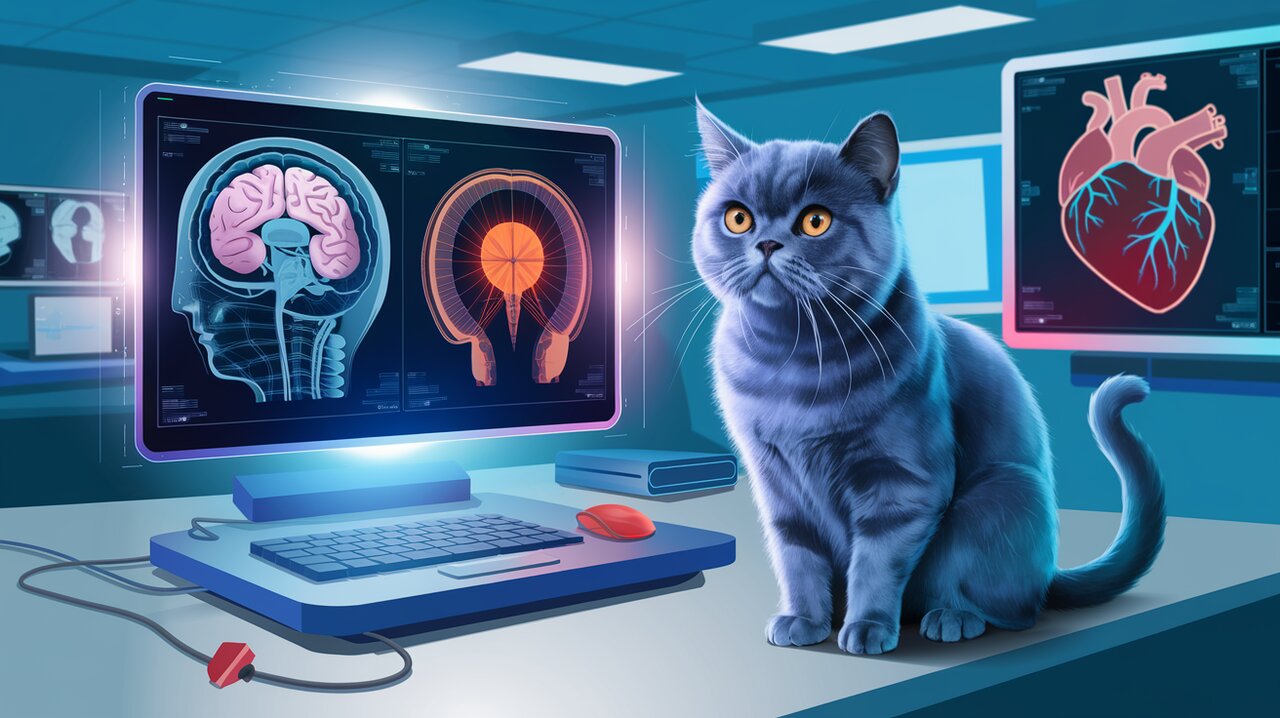
Exploring dwv-react: Bringing Medical Imaging to React Applications
dwv-react is an innovative library that brings the power of medical image viewing to React applications. Built on top of the DWV (DICOM Web Viewer), it allows developers to integrate DICOM image manipulation and visualization directly into web applications. This library is particularly useful for healthcare applications where viewing and interacting with medical images like MRIs, CT scans, and ultrasounds is essential.
Unveiling dwv-react’s Features
The dwv-react library offers several key features that make it a valuable tool for developers working with medical imaging:
- Cross-platform Compatibility: Utilizes JavaScript and HTML5, ensuring it runs smoothly on any modern browser across devices like laptops, tablets, and smartphones.
- DICOM Support: Capable of loading both local and remote DICOM data, the standard format for medical imaging.
- Image Manipulation Tools: Provides essential tools such as contrast adjustment, zooming, and dragging for enhanced image analysis.
- Non-DICOM Formats: Supports additional image formats like JPEG and PNG for broader compatibility.
Getting Started with Installation
To integrate dwv-react
into your project, you can use either npm or yarn as your package manager:
# Using npm
npm install dwv-react
# Using yarn
yarn add dwv-react
Basic Usage in React Applications
Setting Up Your First Viewer
To start using dwv-react
, you need to set up a basic viewer component in your React application. Here’s a simple example:
import React from 'react';
import { Viewer } from 'dwv-react';
const MyImageViewer = () => {
return (
<div>
<h1>My Medical Image Viewer</h1>
<Viewer />
</div>
);
};
export default MyImageViewer;
In this example, we import the Viewer
component from dwv-react
and embed it within our custom component. This setup provides a basic interface to load and interact with DICOM images.
Loading DICOM Data
To load DICOM files into your viewer, you can use the following approach:
import React from 'react';
import { Viewer } from 'dwv-react';
const MyImageViewer = () => {
const handleFileChange = (event: React.ChangeEvent<HTMLInputElement>) => {
const files = event.target.files;
if (files && files.length > 0) {
const file = files[0];
// Logic to handle file loading goes here
}
};
return (
<div>
<h1>My Medical Image Viewer</h1>
<input type="file" onChange={handleFileChange} />
<Viewer />
</div>
);
};
export default MyImageViewer;
This code snippet demonstrates how to add a file input to your viewer component. Users can select a DICOM file to load into the viewer for display.
Diving Deeper: Advanced Usage
Customizing Image Tools
You might want to customize the tools available in your viewer. Here’s how you can configure tool options:
import React from 'react';
import { Viewer } from 'dwv-react';
const MyCustomViewer = () => {
const toolOptions = {
tools: ['Zoom', 'Pan', 'WindowLevel'], // Specify which tools to include
};
return (
<div>
<h1>Custom Medical Image Viewer</h1>
<Viewer options={toolOptions} />
</div>
);
};
export default MyCustomViewer;
This example shows how to customize the tools available in the viewer by specifying them in the options object.
Integrating with External Data Sources
For more advanced applications, you may need to load images from external sources or APIs:
import React, { useEffect } from 'react';
import { Viewer } from 'dwv-react';
const ExternalDataViewer = () => {
useEffect(() => {
// Fetch data from an API or external source here
// Example API call logic goes here
}, []);
return (
<div>
<h1>External Data Image Viewer</h1>
<Viewer />
</div>
);
};
export default ExternalDataViewer;
In this setup, we use useEffect
to fetch data when the component mounts, allowing you to integrate seamlessly with external data sources.
Wrapping Up
The dwv-react library is an excellent choice for developers looking to incorporate sophisticated medical image viewing capabilities into their React applications. With its robust set of features and ease of integration, it empowers developers to create powerful healthcare solutions that enhance diagnostic processes and improve patient care. Whether you’re building a simple viewer or a complex application with custom tools and data integrations, dwv-react provides the flexibility and functionality needed for modern web-based medical imaging.