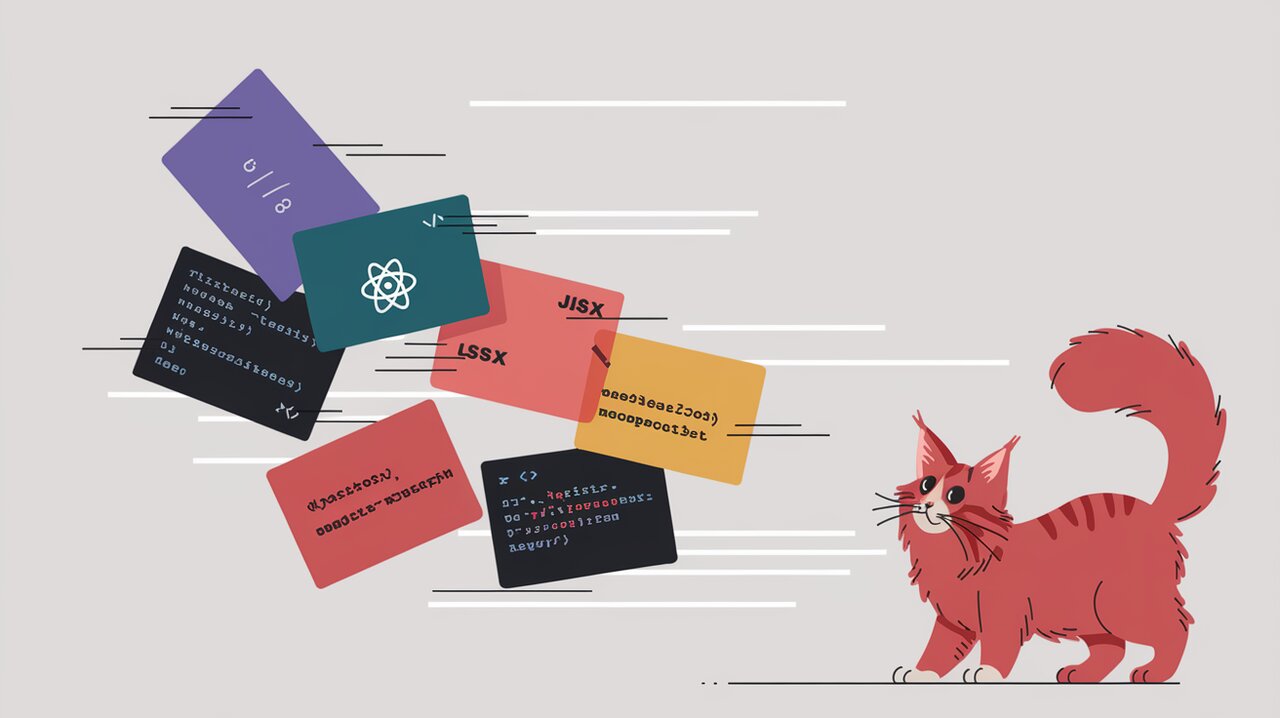
Flipping Awesome: Mastering List Animations with React Flip Move
React Flip Move is a powerful library designed to tackle the common yet challenging task of animating list reordering in React applications. By leveraging the FLIP (First, Last, Invert, Play) technique, it provides smooth, hardware-accelerated animations without the complexity of manual DOM manipulation. This library is particularly useful for developers looking to enhance their user interfaces with fluid transitions when list items are added, removed, or reordered.
Features
React Flip Move offers several key features that make it stand out:
- Effortless Integration: Easily wrap your list items with the FlipMove component for instant animations.
- High Performance: Utilizes 60+ FPS hardware-accelerated CSS transforms for smooth animations.
- Customizable: Supports custom enter/leave animations and easing functions.
- Lightweight: Minimal impact on your bundle size, ensuring fast load times.
Installation
To get started with React Flip Move, you can install it using npm or yarn:
npm install react-flip-move
# or with yarn
yarn add react-flip-move
For those not using a package manager, a UMD build is also available:
<script src="https://unpkg.com/react-flip-move/dist/react-flip-move.js"></script>
Basic Usage
Let’s dive into how you can use React Flip Move in your project. Here’s a simple example of a list component that animates when items are reordered:
Creating an Animated List
import React, { useState } from 'react';
import FlipMove from 'react-flip-move';
interface ListItem {
id: number;
text: string;
}
const AnimatedList: React.FC = () => {
const [items, setItems] = useState<ListItem[]>([
{ id: 1, text: 'Item 1' },
{ id: 2, text: 'Item 2' },
{ id: 3, text: 'Item 3' },
]);
const shuffleItems = () => {
setItems([...items].sort(() => Math.random() - 0.5));
};
return (
<div>
<button onClick={shuffleItems}>Shuffle Items</button>
<FlipMove>
{items.map(item => (
<div key={item.id}>{item.text}</div>
))}
</FlipMove>
</div>
);
};
In this example, we create a simple list of items and a button to shuffle them. The FlipMove
component wraps our list items, automatically handling the animations when the order changes. Each item must have a unique key
prop for React Flip Move to track and animate it correctly.
Customizing Enter and Leave Animations
React Flip Move allows you to customize how elements enter and leave the list:
<FlipMove
enterAnimation="fade"
leaveAnimation="accordionVertical"
>
{/* List items */}
</FlipMove>
This configuration applies a fade effect for entering elements and a vertical accordion effect for leaving elements.
Advanced Usage
React Flip Move offers more advanced features for fine-tuning your animations. Let’s explore some of these capabilities.
Staggered Animations
You can create a staggered effect where items animate one after another:
<FlipMove staggerDurationBy={30} staggerDelayBy={20}>
{/* List items */}
</FlipMove>
This creates a cascading animation effect, with each item’s animation starting slightly after the previous one.
Custom Animation Styles
For more control over the animation, you can provide custom styles:
const customAnimation = {
from: { transform: 'translateY(50px)', opacity: 0 },
to: { transform: 'translateY(0)', opacity: 1 }
};
<FlipMove enterAnimation={customAnimation} leaveAnimation={customAnimation}>
{/* List items */}
</FlipMove>
This allows you to define precise start and end states for your animations.
Handling Stateless Functional Components
React Flip Move requires access to the underlying DOM nodes, which can be problematic with stateless functional components. To work around this, you can use React.forwardRef
:
const ListItem = React.forwardRef<HTMLDivElement, { text: string }>(
({ text }, ref) => (
<div ref={ref}>{text}</div>
)
);
// Usage
<FlipMove>
{items.map(item => (
<ListItem key={item.id} text={item.text} />
))}
</FlipMove>
This ensures that React Flip Move can properly access and animate the DOM nodes of your functional components.
Conclusion
React Flip Move provides a powerful yet easy-to-use solution for creating smooth list animations in React applications. By abstracting away the complexities of DOM manipulation and leveraging the FLIP technique, it allows developers to focus on creating great user experiences without getting bogged down in animation details.
Whether you’re building a simple todo list or a complex data visualization, React Flip Move can help you add that extra layer of polish to your UI transitions. Its flexibility, performance, and ease of use make it a valuable tool in any React developer’s toolkit.
As you continue to explore React Flip Move, remember to experiment with different animation styles, timings, and configurations to find the perfect fit for your project. Happy flipping!