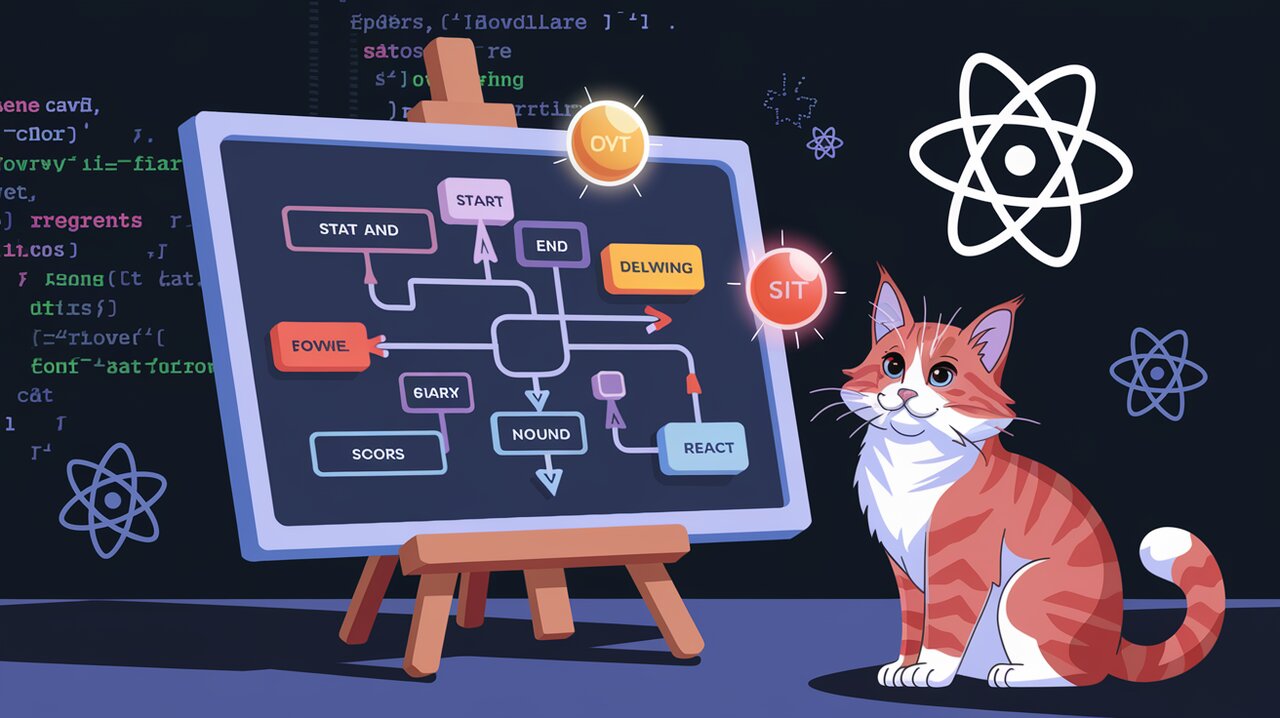
Flowchart React: Weaving Visual Magic into Your React Apps
Flowchart React is a lightweight yet powerful library that brings the art of flowchart creation to your React applications. With its intuitive API and flexible design, this tool empowers developers to craft interactive, visually appealing diagrams that can represent complex processes, decision trees, or any structured flow of information.
Painting with Nodes and Connections
At its core, Flowchart React uses two main building blocks: nodes and connections. Nodes represent individual steps or elements in your flowchart, while connections illustrate the relationships and flow between these nodes. This simple yet effective approach allows for the creation of diverse and intricate flowcharts.
Key Features
- Interactive Design: Easily drag, connect, and modify nodes in real-time.
- Customizable Appearance: Tailor the look of nodes and connections to match your application’s style.
- Responsive Layout: Flowcharts adapt seamlessly to different screen sizes.
- Event Handling: Respond to user interactions with built-in event listeners.
- Toolbar Integration: Quick access to common node types for rapid flowchart construction.
Setting Up Your Canvas
To begin your journey with Flowchart React, you’ll first need to add it to your project. Let’s get started with the installation process.
Installation
You can install Flowchart React using either npm or yarn:
npm install --save flowchart-react
Or if you prefer yarn:
yarn add flowchart-react
Sketching Your First Flowchart
Now that we have Flowchart React installed, let’s create a basic flowchart to showcase its capabilities.
Basic Usage
Here’s a simple example to get you started:
import React, { useState } from "react";
import Flowchart from "flowchart-react";
import { ConnectionData, NodeData } from "flowchart-react/schema";
const SimpleFlowchart: React.FC = () => {
const [nodes, setNodes] = useState<NodeData[]>([
{ id: 1, type: "start", title: "Begin", x: 100, y: 100 },
{ id: 2, type: "operation", title: "Process", x: 100, y: 200 },
{ id: 3, type: "end", title: "Finish", x: 100, y: 300 }
]);
const [connections, setConnections] = useState<ConnectionData[]>([
{ source: { id: 1, position: "bottom" }, destination: { id: 2, position: "top" } },
{ source: { id: 2, position: "bottom" }, destination: { id: 3, position: "top" } }
]);
return (
<Flowchart
nodes={nodes}
connections={connections}
onChange={(newNodes, newConnections) => {
setNodes(newNodes);
setConnections(newConnections);
}}
style={{ width: 600, height: 400 }}
/>
);
};
In this example, we’ve created a simple linear flowchart with three nodes: a start node, an operation node, and an end node. The onChange
prop allows us to update the state of our nodes and connections as the user interacts with the flowchart.
Customizing Node Appearance
Flowchart React allows you to customize the appearance of your nodes. Let’s explore how to add some flair to our flowchart:
const CustomNode: React.FC = () => {
const [nodes, setNodes] = useState<NodeData[]>([
{
id: 1,
type: "operation",
title: "Custom Node",
x: 100,
y: 100,
containerProps: { fill: "#f0f0f0", stroke: "#333" },
textProps: { fill: "#333" }
}
]);
// ... rest of the component
};
By using the containerProps
and textProps
, we can easily adjust the colors and styles of our nodes to match our application’s design.
Advanced Flowchart Techniques
Now that we’ve covered the basics, let’s dive into some more advanced features of Flowchart React.
Dynamic Node Titles
You can make your flowcharts more dynamic by using functions for node titles:
const DynamicTitleNode: React.FC = () => {
const [nodes, setNodes] = useState<NodeData[]>([
{
id: 1,
type: "operation",
title: (node) => `Node ${node.id}: ${new Date().toLocaleTimeString()}`,
x: 100,
y: 100
}
]);
// ... rest of the component
};
This technique allows you to display real-time information or computed values in your node titles.
Custom Connection Types
Flowchart React supports different types of connections to represent various relationships:
const CustomConnections: React.FC = () => {
const [connections, setConnections] = useState<ConnectionData[]>([
{
source: { id: 1, position: "right" },
destination: { id: 2, position: "left" },
type: "success",
title: "Approved"
},
{
source: { id: 1, position: "bottom" },
destination: { id: 3, position: "top" },
type: "fail",
title: "Rejected",
color: "#ff0000"
}
]);
// ... rest of the component
};
By specifying different type
and color
properties, you can create connections that visually represent the nature of the relationship between nodes.
Implementing Interactivity
To make your flowchart truly interactive, you can leverage the various event handlers provided by Flowchart React:
const InteractiveFlowchart: React.FC = () => {
// ... state setup
return (
<Flowchart
nodes={nodes}
connections={connections}
onChange={(newNodes, newConnections) => {
setNodes(newNodes);
setConnections(newConnections);
}}
onNodeDoubleClick={(node) => {
console.log(`Node ${node.id} was double-clicked`);
// Implement edit functionality here
}}
onConnectionDoubleClick={(connection) => {
console.log(`Connection between ${connection.source.id} and ${connection.destination.id} was double-clicked`);
// Implement connection edit functionality here
}}
onDoubleClick={(event, zoom) => {
const newNode: NodeData = {
id: Date.now(),
type: "operation",
title: "New Node",
x: event.nativeEvent.offsetX / zoom,
y: event.nativeEvent.offsetY / zoom
};
setNodes([...nodes, newNode]);
}}
/>
);
};
These event handlers allow you to respond to user interactions, enabling features like node editing, connection modification, and dynamic node creation.
Wrapping Up Our Flowchart Journey
Flowchart React offers a powerful set of tools for creating interactive and visually appealing flowcharts in your React applications. From basic diagrams to complex, data-driven visualizations, this library provides the flexibility and ease of use needed to bring your ideas to life.
By leveraging its customization options, event handling capabilities, and intuitive API, you can create flowcharts that not only look great but also provide meaningful interactivity for your users. Whether you’re mapping out business processes, designing decision trees, or visualizing data flows, Flowchart React empowers you to create clear, engaging, and dynamic visual representations.
As you continue to explore and experiment with Flowchart React, you’ll discover even more ways to enhance your applications with powerful visual storytelling. Happy flowcharting!