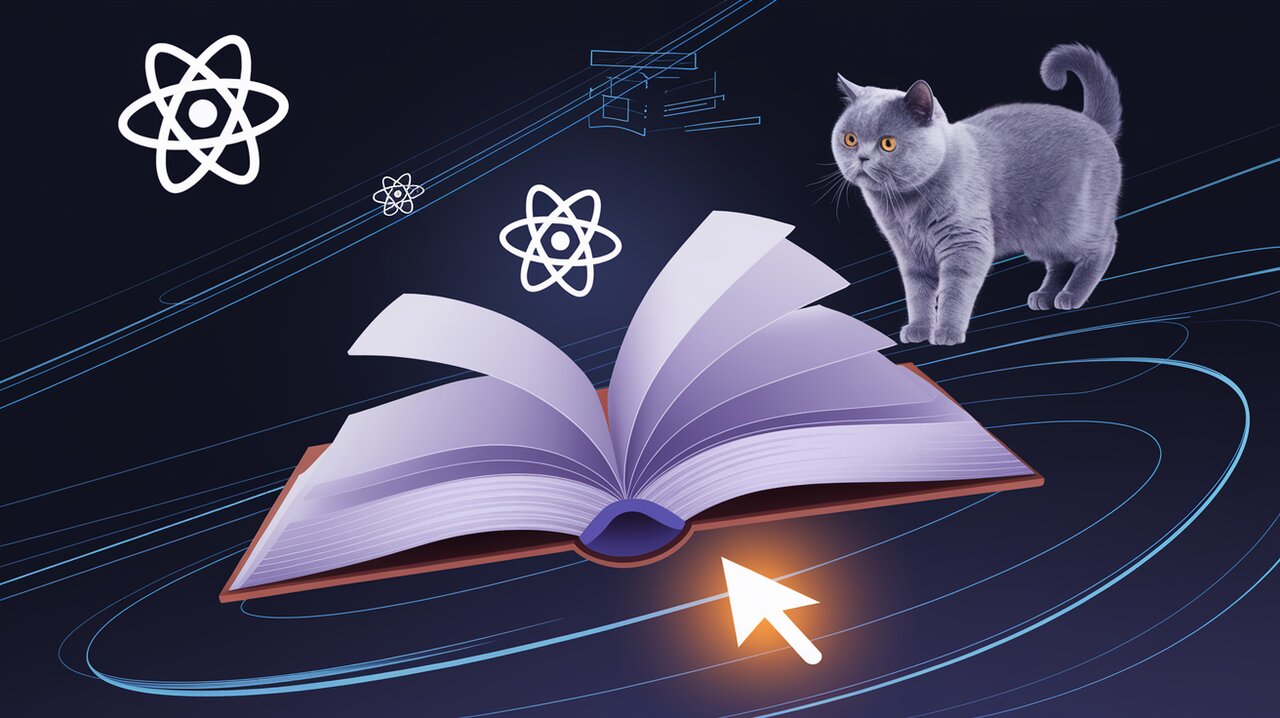
Flipping Fantastic: Mastering React-PageFlip for Dynamic Book Experiences
React developers are constantly seeking ways to enhance user experiences and create more engaging interfaces. Enter react-pageflip
, a library that brings the tactile joy of turning pages to the digital realm. This powerful tool allows you to create realistic book-like interfaces in your React applications, complete with smooth animations and interactive features. Let’s dive into the world of react-pageflip
and explore how it can add a new dimension to your projects.
Turning the First Page: Getting Started with react-pageflip
Before we delve into the intricacies of page-flipping magic, let’s set the stage by installing the library. Open your terminal and run:
npm install react-pageflip
Or if you prefer yarn:
yarn add react-pageflip
With the library now at your fingertips, it’s time to create your first flippable book component.
Basic Usage: Crafting Your First Book
Let’s start with a simple example to get a feel for how react-pageflip
works:
import React from 'react';
import HTMLFlipBook from 'react-pageflip';
function MyBook(props) {
return (
<HTMLFlipBook width={300} height={500}>
<div className="demoPage">Page 1</div>
<div className="demoPage">Page 2</div>
<div className="demoPage">Page 3</div>
<div className="demoPage">Page 4</div>
</HTMLFlipBook>
);
}
In this basic setup, we’ve created a book with four pages, each represented by a div
element. The HTMLFlipBook
component wraps these pages, creating a flippable interface.
Advanced Techniques: Customizing Your Book
For more control over your pages, you can define them as separate components. Here’s how you can create custom page components:
const Page = React.forwardRef((props, ref) => {
return (
<div className="demoPage" ref={ref}>
<h1>Page Header</h1>
<p>{props.children}</p>
<p>Page number: {props.number}</p>
</div>
);
});
function MyBook(props) {
return (
<HTMLFlipBook width={300} height={500}>
<Page number="1">Page text</Page>
<Page number="2">Page text</Page>
<Page number="3">Page text</Page>
<Page number="4">Page text</Page>
</HTMLFlipBook>
);
}
This approach allows for more complex page structures and dynamic content.
Configuring Your Book: Props and Options
react-pageflip
offers a variety of props to customize the behavior and appearance of your book:
width
andheight
: Set the dimensions of your book (required).size
: Choose between “fixed” or “stretch” to determine how the book fits its container.minWidth
,maxWidth
,minHeight
,maxHeight
: Set size thresholds when using “stretch” mode.drawShadow
: Enable or disable page-turning shadows.flippingTime
: Adjust the duration of the flipping animation.usePortrait
: Allow switching to portrait mode.startZIndex
: Set the initial z-index value.autoSize
: Automatically adjust the parent element to the book’s size.maxShadowOpacity
: Control the intensity of shadows.showCover
: Display the first and last pages as hard covers in single page mode.mobileScrollSupport
: Enable or disable content scrolling on mobile devices.clickEventForward
: Forward click events to child elements.
Interactivity: Handling Events
react-pageflip
provides several events that you can listen to and respond to:
function DemoBook() {
const onFlip = React.useCallback((e) => {
console.log('Current page: ' + e.data);
}, []);
return (
<HTMLFlipBook
width={300}
height={500}
onFlip={onFlip}
>
{/* ... pages ... */}
</HTMLFlipBook>
);
}
Available events include:
onFlip
: Triggered when a page is turned.onChangeOrientation
: Fires when the book orientation changes.onChangeState
: Indicates changes in the book’s state.onInit
: Called when the book is initialized.onUpdate
: Triggered when the book’s pages are updated.
Programmatic Control: Methods for Manipulation
To programmatically control your book, you can use the pageFlip()
method accessed through a ref:
function DemoBook() {
const book = React.useRef();
return (
<>
<button onClick={() => book.current.pageFlip().flipNext()}>
Next page
</button>
<HTMLFlipBook
width={300}
height={500}
ref={book}
>
{/* ... pages ... */}
</HTMLFlipBook>
</>
);
}
Some useful methods include:
getPageCount()
: Get the total number of pages.getCurrentPageIndex()
: Retrieve the current page number.turnToPage(pageNum)
: Jump to a specific page.flipNext()
andflipPrev()
: Turn to the next or previous page with animation.loadFromImages(images)
: Load pages from an array of image URLs.loadFromHtml(items)
: Load pages from HTML elements.
Beyond the Basics: Creative Applications
Now that you’ve mastered the fundamentals of react-pageflip
, consider these creative ways to use it in your projects:
- Interactive Portfolios: Create a portfolio that feels like a physical book, showcasing your work with style.
- Digital Magazines: Build engaging digital publications with realistic page-turning effects.
- Educational Resources: Develop interactive textbooks or learning materials that students can flip through.
- Product Catalogs: Design catalogs that customers can browse as if they were holding a physical brochure.
- Story Books: Craft immersive storytelling experiences for children’s books or visual novels.
Conclusion: Turning a New Page in React Development
react-pageflip
opens up a world of possibilities for creating engaging, book-like interfaces in your React applications. By leveraging its realistic page-turning animations and customizable features, you can elevate your user interfaces and provide a unique, interactive experience for your users.
As you continue to explore the capabilities of react-pageflip
, remember that the key to creating truly engaging experiences lies in the details. Experiment with different configurations, combine it with other React libraries, and let your creativity guide you in turning static content into dynamic, flippable masterpieces.
For more React animation magic, check out our articles on Animate with Framer Motion and Flipping Awesome React Animations. Happy flipping!