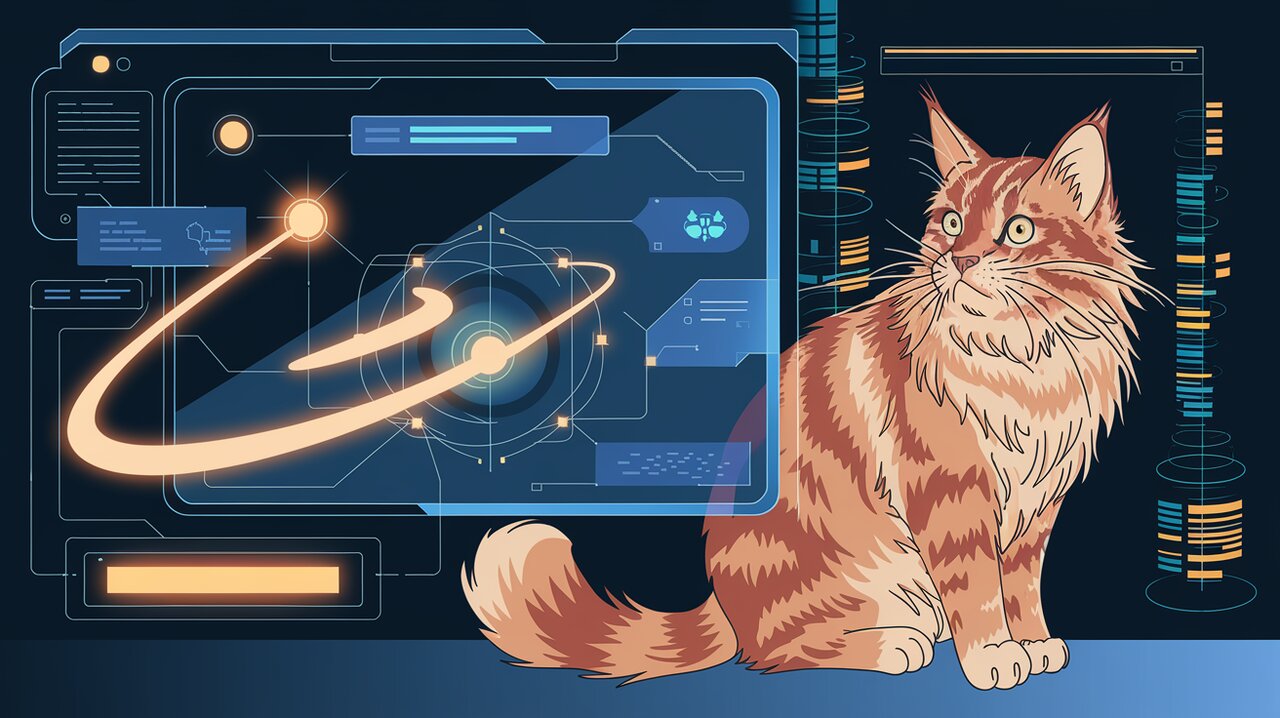
Cursor Position Wizardry: Unleashing the Power of react-cursor-position
React developers, prepare to be enchanted! Today, we’re diving into the magical world of react-cursor-position
, a powerful library that brings cursor and touch position awareness to your React components. Whether you’re building interactive visualizations, custom tooltips, or just want to add some extra pizzazz to your UI, this library is your ticket to creating truly responsive and engaging user experiences.
Conjuring the Cursor: What is react-cursor-position?
react-cursor-position is a primitive component that acts as a foundation for building UI features that need to know about cursor or touch positions. It’s like giving your components a sixth sense, allowing them to react to the user’s every move. The library plots position coordinates relative to the HTML element it renders, and then re-renders child components with updated position props whenever the cursor or touch position changes.
Features That Will Cast a Spell on You
- Versatile Activation: Supports activation by Press, Tap, Touch, Hover, and Click gestures.
- Scroll-Aware: Maintains accuracy even during scroll position changes.
- Environment Detection: Automatically detects mouse and touch environments.
- Customizable: Offers a range of props to fine-tune behavior.
Summoning react-cursor-position to Your Project
Let’s start by adding this magical component to your React arsenal. Open your terminal and cast this incantation:
npm install --save react-cursor-position
Or if you prefer yarn:
yarn add react-cursor-position
Basic Usage: Your First Spell
Now that we have react-cursor-position
in our spellbook, let’s cast our first spell. Here’s a simple example of how to use it:
import React from 'react';
import ReactCursorPosition from 'react-cursor-position';
const CursorAwareComponent: React.FC = () => (
<ReactCursorPosition>
{({position}) => (
<div>
Cursor position: ({position.x}, {position.y})
</div>
)}
</ReactCursorPosition>
);
export default CursorAwareComponent;
In this enchanting snippet, we wrap our component with ReactCursorPosition
. It then provides the current cursor position to its children through a render prop. The position
object contains x
and y
coordinates relative to the component’s boundaries.
Advanced Incantations
Gesture Activation
react-cursor-position
allows you to choose how it activates. Let’s modify our spell to activate on click instead of hover:
import React from 'react';
import ReactCursorPosition, { INTERACTIONS } from 'react-cursor-position';
const ClickActivatedComponent: React.FC = () => (
<ReactCursorPosition activationInteractionMouse={INTERACTIONS.CLICK}>
{({isActive, position}) => (
<div>
{isActive ? `Clicked at: (${position.x}, ${position.y})` : 'Click me!'}
</div>
)}
</ReactCursorPosition>
);
export default ClickActivatedComponent;
Custom Child Props
Sometimes, you might want to reshape the props passed to child components. The mapChildProps
prop is your wand for this transformation:
import React from 'react';
import ReactCursorPosition from 'react-cursor-position';
const CustomPropsComponent: React.FC = () => (
<ReactCursorPosition
mapChildProps={({isActive, position}) => ({
isHovering: isActive,
cursorX: position.x,
cursorY: position.y,
})}
>
{({isHovering, cursorX, cursorY}) => (
<div>
{isHovering ? `Cursor at: (${cursorX}, ${cursorY})` : 'Hover over me!'}
</div>
)}
</ReactCursorPosition>
);
export default CustomPropsComponent;
Mastering the Arcane: Tips and Tricks
-
Performance Optimization: Use the
isEnabled
prop to conditionally enable/disable cursor tracking when needed. -
Touch Devices: Customize touch behavior with
activationInteractionTouch
prop. Options includeINTERACTIONS.PRESS
,INTERACTIONS.TAP
, andINTERACTIONS.TOUCH
. -
Scroll Handling: The library automatically handles scroll position changes, ensuring accurate positioning even in scrollable containers.
-
Environment Detection: Access
detectedEnvironment
in child props to adapt your UI based on whether mouse or touch input is detected.
Conclusion: Unleashing Cursor Magic
react-cursor-position
opens up a world of possibilities for creating interactive and responsive React components. From custom tooltips to interactive data visualizations, the applications are limited only by your imagination.
As you continue your journey into the realm of cursor-aware components, consider exploring related React libraries that can complement your newfound powers. The react-dnd library offers drag-and-drop functionality, while react-measure can help you create resizable components.
Remember, with great power comes great responsibility. Use react-cursor-position
wisely, and your users will be spellbound by the magic of your interactive UIs. Happy coding, and may your cursors always point true!