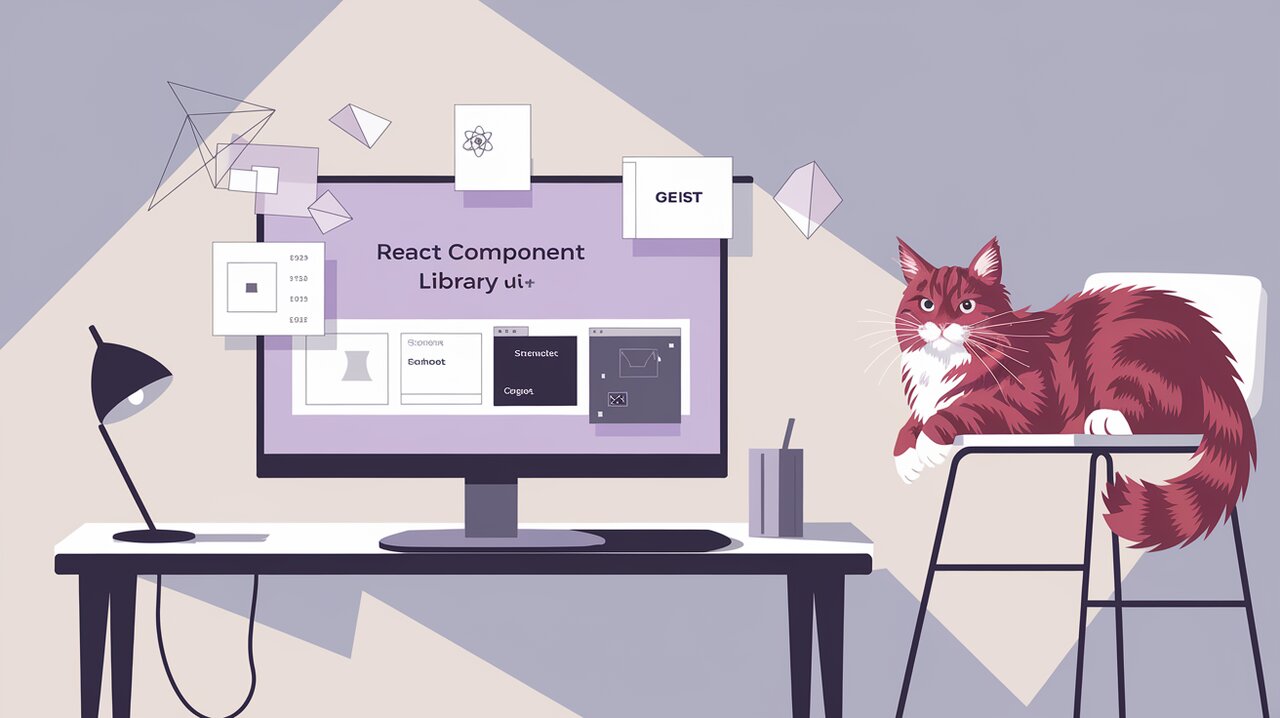
Geist UI: Conjuring Minimalist Magic in React
In the ever-evolving landscape of web development, creating sleek and efficient user interfaces is paramount. Enter Geist UI, a modern and minimalist React UI library that draws inspiration from Vercel’s design philosophy. This powerful toolkit empowers developers to craft stunning web experiences with ease, combining simplicity with functionality in a harmonious blend.
Unveiling the Magic of Geist UI
Geist UI offers a comprehensive set of features that make it a go-to choice for developers seeking a minimalist approach to UI design:
- Minimalist Design: Embrace clean, uncluttered interfaces that put your content front and center.
- Responsive Components: Build adaptive layouts that look great on any device or screen size.
- Customizable Themes: Tailor the look and feel of your application with ease using Geist UI’s theming system.
- Accessibility: Create inclusive experiences with built-in accessibility features.
- TypeScript Support: Enjoy full TypeScript support for type-safe development.
- Dark Mode: Implement dark mode effortlessly to enhance user experience.
Summoning Geist UI into Your Project
To begin your journey with Geist UI, you’ll need to summon it into your project. Open your terminal and cast one of these spells:
npm install @geist-ui/core
Or if you prefer the yarn incantation:
yarn add @geist-ui/core
Weaving the Basic Enchantments
Once you’ve summoned Geist UI, it’s time to weave it into the fabric of your React application. Let’s start with a basic incantation:
import React from 'react'
import { GeistProvider, CssBaseline } from '@geist-ui/core'
const App: React.FC = () => {
return (
<GeistProvider>
<CssBaseline />
<YourApp />
</GeistProvider>
)
}
export default App
This spell wraps your entire application in the GeistProvider
, which ensures that all Geist UI components have access to the necessary context. The CssBaseline
component normalizes styles across different browsers, providing a consistent foundation for your magical creations.
Conjuring a Button
Let’s start with a simple yet powerful component - the Button. Behold the incantation:
import React from 'react'
import { Button } from '@geist-ui/core'
const MagicButton: React.FC = () => {
return <Button>Cast Spell</Button>
}
This spell summons a beautifully styled button that adheres to Geist UI’s minimalist design principles. You can customize its appearance with various props, such as type
, ghost
, or loading
.
Crafting a Card of Wonder
Cards are versatile components that can hold various types of content. Let’s conjure one:
import React from 'react'
import { Card, Text } from '@geist-ui/core'
const EnchantedCard: React.FC = () => {
return (
<Card>
<Card.Content>
<Text h4>Magical Artifact</Text>
<Text>This card contains untold wonders and secrets.</Text>
</Card.Content>
<Card.Footer>Discovered on a moonlit night</Card.Footer>
</Card>
)
}
This spell creates a card with a header, body content, and a footer. The Card
component is highly flexible, allowing you to structure your content in various ways.
Advanced Incantations
As you delve deeper into the arcane arts of Geist UI, you’ll discover more powerful spells to enhance your user interfaces.
Summoning a Modal
Modals are excellent for focusing user attention on important information or actions. Here’s how to conjure one:
import React, { useState } from 'react'
import { Modal, Button, Text } from '@geist-ui/core'
const MysticModal: React.FC = () => {
const [visible, setVisible] = useState(false)
const handler = () => setVisible(true)
const closeHandler = () => setVisible(false)
return (
<div>
<Button auto onClick={handler}>Open Modal</Button>
<Modal visible={visible} onClose={closeHandler}>
<Modal.Title>Mystical Revelation</Modal.Title>
<Modal.Subtitle>Prepare for wonder</Modal.Subtitle>
<Modal.Content>
<Text>Behold the secrets of the universe!</Text>
</Modal.Content>
<Modal.Action passive onClick={closeHandler}>Cancel</Modal.Action>
<Modal.Action>Embrace</Modal.Action>
</Modal>
</div>
)
}
This advanced spell creates a button that, when clicked, reveals a modal with a title, subtitle, content, and action buttons. The useState
hook manages the visibility of the modal, allowing for smooth transitions.
Weaving a Grid of Elements
For more complex layouts, Geist UI offers a powerful Grid system. Observe this intricate weave:
import React from 'react'
import { Grid, Card, Text } from '@geist-ui/core'
const MagicalGrid: React.FC = () => {
return (
<Grid.Container gap={2} justify="center">
<Grid xs={12} md={6}>
<Card shadow>
<Text h4>Elemental Fire</Text>
<Text>Harness the power of flames.</Text>
</Card>
</Grid>
<Grid xs={12} md={6}>
<Card shadow>
<Text h4>Aquatic Mastery</Text>
<Text>Command the seas with a gesture.</Text>
</Card>
</Grid>
<Grid xs={12} md={6}>
<Card shadow>
<Text h4>Earthen Strength</Text>
<Text>Draw power from the very ground.</Text>
</Card>
</Grid>
<Grid xs={12} md={6}>
<Card shadow>
<Text h4>Winds of Change</Text>
<Text>Bend the air to your will.</Text>
</Card>
</Grid>
</Grid.Container>
)
}
This enchantment creates a responsive grid layout with four cards, each representing an elemental power. The grid adjusts seamlessly from a single column on small screens to two columns on medium and larger screens.
Conclusion: Mastering the Art of Geist UI
As we conclude our magical journey through the realm of Geist UI, you’ve witnessed the power and elegance of this minimalist React UI library. From basic components like buttons and cards to more advanced constructs like modals and grid systems, Geist UI provides a comprehensive toolkit for crafting beautiful, functional, and responsive user interfaces.
By embracing the principles of minimalism and leveraging the flexibility of Geist UI, you can create web experiences that are not only visually appealing but also highly performant and accessible. As you continue to explore and experiment with the various components and features, you’ll find that Geist UI empowers you to bring your creative visions to life with ease and precision.
Remember, the true magic of Geist UI lies not just in its components, but in how you wield them to create harmonious and intuitive user experiences. So go forth, fellow developer, and let your imagination run wild as you conjure interfaces that captivate and delight users across the digital landscape.
For those seeking to further enhance their React development skills, you might find inspiration in our articles on Mastering React Datepicker and Unleashing Redux Saga Power. These complementary tools can work in harmony with Geist UI to create even more powerful and dynamic web applications.