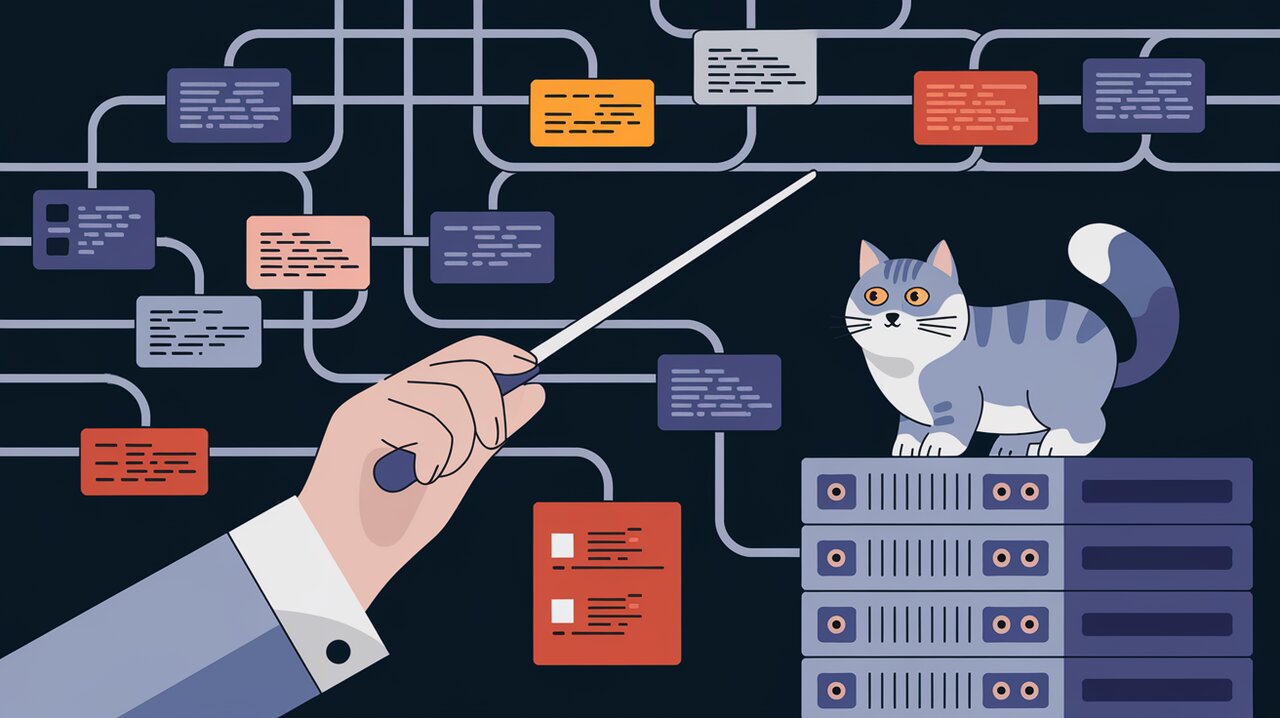
Orchestrating Normalized Data with entities-reducer
In the symphony of React state management, entities-reducer
emerges as a virtuoso conductor, harmonizing the complexities of normalized data structures. This high-order reducer simplifies the process of updating your Redux store with fresh, normalized data, creating a seamless experience for developers working with server-sourced information.
The Overture: Why entities-reducer?
When building React applications that interact with backend services, managing the state of retrieved data can quickly become a complex task. entities-reducer addresses this challenge by providing a streamlined approach to handle normalized data within your Redux store.
The library shines in scenarios where you need to:
- Store and update data from API responses
- Maintain a normalized state structure for efficient data access
- Simplify the process of updating multiple entities at once
Setting the Stage: Installation and Setup
Before we dive into the main performance, let’s set up our environment. You can add entities-reducer
to your project using npm or yarn:
npm install --save entities-reducer
# or
yarn add entities-reducer
Once installed, integrate it into your root reducer:
import { combineReducers } from 'redux';
import entitiesReducer from 'entities-reducer';
const rootReducer = combineReducers({
entities: entitiesReducer({
// Custom reducers can be added here
}),
});
export default rootReducer;
The Main Act: Basic Usage
At its core, entities-reducer
expects actions to contain an entities
property or follow the Flux Standard Action (FSA) specification. Let’s look at a basic example of how it processes an action:
// Action creator
const updateEntities = (data) => ({
type: 'UPDATE_ENTITIES',
payload: {
entities: {
users: {
1: { id: 1, name: 'Alice' },
2: { id: 2, name: 'Bob' }
},
posts: {
101: { id: 101, title: 'Hello World', authorId: 1 }
}
}
}
});
// Dispatch the action
dispatch(updateEntities(data));
In this example, entities-reducer
will automatically update the state with the new user and post entities, merging them with any existing data.
Advanced Techniques: Custom Data Resolution
While entities-reducer
works out of the box for many scenarios, you might encounter situations where the action structure doesn’t quite fit the default expectations. Fear not! The library allows you to pass a custom data resolver to handle these cases.
Crafting a Custom Data Resolver
Here’s an example of a custom data resolver that extracts entities from a nested structure:
const customDataResolver = (action) => {
if (action.error) {
return false; // Skip processing for error actions
}
return action.payload.data.normalizedEntities;
};
const rootReducer = combineReducers({
entities: entitiesReducer({}, { dataResolver: customDataResolver }),
});
This resolver allows entities-reducer
to work with actions that have a different structure, providing flexibility in how you organize your data flow.
Encore: Combining with Custom Reducers
One of the strengths of entities-reducer
is its ability to work alongside custom reducers. This allows you to handle specialized state updates while still benefiting from automatic entity normalization.
const customUserReducer = (state = {}, action) => {
switch (action.type) {
case 'SET_USER_PREFERENCE':
return {
...state,
[action.payload.userId]: {
...state[action.payload.userId],
preferences: action.payload.preferences
}
};
default:
return state;
}
};
const rootReducer = combineReducers({
entities: entitiesReducer({
users: customUserReducer
}),
});
In this setup, entities-reducer
will first process any entity updates, and then the custom reducer will handle specific actions like setting user preferences.
Finale: Embracing the Power of Normalization
By leveraging entities-reducer
, you’re not just simplifying your state management; you’re adopting a best practice in data handling. Normalized state structures offer numerous benefits:
- Reduced data duplication
- Easier state updates and merges
- Improved performance for large datasets
- Simplified data relationships
As you continue to explore the capabilities of entities-reducer
, you’ll find that it harmonizes beautifully with other Redux ecosystem libraries. For instance, you might want to explore how it can be used alongside redux-observable
for handling asynchronous actions, as discussed in our article on Redux Observable Cosmic Symphony.
Additionally, for those looking to further optimize their Redux applications, our guide on Redux Batched Updates Performance Boost offers insights that complement the efficiency gains provided by entities-reducer
.
In conclusion, entities-reducer
offers a powerful yet elegant solution to managing normalized data in React applications. By abstracting away the complexities of state updates, it allows developers to focus on crafting rich, data-driven user experiences. So why not give it a try and let entities-reducer
conduct the symphony of your application’s state?