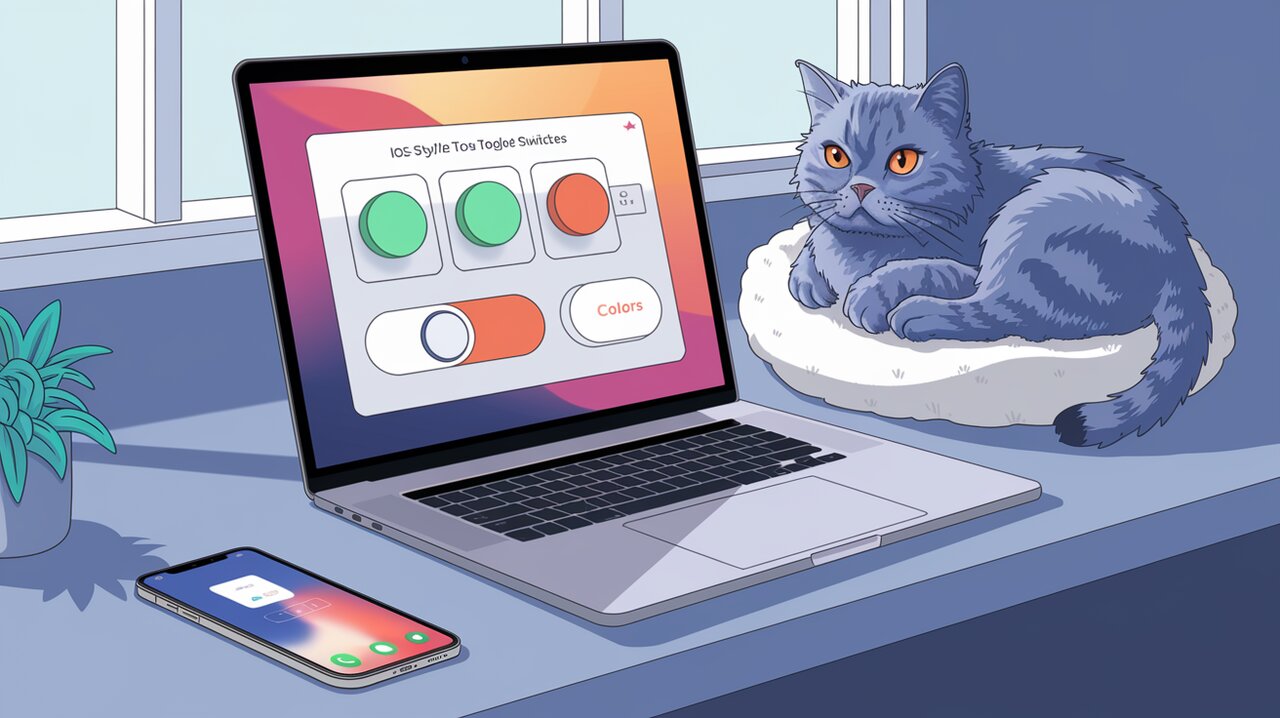
Flipping Switches: Bringing iOS Toggle Magic to React
In the world of user interface design, toggle switches have become an essential element for enabling or disabling features with a simple flick. For React developers looking to bring the sleek and intuitive feel of iOS switches to their web applications, the React iOS Switch library offers an excellent solution. This powerful yet lightweight component allows you to easily implement iOS-style toggle switches in your React projects, enhancing both the functionality and aesthetic appeal of your user interfaces.
Flipping the Switch on Features
React iOS Switch comes packed with a set of features that make it a versatile choice for developers:
- Click or Drag Functionality: Users can toggle the switch by clicking or dragging, providing a natural and interactive experience.
- Label Compatibility: The component works seamlessly with labels, allowing for clear and descriptive toggle options.
- Customizable Appearance: Developers can easily adjust colors and styles to match their application’s design language.
- Responsive Behavior: The switch responds to user interactions with smooth animations, mimicking the iOS native experience.
Installing the Magic
Before we dive into using React iOS Switch, let’s get it installed in your project. You can use either npm or yarn to add the library to your dependencies.
Using npm:
npm install react-ios-switch
Using yarn:
yarn add react-ios-switch
Once installed, you’re ready to start implementing iOS-style switches in your React application.
Basic Usage: Flipping the Switch
Let’s start with a simple implementation of the React iOS Switch component. This basic usage will give you a functional toggle switch with default styling.
Simple Toggle Implementation
import React, { useState } from 'react';
import Switch from 'react-ios-switch';
const ToggleExample: React.FC = () => {
const [isChecked, setIsChecked] = useState(false);
const handleToggle = (checked: boolean) => {
setIsChecked(checked);
};
return (
<Switch
checked={isChecked}
onChange={handleToggle}
/>
);
};
export default ToggleExample;
In this example, we’ve created a functional component that uses the useState
hook to manage the switch’s state. The Switch
component from react-ios-switch
is then rendered with two key props:
checked
: Determines the current state of the switch (on or off).onChange
: A callback function that updates the state when the switch is toggled.
This simple implementation gives you a fully functional iOS-style toggle switch that updates its state when clicked or dragged.
Advanced Customization: Painting Your Switch
While the default styling of React iOS Switch is designed to mimic iOS switches, you might want to customize its appearance to better fit your application’s design. Let’s explore some advanced customization options.
Colorful Toggles
import React, { useState } from 'react';
import Switch from 'react-ios-switch';
const CustomColorSwitch: React.FC = () => {
const [isChecked, setIsChecked] = useState(false);
return (
<Switch
checked={isChecked}
onChange={setIsChecked}
onColor="#4CD964"
offColor="#D1D1D6"
handleColor="#FFFFFF"
/>
);
};
export default CustomColorSwitch;
In this example, we’ve customized the colors of the switch:
onColor
: Sets the background color when the switch is on.offColor
: Defines the background color when the switch is off.handleColor
: Specifies the color of the switch handle.
These color customizations allow you to match the switch’s appearance to your application’s color scheme.
Disabled State Styling
Sometimes, you might need to disable a switch. Here’s how you can style a disabled switch:
import React from 'react';
import Switch from 'react-ios-switch';
const DisabledSwitch: React.FC = () => {
return (
<Switch
checked={true}
disabled={true}
onColor="#A3A3A3"
handleColor="#D4D4D4"
/>
);
};
export default DisabledSwitch;
By setting the disabled
prop to true
, you can create a switch that can’t be interacted with. Adjusting the onColor
and handleColor
for the disabled state helps to visually communicate that the switch is not interactive.
Flipping in Context: Real-World Scenarios
Now that we’ve covered the basics and some advanced customizations, let’s look at how React iOS Switch can be used in real-world scenarios.
Theme Toggler
import React, { useState, useEffect } from 'react';
import Switch from 'react-ios-switch';
const ThemeToggler: React.FC = () => {
const [isDarkMode, setIsDarkMode] = useState(false);
useEffect(() => {
document.body.classList.toggle('dark-mode', isDarkMode);
}, [isDarkMode]);
return (
<div>
<label>
Dark Mode
<Switch
checked={isDarkMode}
onChange={setIsDarkMode}
onColor="#34C759"
offColor="#8E8E93"
/>
</label>
</div>
);
};
export default ThemeToggler;
This example demonstrates how to use React iOS Switch to create a theme toggler. The switch controls the application’s dark mode, applying a CSS class to the body element when toggled on.
Feature Flag Control
import React, { useState } from 'react';
import Switch from 'react-ios-switch';
interface FeatureFlag {
name: string;
enabled: boolean;
}
const FeatureFlagControl: React.FC = () => {
const [features, setFeatures] = useState<FeatureFlag[]>([
{ name: 'New Dashboard', enabled: false },
{ name: 'Advanced Analytics', enabled: true },
{ name: 'Beta Features', enabled: false },
]);
const toggleFeature = (index: number) => {
setFeatures(prevFeatures =>
prevFeatures.map((feature, i) =>
i === index ? { ...feature, enabled: !feature.enabled } : feature
)
);
};
return (
<div>
<h3>Feature Flags</h3>
{features.map((feature, index) => (
<div key={feature.name}>
<label>
{feature.name}
<Switch
checked={feature.enabled}
onChange={() => toggleFeature(index)}
onColor="#007AFF"
offColor="#E5E5EA"
/>
</label>
</div>
))}
</div>
);
};
export default FeatureFlagControl;
This more complex example shows how React iOS Switch can be used to manage feature flags in an application. Each feature is represented by a switch, allowing easy toggling of different application features.
Wrapping Up: The Power of the Flip
React iOS Switch brings the familiar and user-friendly iOS toggle switch experience to React applications. Its simplicity in basic usage, combined with the flexibility for advanced customization, makes it an excellent choice for developers looking to enhance their user interfaces with intuitive toggle controls.
From simple on/off switches to complex feature management systems, this library provides the tools you need to create responsive and visually appealing toggle interfaces. By leveraging the power of React iOS Switch, you can significantly improve the user experience of your React applications, making interactions more intuitive and enjoyable for your users.
Whether you’re building a theme switcher, managing feature flags, or simply need a sleek toggle for your settings page, React iOS Switch offers a robust solution that combines functionality with style. So go ahead, flip the switch, and watch your React applications come to life with iOS-inspired toggle magic!