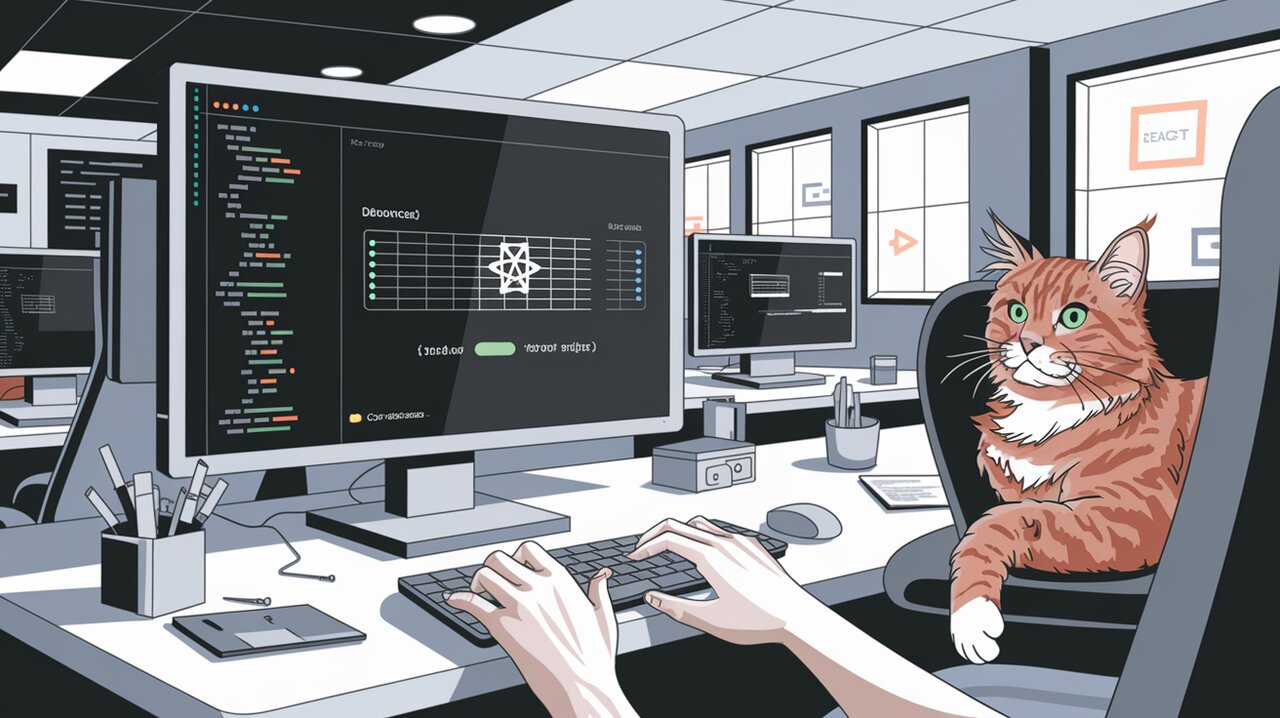
Taming User Input: Mastering Debouncing in React with use-debounce
Understanding Debouncing in React
Debouncing is a programming practice used to ensure that time-consuming tasks don’t fire so often, making it particularly useful in React applications. It’s especially valuable when dealing with user inputs or events that can trigger frequent updates or API calls.
Introducing use-debounce
The use-debounce library is a lightweight, powerful tool for implementing debouncing in React applications. It provides a set of hooks that make it easy to debounce values and callbacks, improving performance and user experience.
Features
- Simple API with React hooks
- Support for debouncing values and callbacks
- Customizable delay and options
- TypeScript support
- Server-side rendering compatibility
Installation
To get started with use-debounce, you can install it using npm or yarn:
npm install use-debounce
or
yarn add use-debounce
Basic Usage
Debouncing Values
The most straightforward way to use use-debounce is with the useDebounce
hook. Here’s a simple example:
import React, { useState } from 'react';
import { useDebounce } from 'use-debounce';
const SearchComponent = () => {
const [searchTerm, setSearchTerm] = useState('');
const [debouncedSearchTerm] = useDebounce(searchTerm, 300);
return (
<div>
<input
type="text"
value={searchTerm}
onChange={(e) => setSearchTerm(e.target.value)}
/>
<p>Debounced search term: {debouncedSearchTerm}</p>
</div>
);
};
In this example, the debouncedSearchTerm
will update 300 milliseconds after the user stops typing. This can be useful for preventing unnecessary API calls or expensive computations while the user is still typing.
Debouncing Callbacks
For more complex scenarios, you might want to debounce a callback function. The useDebouncedCallback
hook is perfect for this:
import React from 'react';
import { useDebouncedCallback } from 'use-debounce';
const AutosaveComponent = () => {
const debouncedSave = useDebouncedCallback(
(value) => {
// Perform save operation
console.log('Saving:', value);
},
500
);
return (
<textarea
onChange={(e) => debouncedSave(e.target.value)}
placeholder="Start typing to autosave..."
/>
);
};
This setup ensures that the save operation is only triggered 500 milliseconds after the user stops typing, reducing the number of save operations and potentially improving performance.
Advanced Usage
Cancelling Debounced Calls
Sometimes you might need to cancel a debounced call. The useDebouncedCallback
hook returns a function with a cancel
method:
const debouncedFunction = useDebouncedCallback(() => {
// Your function logic here
}, 1000);
// Later in your code
debouncedFunction.cancel();
Leading Calls
By default, debounced functions are invoked on the trailing edge of the timeout. If you want to invoke the function on the leading edge instead, you can use the leading
option:
const leadingDebouncedFunction = useDebouncedCallback(
() => {
// Your function logic here
},
1000,
{ leading: true }
);
This configuration will cause the function to be called immediately on the first invocation, and then debounce subsequent calls.
Performance Considerations
While debouncing can significantly improve performance, it’s essential to use it judiciously. Here are some tips:
- Choose appropriate delay times based on your use case.
- Use memoization techniques like
useMemo
anduseCallback
in conjunction with debouncing for optimal performance. - Consider the trade-off between responsiveness and performance when setting delay times.
Conclusion
The use-debounce library provides a powerful and flexible way to implement debouncing in React applications. By leveraging its hooks, you can easily optimize user input handling, API calls, and other time-sensitive operations. Whether you’re building a search interface, an autosave feature, or any other interactive component, use-debounce can help you create smoother, more efficient React applications.
Remember, the key to effective debouncing is finding the right balance between responsiveness and performance. Experiment with different delay times and options to find the sweet spot for your specific use case. With use-debounce in your toolkit, you’re well-equipped to handle the challenges of real-time user interactions in React.