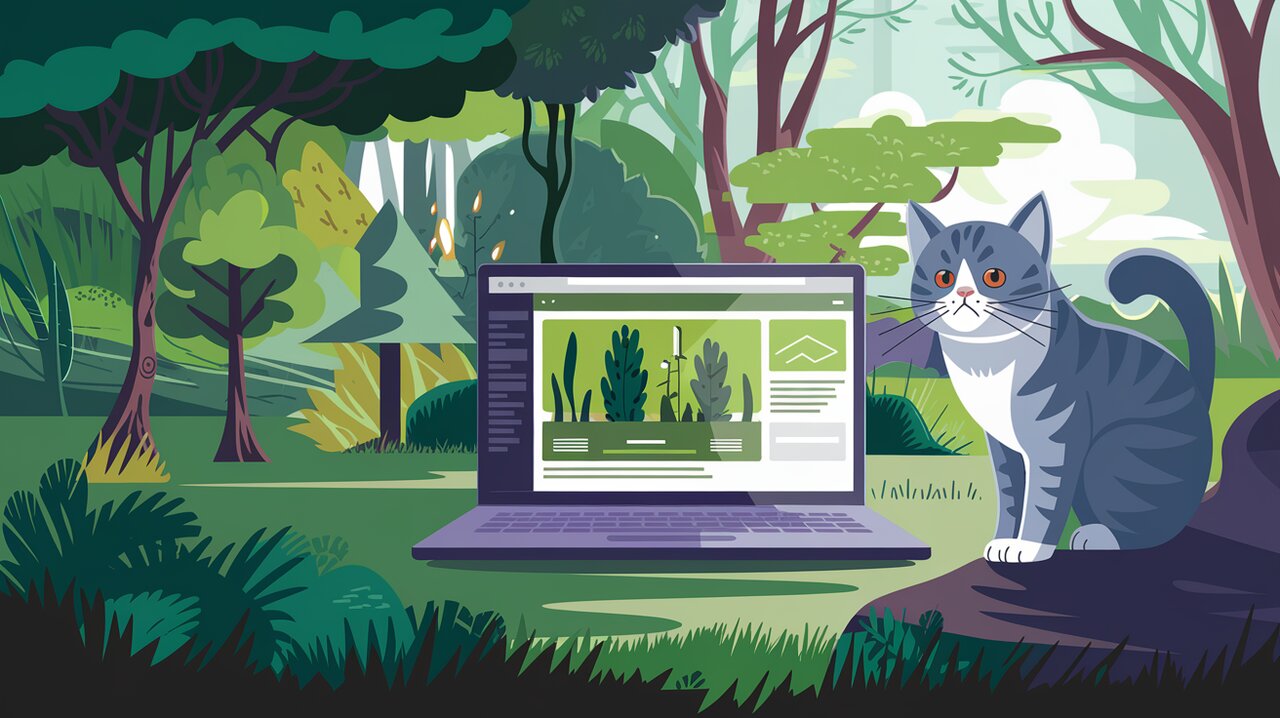
Evergreen UI: Cultivating a Forest of React Components
Evergreen UI is a comprehensive React component library that provides developers with a robust set of tools for building enterprise-grade web applications. Developed by Segment, this UI framework offers a collection of polished, flexible, and composable components that work seamlessly out of the box. Let’s explore the key features and usage of Evergreen UI to understand how it can elevate your React development experience.
Key Features
Evergreen UI stands out with its thoughtfully designed features:
- Out-of-the-box functionality: Evergreen components are ready to use with minimal configuration, saving development time and effort.
- Flexibility and composability: Built on top of React UI primitives, Evergreen components can be easily combined and customized to suit your needs.
- Enterprise-grade design: The library incorporates a UI design language specifically tailored for enterprise web applications.
- Theming support: Evergreen offers a robust theming layer, allowing for easy customization of the visual appearance.
- TypeScript support: The library is fully typed, providing excellent developer experience and type safety.
Getting Started
To begin using Evergreen UI in your React project, you’ll first need to install the package:
npm install evergreen-ui
# or
yarn add evergreen-ui
Once installed, you can start importing and using Evergreen components in your React application:
import React from 'react'
import { Button } from 'evergreen-ui'
const MyComponent = () => {
return (
<Button appearance="primary">
Click me!
</Button>
)
}
export default MyComponent
Basic Usage
Evergreen UI provides a wide array of components to cover various UI needs. Let’s explore some of the commonly used components and their basic usage.
Buttons
Evergreen offers different button styles to suit various contexts:
import React from 'react'
import { Button } from 'evergreen-ui'
const ButtonExample = () => {
return (
<>
<Button appearance="default">Default Button</Button>
<Button appearance="primary">Primary Button</Button>
<Button appearance="minimal">Minimal Button</Button>
<Button intent="success">Success Button</Button>
<Button intent="danger">Danger Button</Button>
</>
)
}
Text Inputs
For capturing user input, Evergreen provides customizable text input components:
import React from 'react'
import { TextInput } from 'evergreen-ui'
const TextInputExample = () => {
return (
<TextInput
name="username"
placeholder="Enter your username"
width={300}
/>
)
}
Dialogs
Evergreen’s dialog component allows you to create modal windows for important information or actions:
import React, { useState } from 'react'
import { Button, Dialog } from 'evergreen-ui'
const DialogExample = () => {
const [isShown, setIsShown] = useState(false)
return (
<>
<Button onClick={() => setIsShown(true)}>Open Dialog</Button>
<Dialog
isShown={isShown}
title="Dialog Title"
onCloseComplete={() => setIsShown(false)}
confirmLabel="Confirm"
>
Dialog content goes here
</Dialog>
</>
)
}
Advanced Usage
Evergreen UI’s power lies in its flexibility and composability. Let’s explore some advanced usage scenarios that showcase these qualities.
Theming
Evergreen provides a theming system that allows you to customize the appearance of components across your application:
import React from 'react'
import { ThemeProvider, defaultTheme, Button } from 'evergreen-ui'
const customTheme = {
...defaultTheme,
colors: {
...defaultTheme.colors,
button: {
...defaultTheme.colors.button,
primary: {
base: '#007bff',
hover: '#0056b3'
}
}
}
}
const ThemedApp = () => {
return (
<ThemeProvider value={customTheme}>
<Button appearance="primary">Themed Button</Button>
</ThemeProvider>
)
}
Composing Components
Evergreen components can be easily composed to create more complex UI elements:
import React from 'react'
import { Pane, Card, Heading, Paragraph, Button } from 'evergreen-ui'
const ComposedCard = () => {
return (
<Card elevation={2} padding={16} width={300}>
<Pane>
<Heading size={700} marginBottom={8}>
Card Title
</Heading>
<Paragraph marginBottom={16}>
This is a composed card using multiple Evergreen components.
</Paragraph>
<Button appearance="primary">Learn More</Button>
</Pane>
</Card>
)
}
Form Validation
Evergreen UI can be integrated with form libraries like Formik for advanced form handling and validation:
import React from 'react'
import { Formik, Form, Field } from 'formik'
import { TextInputField, Button } from 'evergreen-ui'
const FormExample = () => {
return (
<Formik
initialValues={{ email: '', password: '' }}
onSubmit={(values) => console.log(values)}
>
{({ errors, touched }) => (
<Form>
<Field
name="email"
component={TextInputField}
label="Email"
isInvalid={touched.email && errors.email}
validationMessage={touched.email && errors.email}
/>
<Field
name="password"
component={TextInputField}
label="Password"
type="password"
isInvalid={touched.password && errors.password}
validationMessage={touched.password && errors.password}
/>
<Button type="submit" appearance="primary">
Submit
</Button>
</Form>
)}
</Formik>
)
}
Conclusion
Evergreen UI offers a comprehensive solution for building enterprise-grade React applications with its extensive set of polished and flexible components. Its out-of-the-box functionality, combined with powerful theming and composition capabilities, makes it an excellent choice for developers looking to create sophisticated user interfaces efficiently.
By leveraging Evergreen UI, you can significantly reduce development time and ensure consistency across your application. Whether you’re building a simple prototype or a complex enterprise system, Evergreen UI provides the tools you need to create beautiful, functional, and scalable user interfaces.
As you continue your journey with React development, you might also be interested in exploring other UI libraries and tools. Check out our articles on Chakra UI: React Symphony for another powerful component library, or Mastering React Icons Library to enhance your UI with a wide variety of icons. Happy coding!