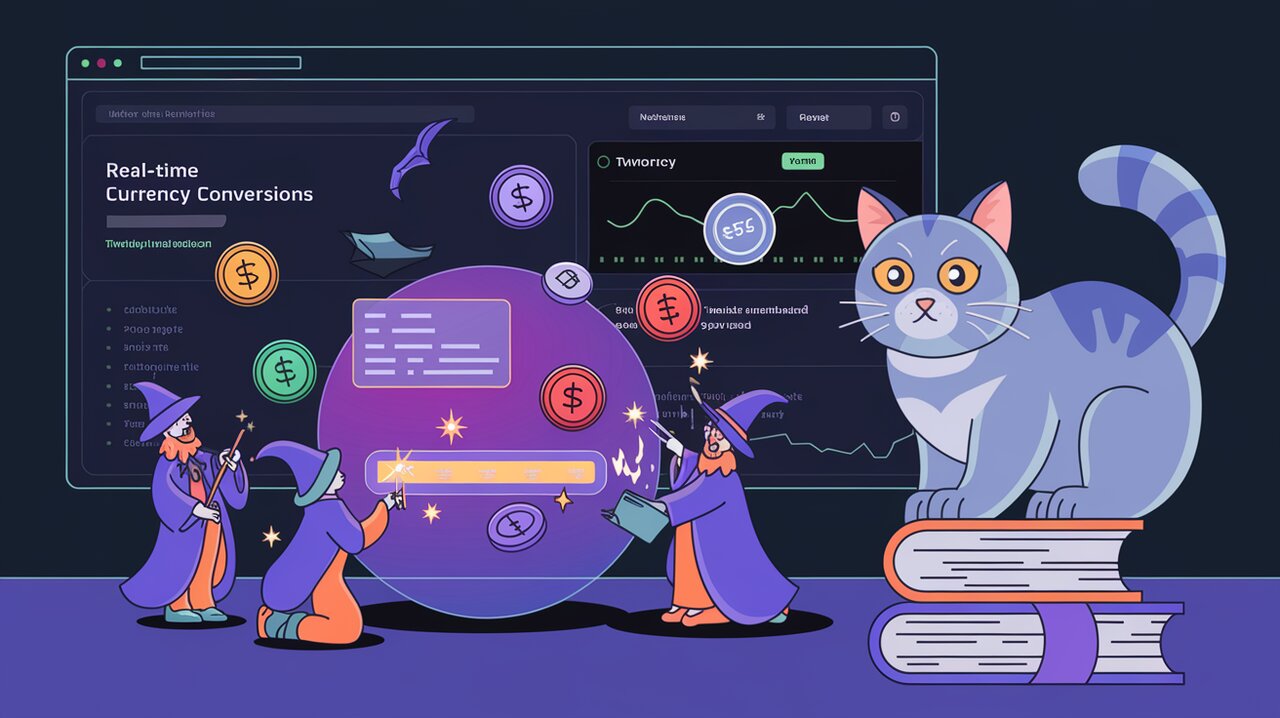
Currency Wizardry: Mastering React Currency Input Field
React Currency Input Field is a powerful and flexible component that brings the magic of currency handling to your React applications. Whether you’re building a financial dashboard, an e-commerce platform, or any application that deals with monetary values, this library offers a comprehensive solution for managing currency inputs with ease and precision.
Unveiling the Enchanted Features
React Currency Input Field comes packed with an array of features that make it a must-have tool in any React developer’s arsenal:
- Abbreviation Support: Easily handle shorthand notations like “1k” for 1,000 or “2.5m” for 2,500,000.
- Prefix and Suffix Options: Add currency symbols or other indicators before or after the input value.
- Automatic Formatting: Seamlessly insert group separators (e.g., commas for thousands) as the user types.
- Internationalization: Leverage Intl locale configurations for globally-aware formatting.
- Decimal Control: Allow or disallow decimals, and set limits on decimal places.
- Keyboard Navigation: Use arrow keys to increment or decrement values.
- TypeScript Support: Enjoy full type support for enhanced development experience.
Conjuring the Component: Installation
To begin your journey with React Currency Input Field, you’ll need to summon it into your project. Open your terminal and cast one of the following spells:
npm install react-currency-input-field
Or if you prefer the yarn incantation:
yarn add react-currency-input-field
Casting Your First Spell: Basic Usage
Now that you have the component at your fingertips, let’s create a simple currency input:
import React from 'react';
import CurrencyInput from 'react-currency-input-field';
const CurrencyInputExample: React.FC = () => {
const handleValueChange = (value: string | undefined, name: string | undefined) => {
console.log(value, name);
};
return (
<CurrencyInput
id="currency-input"
name="currency-input"
placeholder="Enter amount"
defaultValue={1000}
decimalsLimit={2}
onValueChange={handleValueChange}
/>
);
};
export default CurrencyInputExample;
This spell creates a basic currency input with a default value of 1000, limits decimals to two places, and logs any changes to the console.
Advanced Incantations: Customization
Prefix and Suffix Magic
Add currency symbols or other indicators to your input:
<CurrencyInput
prefix="$"
suffix=" USD"
defaultValue={1500.75}
decimalsLimit={2}
onValueChange={(value, name) => console.log(value, name)}
/>
International Charm
Adapt your input to different locales:
<CurrencyInput
intlConfig={{ locale: 'de-DE', currency: 'EUR' }}
defaultValue={1234.56}
onValueChange={(value, name) => console.log(value, name)}
/>
Decimal Sorcery
Control the behavior of decimal places:
<CurrencyInput
allowDecimals={true}
decimalsLimit={3}
fixedDecimalLength={2}
defaultValue={100}
onValueChange={(value, name) => console.log(value, name)}
/>
Handling the Magic: Value Changes
The onValueChange
prop is your window into the changing values of the input. It provides three magical parameters:
value
: The current value as a string, sans formatting.name
: The name you assigned to the input.values
: An object containing additional information:
float
: The value as a float (or null if empty).formatted
: The value with applied formatting.value
: The same as the first parameter.
const handleValueChange = (value: string | undefined, name: string | undefined, values: Object | undefined) => {
console.log('Raw value:', value);
console.log('Input name:', name);
console.log('Formatted value:', values?.formatted);
console.log('Float value:', values?.float);
};
Enchanted Formatting: Beyond the Input
Sometimes, you need to format currency values outside of the input component. Fear not, for React Currency Input Field provides a powerful formatValue
function:
import { formatValue } from 'react-currency-input-field';
const formattedValue = formatValue({
value: '1234567.89',
groupSeparator: ',',
decimalSeparator: '.',
prefix: '£',
});
console.log(formattedValue); // Outputs: £1,234,567.89
This function allows you to apply the same formatting magic to any string value, making it perfect for displaying totals or other currency amounts throughout your application.
Conclusion: Mastering the Currency Arts
React Currency Input Field is a powerful ally in the quest for seamless currency handling in React applications. Its flexibility, extensive feature set, and ease of use make it an invaluable tool for developers working with monetary values.
As you continue your journey in the realm of React development, consider exploring other magical components that can enhance your applications. The React Select library offers advanced dropdown capabilities that pair well with currency inputs, while the React Datepicker can help you handle date inputs for financial applications.
By mastering React Currency Input Field, you’ve added a powerful spell to your developer’s spellbook. Use it wisely, and watch as your forms transform from mundane inputs to enchanted interfaces that delight users and simplify complex currency handling tasks.