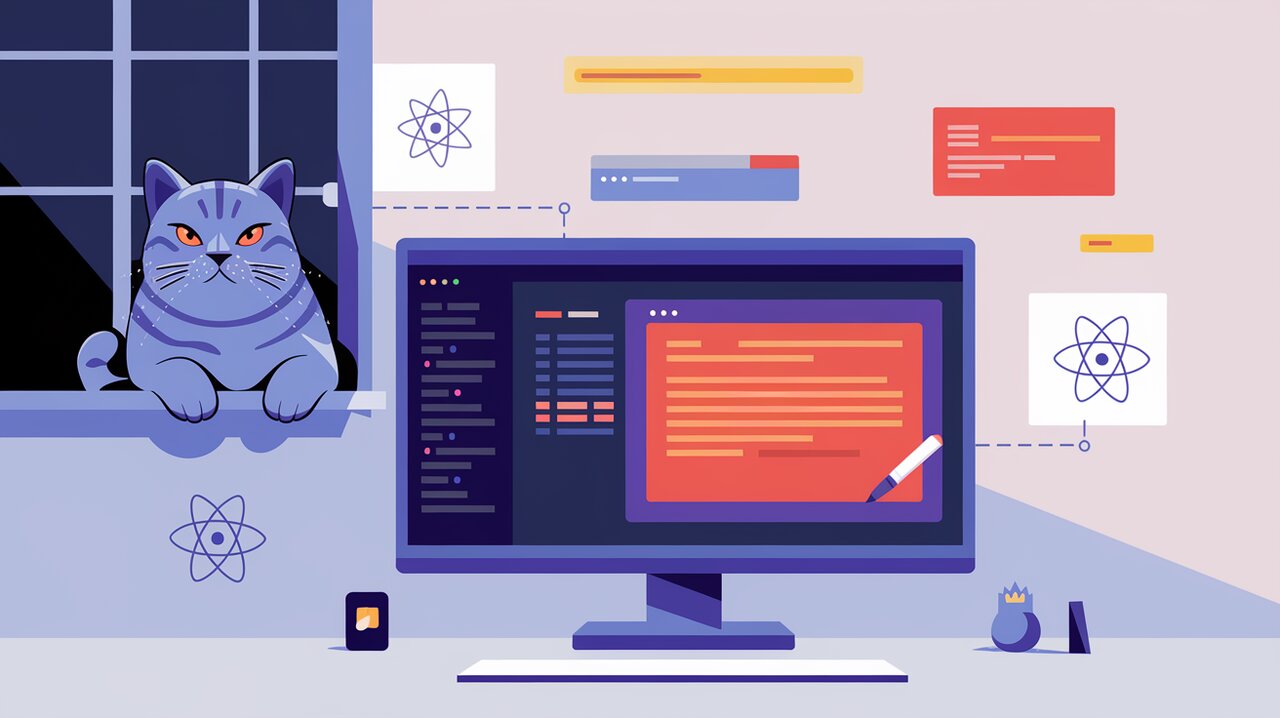
Transforming Static to Dynamic: React-Contenteditable for Interactive Content
In the ever-evolving landscape of web development, creating interactive and user-friendly interfaces is paramount. React-Contenteditable emerges as a powerful tool in a developer’s arsenal, offering a seamless way to transform static div
elements into fully editable content areas. This versatile component opens up a world of possibilities for creating dynamic, engaging user experiences with minimal effort.
Elevating User Interaction
React-Contenteditable brings a host of features to the table, making it an indispensable tool for developers seeking to enhance their React applications:
- Effortless Integration: Seamlessly incorporate editable content areas into your React components.
- HTML Support: Edit and render HTML content directly within your application.
- Customizable Behavior: Fine-tune the editing experience with a variety of props and event handlers.
- Lightweight Solution: With a minimal footprint, it won’t bloat your application.
Setting Up Your Development Environment
Before we dive into the exciting world of editable divs, let’s get our development environment ready to go.
Installation Process
You can easily install React-Contenteditable using either npm or yarn:
# Using npm
npm install react-contenteditable
# Using yarn
yarn add react-contenteditable
Implementing Basic Functionality
Let’s explore how to implement a simple editable div using React-Contenteditable.
Building Your First Editable Component
Here’s a basic example of how to use the ContentEditable
component:
import React, { useState, useRef } from 'react';
import ContentEditable from 'react-contenteditable';
const EditableComponent: React.FC = () => {
const [content, setContent] = useState('<p>Edit me!</p>');
const contentEditableRef = useRef<HTMLElement>(null);
const handleChange = (evt: React.FormEvent<HTMLDivElement>) => {
setContent(evt.currentTarget.innerHTML);
};
return (
<ContentEditable
innerRef={contentEditableRef}
html={content}
disabled={false}
onChange={handleChange}
tagName='article'
/>
);
};
export default EditableComponent;
In this example, we create a functional component that utilizes the ContentEditable
component. We manage the content state using the useState
hook and handle changes with the handleChange
function.
Decoding the Props
Let’s break down the key props used in the ContentEditable
component:
innerRef
: A reference to the underlying DOM element.html
: The HTML content to be rendered and edited.disabled
: A boolean to enable or disable editing.onChange
: A function to handle content changes.tagName
: The HTML tag to use for the editable element (default is ‘div’).
Advanced Techniques and Customizations
Now that we’ve covered the basics, let’s explore some more advanced uses of React-Contenteditable.
Controlling Content Sanitization
When working with user-editable HTML content, it’s crucial to implement proper sanitization to prevent XSS attacks. Here’s an example of how you can sanitize the input:
import DOMPurify from 'dompurify';
const EditableComponentWithSanitization: React.FC = () => {
const [content, setContent] = useState('<p>Edit me safely!</p>');
const handleChange = (evt: React.FormEvent<HTMLDivElement>) => {
const sanitizedContent = DOMPurify.sanitize(evt.currentTarget.innerHTML);
setContent(sanitizedContent);
};
return (
<ContentEditable
html={content}
onChange={handleChange}
/>
);
};
This example uses the DOMPurify
library to sanitize the input, ensuring that any potentially harmful HTML is removed before updating the state.
Implementing Custom Styling
You can easily apply custom styles to your editable content area:
import styled from 'styled-components';
const StyledContentEditable = styled(ContentEditable)`
border: 1px solid #ccc;
padding: 10px;
min-height: 100px;
font-family: Arial, sans-serif;
&:focus {
outline: none;
border-color: #007bff;
}
`;
const StyledEditableComponent: React.FC = () => {
// ... state and handlers
return (
<StyledContentEditable
html={content}
onChange={handleChange}
/>
);
};
This example uses styled-components to create a custom-styled version of the ContentEditable
component.
Handling Paste Events
Sometimes you may want to control how content is pasted into your editable area. Here’s an example of how to handle paste events:
const EditableComponentWithPasteHandler: React.FC = () => {
const [content, setContent] = useState('<p>Paste here!</p>');
const handlePaste = (evt: React.ClipboardEvent<HTMLDivElement>) => {
evt.preventDefault();
const text = evt.clipboardData.getData('text/plain');
document.execCommand('insertText', false, text);
};
return (
<ContentEditable
html={content}
onChange={(evt) => setContent(evt.target.value)}
onPaste={handlePaste}
/>
);
};
This example demonstrates how to intercept the paste event, extract plain text from the clipboard, and insert it into the editable area using the execCommand
method.
Wrapping Up
React-Contenteditable offers a powerful and flexible solution for creating editable content areas in React applications. From basic text editing to advanced HTML manipulation, this library provides developers with the tools they need to create rich, interactive user experiences.
By leveraging the features and techniques discussed in this guide, you can enhance your React applications with dynamic, user-editable content areas that go beyond the limitations of traditional form inputs. Whether you’re building a simple text editor or a complex content management system, React-Contenteditable provides the foundation for creating engaging, interactive interfaces that your users will love.
As you continue to explore the capabilities of this library, remember to always prioritize user experience and security. With proper implementation and careful consideration of edge cases, React-Contenteditable can become an invaluable tool in your React development toolkit.