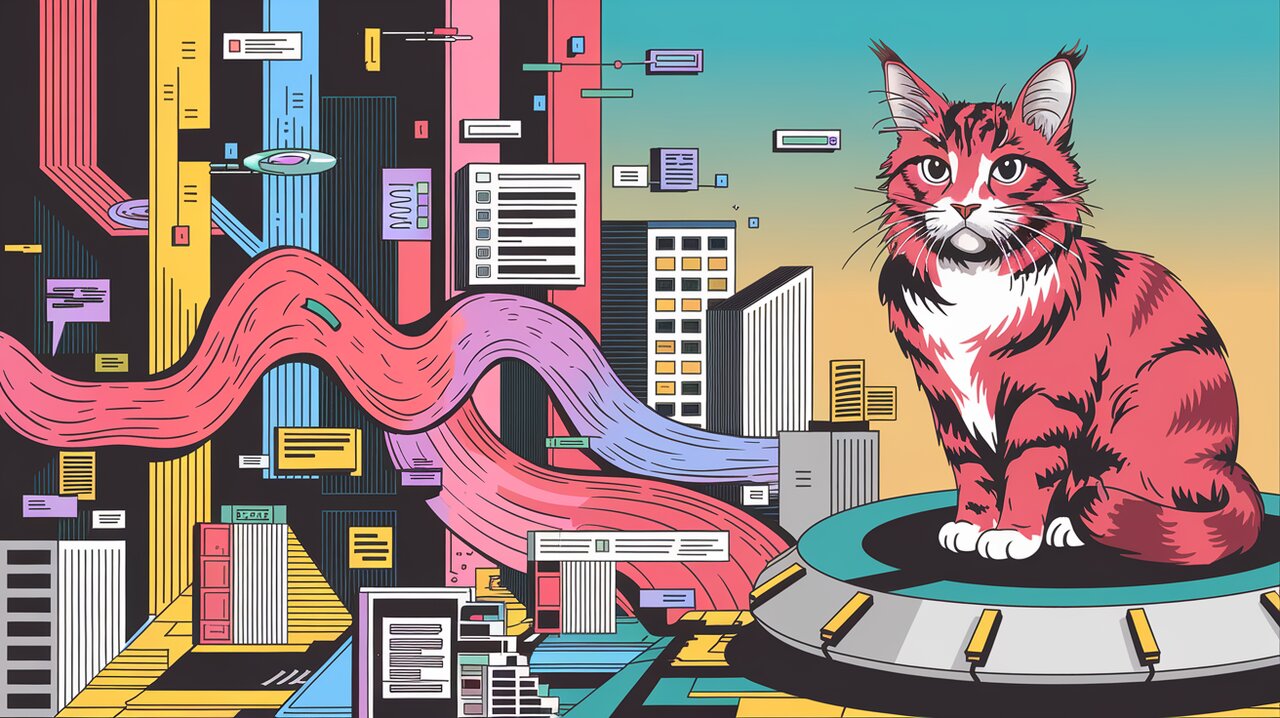
DevExtreme React: Unleashing UI Wizardry in React Applications
DevExtreme React is a comprehensive suite of over 70 responsive and touch-enabled UI components designed specifically for React applications. This powerful library empowers developers to create sophisticated, interactive user interfaces with minimal effort, offering a wide range of components from data grids and charts to forms and navigation elements.
Unleashing the Power of DevExtreme React
At its core, DevExtreme React provides a rich set of features that cater to various aspects of modern web application development:
- Responsive Design: All components are built with responsiveness in mind, ensuring your application looks great on any device.
- Touch-Enabled: Perfect for mobile and tablet applications, with intuitive touch interactions.
- Data Visualization: A wide array of chart types and data visualization tools to bring your data to life.
- Advanced Data Management: Powerful data grid and pivot grid components for complex data manipulation and analysis.
- Customizable Themes: Easily adapt the look and feel of components to match your application’s design.
- Accessibility: Built-in accessibility features to ensure your application is usable by everyone.
Getting Started with DevExtreme React
To begin your journey with DevExtreme React, you’ll need to install the library in your project. You can do this using npm or yarn:
npm install devextreme@24.1 devextreme-react@24.1 --save --save-exact
or
yarn add devextreme@24.1 devextreme-react@24.1 --exact
Crafting Your First DevExtreme React Component
Let’s dive into creating a simple button component to get a feel for how DevExtreme React works:
import React from 'react';
import 'devextreme/dist/css/dx.light.css';
import { Button } from 'devextreme-react/button';
const App: React.FC = () => {
const handleClick = () => {
alert('Hello, DevExtreme React!');
};
return (
<Button
text="Click me"
onClick={handleClick}
stylingMode="contained"
type="success"
/>
);
};
export default App;
In this example, we’ve imported the necessary CSS file and the Button component. We’ve then created a simple functional component that renders a button with custom styling and an onClick handler.
Mastering the Data Grid
One of the most powerful components in DevExtreme React is the Data Grid. Let’s explore how to create a basic data grid with sorting and filtering capabilities:
import React from 'react';
import 'devextreme/dist/css/dx.light.css';
import { DataGrid, Column, Sorting, FilterRow } from 'devextreme-react/data-grid';
const employees = [
{ id: 1, name: 'John Doe', position: 'CEO', salary: 120000 },
{ id: 2, name: 'Jane Smith', position: 'CTO', salary: 110000 },
// ... more data
];
const App: React.FC = () => {
return (
<DataGrid
dataSource={employees}
showBorders={true}
columnAutoWidth={true}
>
<Sorting mode="multiple" />
<FilterRow visible={true} />
<Column dataField="name" caption="Employee Name" />
<Column dataField="position" caption="Job Title" />
<Column dataField="salary" caption="Annual Salary" dataType="number" format="currency" />
</DataGrid>
);
};
export default App;
This code creates a data grid with three columns, enables multi-column sorting, and adds a filter row for easy data searching. The salary column is formatted as currency for better readability.
Visualizing Data with Charts
DevExtreme React offers a variety of chart types to visualize your data effectively. Here’s an example of creating a simple bar chart:
import React from 'react';
import 'devextreme/dist/css/dx.light.css';
import { Chart, Series, ArgumentAxis, ValueAxis, Title } from 'devextreme-react/chart';
const data = [
{ fruit: 'Apples', amount: 10 },
{ fruit: 'Oranges', amount: 15 },
{ fruit: 'Bananas', amount: 9 },
{ fruit: 'Grapes', amount: 7 },
];
const App: React.FC = () => {
return (
<Chart dataSource={data}>
<Series
valueField="amount"
argumentField="fruit"
type="bar"
color="#ffaa66"
/>
<ArgumentAxis title="Fruit" />
<ValueAxis title="Amount" />
<Title text="Fruit Consumption" />
</Chart>
);
};
export default App;
This code creates a bar chart showing fruit consumption data. The chart includes labeled axes and a title, making it easy to understand at a glance.
Building Dynamic Forms
DevExtreme React simplifies form creation with its Form component. Here’s how you can create a dynamic form with validation:
import React from 'react';
import 'devextreme/dist/css/dx.light.css';
import { Form, SimpleItem, ButtonItem } from 'devextreme-react/form';
const App: React.FC = () => {
const formData = {
name: '',
email: '',
age: null,
};
const validationRules = {
name: [{ type: 'required', message: 'Name is required' }],
email: [
{ type: 'required', message: 'Email is required' },
{ type: 'email', message: 'Email is invalid' },
],
age: [
{ type: 'required', message: 'Age is required' },
{ type: 'range', min: 18, max: 100, message: 'Age must be between 18 and 100' },
],
};
const handleSubmit = (e: any) => {
e.preventDefault();
console.log('Form submitted:', formData);
};
return (
<Form
formData={formData}
onSubmit={handleSubmit}
>
<SimpleItem dataField="name" editorType="dxTextBox" validationRules={validationRules.name} />
<SimpleItem dataField="email" editorType="dxTextBox" validationRules={validationRules.email} />
<SimpleItem dataField="age" editorType="dxNumberBox" validationRules={validationRules.age} />
<ButtonItem buttonOptions={{ text: 'Submit', type: 'success', useSubmitBehavior: true }} />
</Form>
);
};
export default App;
This example demonstrates a form with three fields (name, email, and age) along with validation rules. The form automatically handles data binding and validation, streamlining the process of creating complex forms.
Optimizing Performance
DevExtreme React components are designed with performance in mind, but there are ways to further optimize your application. One key technique is to use the deferRendering
prop for complex components:
import React from 'react';
import 'devextreme/dist/css/dx.light.css';
import { DataGrid } from 'devextreme-react/data-grid';
const App: React.FC = () => {
return (
<DataGrid
dataSource={largeDataSet}
deferRendering={true}
// other props...
/>
);
};
export default App;
By setting deferRendering
to true, the component will render its content only when it becomes visible, significantly improving initial load times for applications with many complex components.
Wrapping Up the DevExtreme React Journey
DevExtreme React offers a treasure trove of UI components and features that can significantly accelerate your React application development. From responsive data grids and interactive charts to dynamic forms and performance optimization techniques, this library provides the tools you need to create stunning, efficient user interfaces.
As you continue to explore DevExtreme React, you’ll discover even more advanced features and customization options. Remember to consult the official documentation for in-depth information on each component and to stay updated with the latest releases. With DevExtreme React in your toolkit, you’re well-equipped to tackle even the most challenging UI development tasks in your React projects.