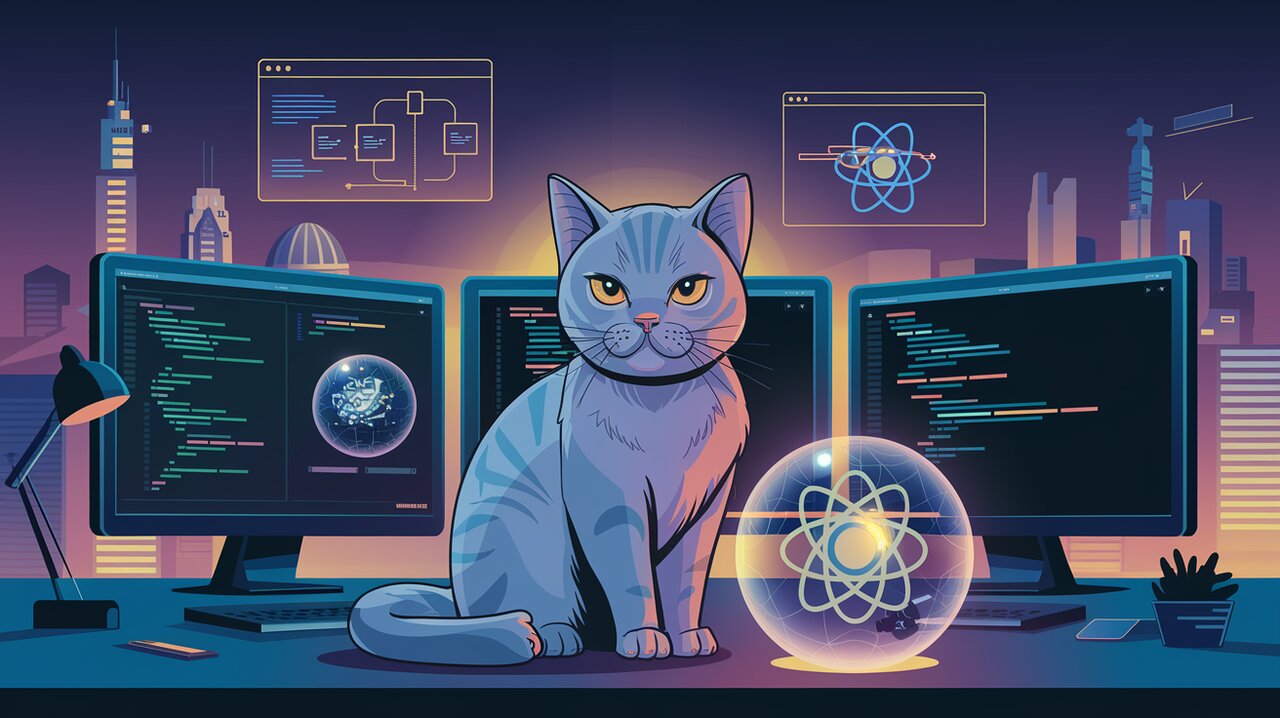
Future-Proofing Redux: Taming Time with redux-future
In the ever-evolving landscape of React and Redux applications, handling asynchronous operations can be a challenging endeavor. Enter redux-future, a powerful middleware that brings the elegance and predictability of Future monads to your Redux workflow. This library allows developers to manage time-dependent actions with grace and precision, making complex asynchronous flows feel like a breeze.
Embracing the Future
redux-future is designed to work seamlessly with Flux Standard Action (FSA) compliant actions, ensuring consistency across your Redux ecosystem. By leveraging the Future monad, it provides a clean and functional approach to dealing with asynchronous operations.
Key Features
- FSA-compliant action handling
- Seamless integration with existing Redux setups
- Elegant error handling for asynchronous operations
- Compatible with popular Redux utilities like redux-actions
Getting Started
To begin your journey with redux-future, you’ll need to install it in your project. Open your terminal and run one of the following commands:
npm install --save redux-future
Or if you prefer yarn:
yarn add redux-future
Basic Usage: Unleashing the Power of Futures
Once installed, you can start using redux-future in your Redux setup. Here’s how to integrate the middleware:
import { createStore, applyMiddleware } from 'redux';
import futureMiddleware from 'redux-future';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
applyMiddleware(futureMiddleware)
);
With the middleware in place, you can now dispatch actions that contain Futures. Let’s look at a basic example:
import Future from 'fluture';
const fetchUserData = (userId: string) => new Future((reject, resolve) => {
fetch(`https://api.example.com/users/${userId}`)
.then(response => response.json())
.then(resolve)
.catch(reject);
});
// In your component or action creator
store.dispatch(fetchUserData('123'));
In this example, fetchUserData
returns a Future that resolves with the user data. The redux-future middleware will automatically handle the resolution of this Future and dispatch the result as a new action.
Advanced Techniques: Composing Futures
One of the strengths of redux-future is its ability to work with composed Futures, allowing for complex asynchronous flows. Let’s explore a more advanced example:
Filtering and Transforming Results
import { compose, filter, gt, assoc } from 'ramda';
const fetchNumbers = new Future((reject, resolve) =>
resolve([1, 2, 3, 4, 5, 6])
);
const filterAndTransform = fetchNumbers.map(
compose(
assoc('numbers', R.__, { type: 'FILTER_NUMBERS' }),
filter(gt(3))
)
);
store.dispatch(filterAndTransform);
In this example, we fetch a list of numbers, filter out those greater than 3, and then associate the result with a ‘FILTER_NUMBERS’ action. The redux-future middleware will handle the entire process, dispatching the final action when the Future resolves.
Integrating with redux-actions
redux-future plays nicely with other Redux utilities, such as redux-actions. Here’s how you can use them together:
import { createAction } from 'redux-actions';
const fetchData = createAction('FETCH_DATA', () =>
new Future((reject, resolve) => {
setTimeout(() => resolve({ data: 'Async data' }), 1000);
})
);
// Usage
store.dispatch(fetchData());
This combination allows for clean, declarative action creators that handle asynchronous operations seamlessly.
Error Handling: Graceful Failure
redux-future doesn’t just handle successful resolutions; it also provides elegant error handling. If a Future is rejected, the middleware will dispatch an error action:
const riskyOperation = new Future((reject, resolve) => {
if (Math.random() > 0.5) {
resolve('Success!');
} else {
reject(new Error('Operation failed'));
}
});
store.dispatch(riskyOperation);
If the Future is rejected, an action with { type: 'RISKY_OPERATION', payload: Error, error: true }
will be dispatched, allowing your reducers to handle the error state appropriately.
Conclusion: Embracing the Future of Asynchronous Redux
redux-future brings the power and elegance of Future monads to the Redux ecosystem, offering a functional and composable approach to handling asynchronous operations. By leveraging this middleware, developers can write cleaner, more predictable code for complex asynchronous flows.
As you continue to explore redux-future, you’ll discover its flexibility in handling various asynchronous scenarios. Whether you’re fetching data, managing user interactions, or orchestrating complex business logic, redux-future provides the tools to make your Redux applications more robust and maintainable.
Remember, the world of Redux is vast, and redux-future is just one of many powerful tools at your disposal. Consider exploring related concepts like redux-saga for more advanced asynchronous flow control, or redux-observable for reactive programming paradigms in Redux.
By mastering redux-future, you’re taking a significant step towards writing more functional, composable, and time-aware Redux applications. Embrace the power of Futures, and watch your asynchronous code transform from a tangled web of callbacks into a elegant flow of data through time.