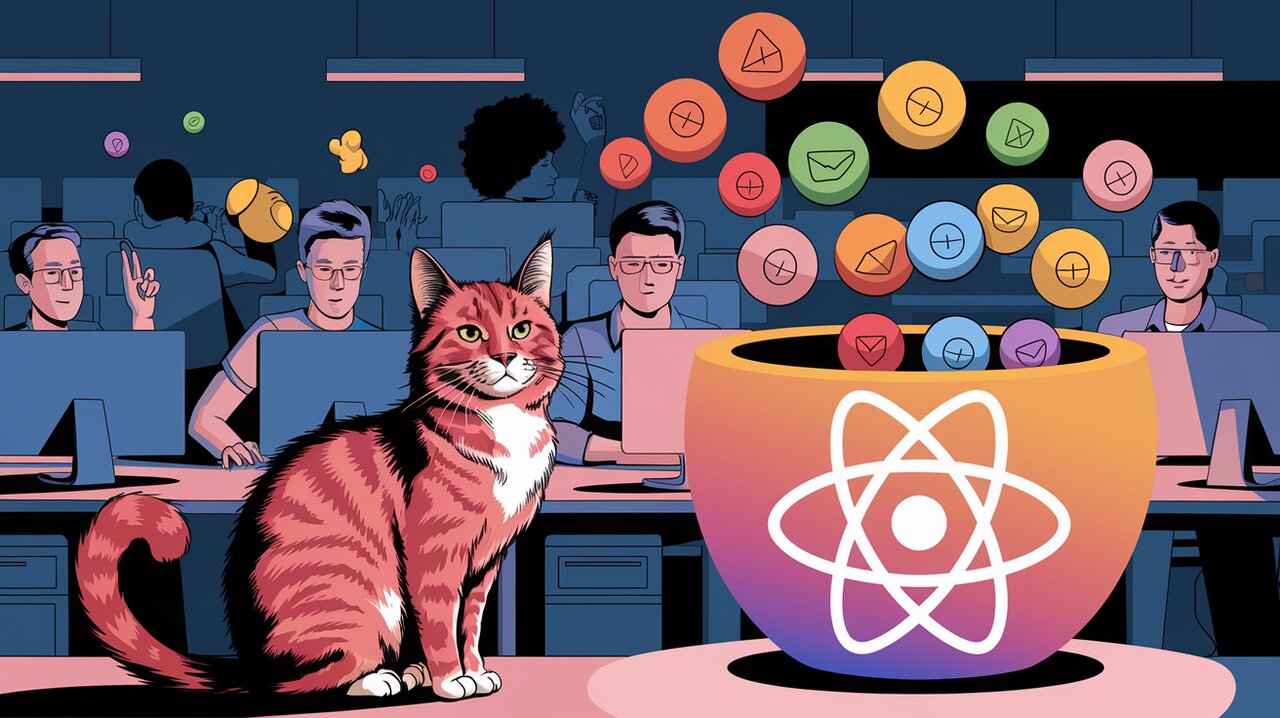
Email Juggling: Mastering Multiple Inputs with react-multi-email
In the ever-evolving landscape of web development, managing user inputs efficiently is crucial for creating smooth and intuitive interfaces. When it comes to collecting multiple email addresses, the process can quickly become cumbersome without the right tools. This is where react-multi-email steps in, offering a elegant solution for React developers looking to streamline their email input fields.
Unpacking the Email Toolbox
Before we dive into the code, let’s explore what makes react-multi-email a valuable addition to your React project:
- Simplicity at Its Core: With a focus on simplicity, this library provides a straightforward way to handle multiple email inputs.
- Lightweight and Independent: No external dependencies mean a smaller footprint and fewer potential conflicts in your project.
- Customization Freedom: Tailor the component to fit your application’s look and feel with ease.
- TypeScript Support: Enjoy the benefits of type safety with built-in TypeScript definitions.
- Validation On-the-Go: Validate emails as they’re entered, ensuring data integrity from the start.
Setting Up Your Email Juggling Act
To begin your email juggling adventure, you’ll need to install react-multi-email. Open your terminal and run one of the following commands:
npm install react-multi-email
# or if you prefer yarn
yarn add react-multi-email
Once installed, you’re ready to import the component and its styles into your React application.
Basic Tricks: Getting Started with react-multi-email
Let’s start with a simple example to see react-multi-email in action:
import React, { useState } from 'react';
import { ReactMultiEmail, isEmail } from 'react-multi-email';
import 'react-multi-email/dist/style.css';
const EmailInput: React.FC = () => {
const [emails, setEmails] = useState<string[]>([]);
const [focused, setFocused] = useState(false);
return (
<div>
<h3>Enter Email Addresses</h3>
<ReactMultiEmail
placeholder="Type an email and press enter"
emails={emails}
onChange={(newEmails: string[]) => setEmails(newEmails)}
onFocus={() => setFocused(true)}
onBlur={() => setFocused(false)}
autoFocus
/>
<p>Focused: {focused ? 'Yes' : 'No'}</p>
<p>Emails: {emails.join(', ')}</p>
</div>
);
};
export default EmailInput;
In this basic setup, we’re creating a component that allows users to input multiple email addresses. The ReactMultiEmail
component handles the heavy lifting, while we manage the state of the emails and focus using React hooks.
Advanced Maneuvers: Customizing Your Email Input
Now that we’ve got the basics down, let’s explore some more advanced features of react-multi-email:
Custom Email Tags
You can customize how each email tag appears by using the getLabel
prop:
<ReactMultiEmail
// ... other props
getLabel={(email, index, removeEmail) => (
<div key={index} style={{ border: '1px solid #ccc', borderRadius: '25px', padding: '2px 8px', margin: '2px' }}>
{email}
<button onClick={() => removeEmail(index)} style={{ marginLeft: '5px', border: 'none', background: 'none', cursor: 'pointer' }}>
×
</button>
</div>
)}
/>
This custom label gives each email tag a pill-like appearance with a remove button, enhancing the visual feedback for users.
Email Validation
While react-multi-email provides basic email validation, you can implement custom validation logic:
const validateEmail = (email: string) => {
// A more comprehensive regex for email validation
const re = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
return re.test(String(email).toLowerCase());
};
<ReactMultiEmail
// ... other props
validateEmail={validateEmail}
/>
This custom validation function allows for more stringent email format checking.
Limiting the Number of Emails
You might want to limit the number of email addresses a user can input:
const MAX_EMAILS = 5;
<ReactMultiEmail
// ... other props
enable={({ emailCnt }) => emailCnt < MAX_EMAILS}
onDisabled={() => alert('You can only enter up to 5 email addresses.')}
/>
This configuration limits the input to a maximum of 5 email addresses and displays an alert when the limit is reached.
Wrapping Up the Email Circus
react-multi-email brings a touch of magic to the often mundane task of collecting multiple email addresses in React applications. Its simplicity and flexibility make it an excellent choice for developers looking to enhance their forms without the complexity of building a custom solution from scratch.
By leveraging the features we’ve explored, you can create intuitive, user-friendly email input fields that handle validation, customization, and state management with ease. Whether you’re building a contact form, a mailing list signup, or any other feature requiring multiple email inputs, react-multi-email proves to be a valuable addition to your React toolkit.
Remember, the key to a great user experience often lies in the details. With react-multi-email, you’re not just collecting emails; you’re crafting a smooth, responsive interface that users will appreciate. So go ahead, give it a try in your next project, and watch as email input juggling becomes a breeze!
For more React form handling techniques, check out our articles on Autocomplete Wizardry with react-autocomplete-input and Numeric Wizardry with react-numeric-input. These complementary libraries can further enhance your form-building arsenal, creating a comprehensive and user-friendly input experience.