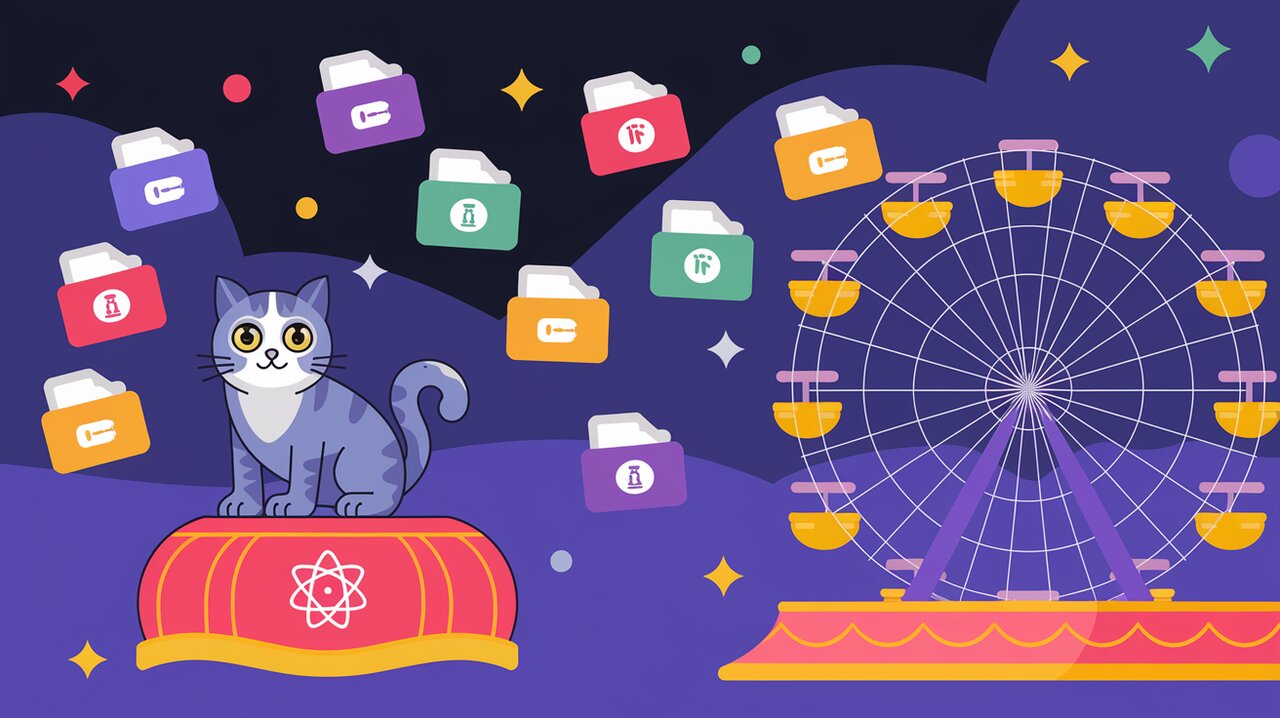
File Upload Fiesta: Simplifying React Input with react-simple-file-input
React developers, get ready to revolutionize your file upload experience! The react-simple-file-input
library is here to turn the often tedious task of handling file inputs into a delightful fiesta of functionality. Let’s dive into this powerful tool that wraps the HTML input tag and HTML5 FileReader API with React goodness.
Unwrapping the Gift: Features and Installation
react-simple-file-input comes packed with features that make file handling a breeze:
- Simple integration with React components
- Support for multiple file selection
- Customizable file reading methods
- Extensive event handling capabilities
- Cancellation and abortion of file reads
To add this gem to your project, simply run:
npm install react-simple-file-input --save
For yarn enthusiasts:
yarn add react-simple-file-input
The Grand Entrance: Basic Usage
Let’s start the party with a basic example of how to use react-simple-file-input
:
import React from 'react';
import FileInput from 'react-simple-file-input';
const FileUploadComponent: React.FC = () => {
const handleFileSelected = (event: ProgressEvent<FileReader>, file: File) => {
console.log('File content:', event.target.result);
console.log('File details:', file);
};
return (
<FileInput
readAs="text"
onLoad={handleFileSelected}
/>
);
};
export default FileUploadComponent;
In this example, we’re creating a simple file input that reads the file as text and logs its content and details when loaded. The readAs
prop specifies how the file should be read, and the onLoad
prop defines what happens after the file is read.
The Carnival of Props: Customizing Your Input
react-simple-file-input offers a variety of props to tailor the file input to your needs:
Reading Files
The readAs
prop is your ticket to specifying how files should be read:
'text'
for plain text'buffer'
for array buffers'binary'
for binary strings'dataUrl'
for data URLs
If readAs
is undefined, the file won’t be read from disk, and only the onChange
event will fire.
Event Extravaganza
The library supports a wide range of events to give you full control over the file upload process:
import React from 'react';
import FileInput from 'react-simple-file-input';
const AdvancedFileUpload: React.FC = () => {
return (
<FileInput
readAs="binary"
onLoadStart={(e, file) => console.log('Started loading:', file.name)}
onProgress={(e, file) => console.log(`Progress: ${(e.loaded / e.total) * 100}%`)}
onLoad={(e, file) => console.log('File loaded:', file.name)}
onLoadEnd={(e, file) => console.log('Finished loading:', file.name)}
onAbort={(e, file) => console.log('File read aborted:', file.name)}
onError={(e, file) => console.error('Error reading file:', file.name)}
onChange={(file) => console.log('File selected:', file.name)}
/>
);
};
export default AdvancedFileUpload;
This carnival of events allows you to track every step of the file upload process, from selection to completion.
The Multi-File Parade: Handling Multiple Uploads
Want to allow users to upload multiple files? It’s as simple as adding the multiple
prop:
import React from 'react';
import FileInput from 'react-simple-file-input';
const MultiFileUpload: React.FC = () => {
const handleMultipleFiles = (files: FileList) => {
Array.from(files).forEach(file => {
console.log('File selected:', file.name);
});
};
return (
<FileInput
multiple
onChange={handleMultipleFiles}
/>
);
};
export default MultiFileUpload;
With the multiple
prop, users can select multiple files, and the onChange
handler receives a FileList
instead of a single File
object.
The Safety Net: Cancellation and Abortion
Sometimes, you need to stop the show. react-simple-file-input provides props for cancelling and aborting file reads:
import React, { useState } from 'react';
import FileInput from 'react-simple-file-input';
const CancellableFileUpload: React.FC = () => {
const [cancelUpload, setCancelUpload] = useState(false);
const checkFileType = (file: File) => {
const allowedTypes = ['image/jpeg', 'image/png'];
return !allowedTypes.includes(file.type);
};
return (
<>
<FileInput
readAs="dataUrl"
cancelIf={checkFileType}
abortIf={() => cancelUpload}
onCancel={(file) => console.log(`Cancelled upload of ${file.name}: Invalid file type`)}
onAbort={(e, file) => console.log(`Aborted upload of ${file.name}`)}
/>
<button onClick={() => setCancelUpload(true)}>Cancel Upload</button>
</>
);
};
export default CancellableFileUpload;
The cancelIf
prop allows you to prevent file reads based on certain conditions, while abortIf
can stop an ongoing read process.
The Grand Finale: Styling and Integration
While react-simple-file-input doesn’t support children components, you can easily style and customize the input using standard HTML attributes:
import React from 'react';
import FileInput from 'react-simple-file-input';
const StyledFileUpload: React.FC = () => {
return (
<label className="custom-file-upload">
<FileInput
className="file-input"
style={{ display: 'none' }}
onChange={(file) => console.log('File selected:', file.name)}
/>
<span>Choose a file</span>
</label>
);
};
export default StyledFileUpload;
This approach allows you to create a custom-styled button that triggers the file input when clicked.
Conclusion: The After-Party
react-simple-file-input transforms the mundane task of file uploading into an exciting and manageable process. Its simplicity in basic usage, combined with the power of advanced features, makes it a valuable addition to any React developer’s toolkit.
Whether you’re building a simple upload form or a complex file management system, this library provides the flexibility and control you need. So why not give your next project a file upload fiesta with react-simple-file-input
?
For more React component magic, check out our articles on react-dropzone for drag-and-drop file uploads, and react-filepond for a full-featured file upload component. Happy uploading, React developers!