrop>
<canvas
ref={previewCanvasRef}
style={{
border: '1px solid black',
objectFit: 'contain',
width: completedCrop?.width,
height: completedCrop?.height,
}}
/>
</div>
)
}
This example creates a live preview of the crop selection using a canvas element, updating it whenever the crop is completed.
Conclusion
React Image Crop provides a powerful toolset for implementing image cropping functionality in React applications. From basic setups to advanced features like aspect ratio constraints and circular crops, this library offers flexibility and ease of use for developers.
By leveraging React Image Crop, you can create intuitive and responsive image editing interfaces that enhance user experience and streamline content creation processes. Whether you’re building a social media platform, a content management system, or any application that deals with user-uploaded images, React Image Crop is a valuable addition to your toolkit.
Remember to explore the library’s documentation for even more features and customization options. Happy cropping!