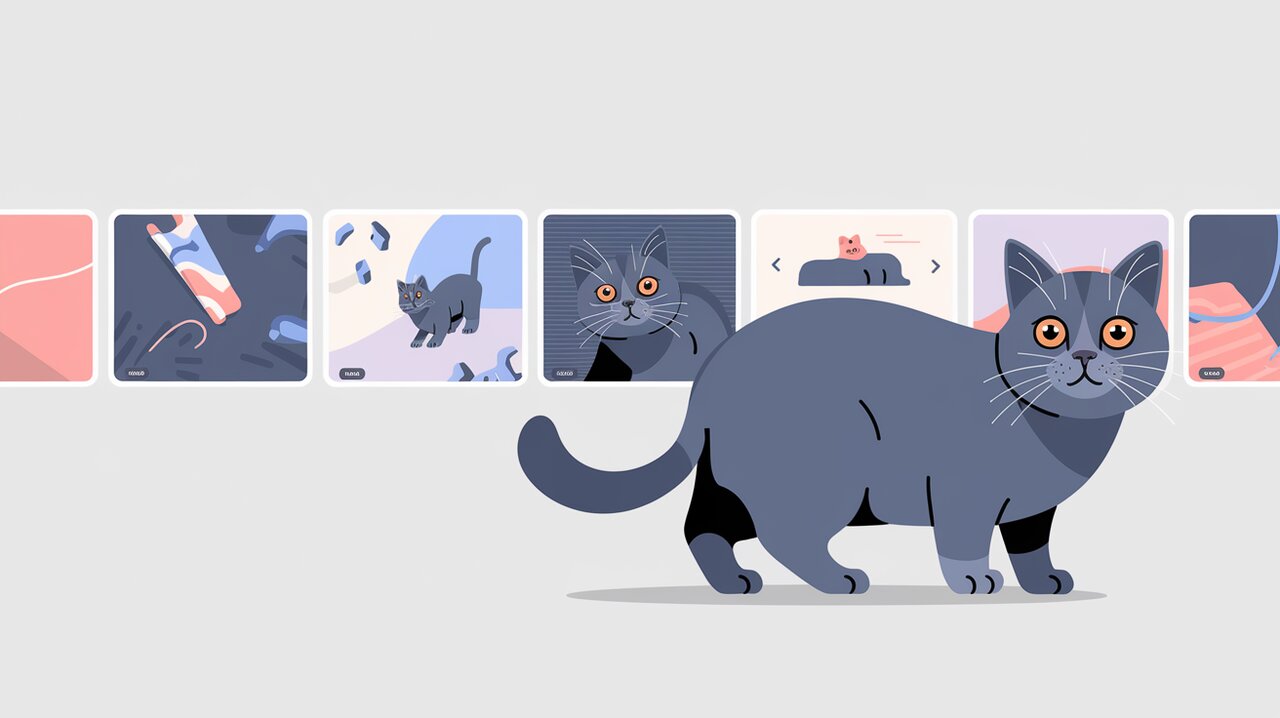
Embla Carousel React: Orchestrating Smooth Sliding Symphonies
Embla Carousel React is a lightweight and flexible carousel library that brings fluid motion and precise swipe interactions to your React applications. With its bare-bones approach and great performance, it’s an excellent choice for developers looking to implement responsive and customizable carousels.
Features
Embla Carousel React comes packed with a range of features that make it stand out:
- Lightweight and dependency-free
- Fluid motion with great swipe precision
- Responsive design
- Customizable options
- Support for infinite looping
- RTL (Right-to-Left) support
- Extensible plugin system
Installation
To get started with Embla Carousel React, you’ll need to install it in your project. You can do this using npm or yarn:
npm install embla-carousel-react
or
yarn add embla-carousel-react
Basic Usage
Let’s dive into a basic example of how to use Embla Carousel React in your project:
import React from 'react'
import useEmblaCarousel from 'embla-carousel-react'
const EmblaCarousel = () => {
const [emblaRef] = useEmblaCarousel()
return (
<div className="embla" ref={emblaRef}>
<div className="embla__container">
<div className="embla__slide">Slide 1</div>
<div className="embla__slide">Slide 2</div>
<div className="embla__slide">Slide 3</div>
</div>
</div>
)
}
export default EmblaCarousel
In this example, we’re using the useEmblaCarousel
hook to create a basic carousel. The emblaRef
is attached to the outer container, while the slides are placed inside the embla__container
div.
Customizing Your Carousel
Embla Carousel React offers a wide range of options to customize your carousel’s behavior. Let’s explore some of these:
import React from 'react'
import useEmblaCarousel from 'embla-carousel-react'
const EmblaCarousel = () => {
const [emblaRef] = useEmblaCarousel({
loop: true,
align: 'center',
slidesToScroll: 2,
dragFree: true
})
return (
<div className="embla" ref={emblaRef}>
{/* Carousel content */}
</div>
)
}
In this example, we’ve added some options to our carousel:
loop: true
enables infinite loopingalign: 'center'
centers the active slideslidesToScroll: 2
sets the number of slides to scroll at a timedragFree: true
allows free-form dragging of the carousel
Advanced Usage: Adding Navigation
Let’s enhance our carousel by adding navigation buttons:
import React, { useCallback } from 'react'
import useEmblaCarousel from 'embla-carousel-react'
const EmblaCarousel = () => {
const [emblaRef, emblaApi] = useEmblaCarousel({ loop: false })
const scrollPrev = useCallback(() => {
if (emblaApi) emblaApi.scrollPrev()
}, [emblaApi])
const scrollNext = useCallback(() => {
if (emblaApi) emblaApi.scrollNext()
}, [emblaApi])
return (
<div className="embla">
<div className="embla__viewport" ref={emblaRef}>
<div className="embla__container">
{/* Slides */}
</div>
</div>
<button className="embla__prev" onClick={scrollPrev}>Prev</button>
<button className="embla__next" onClick={scrollNext}>Next</button>
</div>
)
}
Here, we’re using the emblaApi
returned by useEmblaCarousel
to implement scrollPrev
and scrollNext
functions, which are then attached to our navigation buttons.
Plugins: Extending Functionality
Embla Carousel React supports plugins to extend its functionality. Let’s look at how to use the autoplay plugin:
import React from 'react'
import useEmblaCarousel from 'embla-carousel-react'
import Autoplay from 'embla-carousel-autoplay'
const EmblaCarousel = () => {
const [emblaRef] = useEmblaCarousel({ loop: true }, [Autoplay()])
return (
<div className="embla" ref={emblaRef}>
{/* Carousel content */}
</div>
)
}
In this example, we’ve added the autoplay plugin to our carousel, which will automatically advance the slides.
Conclusion
Embla Carousel React offers a powerful and flexible solution for implementing carousels in your React applications. Its lightweight nature, combined with fluid motion and great swipe precision, makes it an excellent choice for both mobile and desktop interfaces.
By leveraging its customization options and plugin system, you can create carousels that perfectly fit your project’s needs. Whether you’re building a simple image slider or a complex product showcase, Embla Carousel React provides the tools to create smooth, responsive, and user-friendly carousel experiences.
As you continue to explore Embla Carousel React, you might find it helpful to check out related articles on ReactLibs.dev, such as “React Beautiful DnD Exploration” or “React Grid Layout: Mastering Responsive Layouts”. These resources can provide additional insights into creating dynamic and interactive UI components in React.
Remember, the key to mastering Embla Carousel React lies in experimenting with its various options and plugins. So go ahead, start sliding, and create your own carousel symphony!