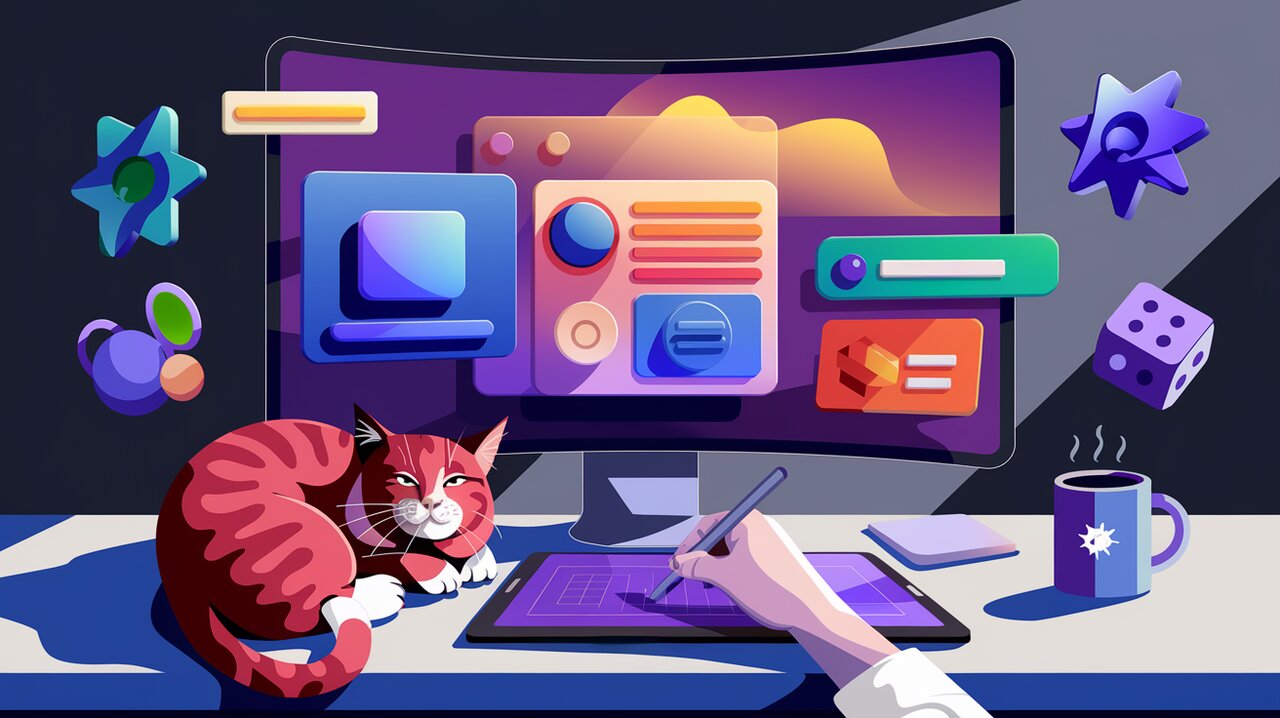
Orchestrating UI Harmony with Fluent UI React
Fluent UI React, formerly known as Office UI Fabric React, is Microsoft’s powerful UI library for building modern web applications. It provides a comprehensive set of React components that implement Microsoft’s Fluent Design System, enabling developers to create stunning, accessible, and consistent user interfaces with ease. In this article, we’ll explore the features, installation process, and usage of Fluent UI React, helping you orchestrate a symphony of beautiful and functional UI components in your React applications.
Key Features of Fluent UI React
Fluent UI React offers a wealth of features that make it a top choice for developers:
- Extensive Component Library: A wide range of pre-built, customizable React components.
- Accessibility: Built-in accessibility features to ensure your applications are usable by everyone.
- Responsive Design: Components that adapt seamlessly to different screen sizes and devices.
- Customization: Flexible theming and styling options to match your brand identity.
- Performance: Optimized for speed and efficiency in React applications.
- TypeScript Support: Full TypeScript definitions for enhanced developer productivity.
Getting Started with Fluent UI React
Let’s dive into how you can start using Fluent UI React in your projects.
Installation
To begin your journey with Fluent UI React, you’ll need to install the package in your React project. You can do this using npm or yarn:
npm install @fluentui/react
or
yarn add @fluentui/react
Basic Usage
Once installed, you can start importing and using Fluent UI React components in your application. Here’s a simple example of how to use a button component:
import React from 'react';
import { DefaultButton } from '@fluentui/react';
const MyComponent: React.FC = () => {
return (
<DefaultButton onClick={() => console.log('Button clicked!')}>
Click me!
</DefaultButton>
);
};
export default MyComponent;
This code snippet demonstrates how easy it is to add a Fluent UI button to your React component. The DefaultButton
component comes with built-in styling and accessibility features, making it simple to create professional-looking UI elements.
Exploring Fluent UI React Components
Fluent UI React offers a wide array of components to suit various UI needs. Let’s explore some of the most commonly used components and how to implement them.
Text Inputs
Text inputs are fundamental to any form. Fluent UI React provides the TextField
component for this purpose:
import React from 'react';
import { TextField } from '@fluentui/react';
const FormComponent: React.FC = () => {
return (
<TextField
label="Enter your name"
placeholder="John Doe"
onChange={(event, newValue) => console.log(newValue)}
/>
);
};
The TextField
component offers built-in label support and handles state changes, making form creation a breeze.
Dropdown Menus
For selection inputs, the Dropdown
component is an excellent choice:
import React from 'react';
import { Dropdown, IDropdownOption } from '@fluentui/react';
const options: IDropdownOption[] = [
{ key: 'apple', text: 'Apple' },
{ key: 'banana', text: 'Banana' },
{ key: 'orange', text: 'Orange' },
];
const DropdownComponent: React.FC = () => {
return (
<Dropdown
placeholder="Select a fruit"
options={options}
onChange={(event, option) => console.log(option?.text)}
/>
);
};
This example shows how to create a dropdown menu with predefined options, demonstrating the simplicity and power of Fluent UI React components.
Advanced Usage and Customization
Fluent UI React isn’t just about basic components; it also offers advanced features for more complex UI requirements.
Theming
One of the strengths of Fluent UI React is its theming capabilities. You can easily customize the look and feel of your components to match your brand:
import React from 'react';
import { ThemeProvider, createTheme, DefaultButton } from '@fluentui/react';
const myTheme = createTheme({
palette: {
themePrimary: '#0078d4',
themeLighterAlt: '#eff6fc',
themeLighter: '#deecf9',
themeLight: '#c7e0f4',
themeTertiary: '#71afe5',
themeSecondary: '#2b88d8',
themeDarkAlt: '#106ebe',
themeDark: '#005a9e',
themeDarker: '#004578',
},
});
const ThemedComponent: React.FC = () => {
return (
<ThemeProvider theme={myTheme}>
<DefaultButton>Themed Button</DefaultButton>
</ThemeProvider>
);
};
This example demonstrates how to create a custom theme and apply it to your components using the ThemeProvider
.
Responsive Design
Fluent UI React components are designed to be responsive out of the box. However, for more complex layouts, you can use the Stack
component to create flexible, responsive designs:
import React from 'react';
import { Stack, IStackTokens, DefaultButton } from '@fluentui/react';
const stackTokens: IStackTokens = { childrenGap: 20 };
const ResponsiveComponent: React.FC = () => {
return (
<Stack tokens={stackTokens} horizontal wrap>
<DefaultButton>Button 1</DefaultButton>
<DefaultButton>Button 2</DefaultButton>
<DefaultButton>Button 3</DefaultButton>
</Stack>
);
};
This code creates a horizontal stack of buttons that will wrap to the next line on smaller screens, ensuring your UI remains usable across different device sizes.
Conclusion
Fluent UI React is a powerful tool in the React developer’s arsenal, offering a comprehensive suite of components that implement Microsoft’s Fluent Design System. With its focus on accessibility, performance, and customization, it enables developers to create beautiful, functional, and inclusive user interfaces with ease.
By leveraging Fluent UI React, you can significantly speed up your development process while ensuring a consistent and professional look across your applications. Whether you’re building a simple form or a complex enterprise application, Fluent UI React provides the tools you need to orchestrate a symphony of UI components that will delight your users.
As you continue your journey with Fluent UI React, you might find it helpful to explore related topics. Check out our articles on React Beautiful DND Exploration for insights into drag-and-drop functionality, or React Big Calendar Guide to learn about implementing calendars in your React applications. These resources can complement your Fluent UI React knowledge and help you create even more dynamic and interactive user interfaces.