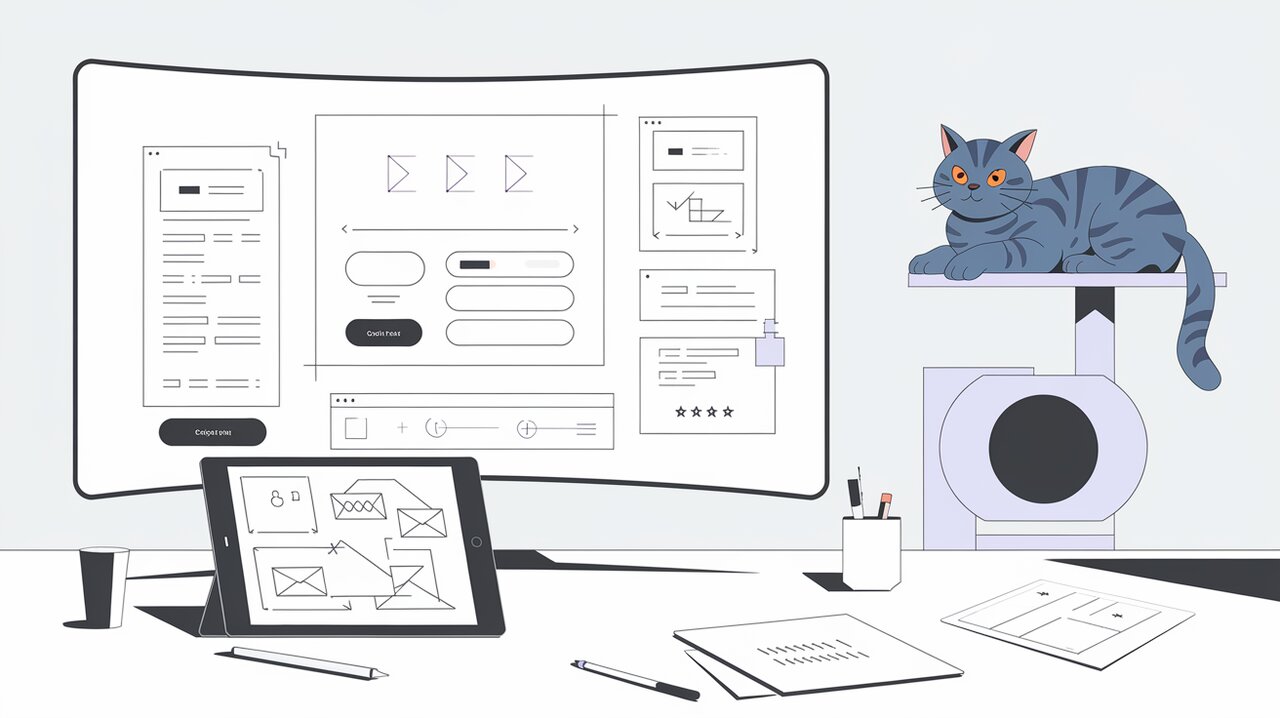
Geist UI React: Minimalist Magic for Modern Web Apps
Geist UI React, officially known as @geist-ui/core
, is a modern and minimalist React component library that brings the sleek design aesthetics of Vercel to your web applications. With its focus on simplicity and functionality, Geist UI empowers developers to create stunning user interfaces without sacrificing performance or customization options. Whether you’re building a personal portfolio, a cutting-edge web app, or anything in between, Geist UI provides the tools you need to craft beautiful, responsive designs with ease.
Enchanting Features of Geist UI
Geist UI comes packed with a variety of features that make it stand out in the crowded world of React UI libraries:
- Minimalist Design: Embrace the power of simplicity with clean, uncluttered components that put your content front and center.
- Dark Mode Support: Effortlessly switch between light and dark themes to cater to user preferences and reduce eye strain.
- Responsive Components: Build layouts that look great on any device, from mobile phones to large desktop screens.
- Customizable Theming: Tailor the look and feel of your application with easy-to-use theming options.
- TypeScript Support: Enjoy full TypeScript definitions for enhanced development experience and type safety.
- Accessibility: Create inclusive applications with components that adhere to web accessibility standards.
Conjuring Geist UI Into Your Project
To begin your journey with Geist UI, you’ll need to summon it into your React project. Open your terminal and cast one of the following spells:
# If you're a yarn wizard
yarn add @geist-ui/core
# For npm sorcerers
npm install @geist-ui/core
With the library now at your fingertips, you’re ready to weave some UI magic!
Crafting Your First Geist UI Spell
Let’s start by creating a basic application that showcases the power of Geist UI. First, we’ll set up the foundational structure:
import React from 'react'
import { GeistProvider, CssBaseline, Button } from '@geist-ui/core'
const App: React.FC = () => {
return (
<GeistProvider>
<CssBaseline />
<Button>Click me!</Button>
</GeistProvider>
)
}
export default App
In this enchanting snippet, we’ve introduced three key elements:
- GeistProvider: This component wraps your entire application, providing the necessary context for Geist UI components to function properly.
- CssBaseline: A magical reset that normalizes styles across different browsers, ensuring a consistent starting point for your designs.
- Button: A simple yet powerful component that demonstrates the sleek aesthetics of Geist UI.
Unleashing the Power of Geist UI Components
Now that we’ve laid the groundwork, let’s explore some of the more advanced incantations that Geist UI offers.
Summoning a Modal Dialog
Modals are an essential part of modern web applications. Geist UI makes creating them a breeze:
import React, { useState } from 'react'
import { Modal, Button, Text } from '@geist-ui/core'
const ModalExample: React.FC = () => {
const [visible, setVisible] = useState(false)
const handler = () => setVisible(true)
const closeHandler = () => setVisible(false)
return (
<div>
<Button auto onClick={handler}>Open Modal</Button>
<Modal visible={visible} onClose={closeHandler}>
<Modal.Title>Modal Title</Modal.Title>
<Modal.Subtitle>This is a modal</Modal.Subtitle>
<Modal.Content>
<Text>Some content contained within the modal.</Text>
</Modal.Content>
<Modal.Action passive onClick={closeHandler}>Cancel</Modal.Action>
<Modal.Action>Submit</Modal.Action>
</Modal>
</div>
)
}
This spell creates a button that, when clicked, conjures a modal dialog. The modal showcases various sub-components like titles, content, and action buttons, all styled consistently with the Geist UI design language.
Crafting a Responsive Grid Layout
Geist UI’s grid system allows you to create complex layouts with ease:
import React from 'react'
import { Grid, Card, Text } from '@geist-ui/core'
const GridExample: React.FC = () => {
return (
<Grid.Container gap={2} justify="center">
<Grid xs={24} md={12}>
<Card shadow>
<Text h4>Card 1</Text>
<Text>This card spans full width on mobile and half width on larger screens.</Text>
</Card>
</Grid>
<Grid xs={24} md={12}>
<Card shadow>
<Text h4>Card 2</Text>
<Text>This card also adapts its width based on the screen size.</Text>
</Card>
</Grid>
</Grid.Container>
)
}
This incantation creates a responsive grid layout with two cards. On mobile devices, each card takes up the full width, while on larger screens, they sit side by side, each occupying half the available space.
Advanced Geist UI Sorcery
For those ready to delve deeper into the arcane arts of Geist UI, let’s explore some more advanced techniques.
Theming Your Realm
Geist UI allows you to customize the look and feel of your application through theming:
import React from 'react'
import { GeistProvider, CssBaseline, Button } from '@geist-ui/core'
const myTheme = {
type: 'dark',
theme: {
palette: {
success: '#7CFC00',
error: '#FF6347',
},
},
}
const ThemedApp: React.FC = () => {
return (
<GeistProvider themes={[myTheme]} themeType="dark">
<CssBaseline />
<Button type="success">Success Button</Button>
<Button type="error">Error Button</Button>
</GeistProvider>
)
}
This spell creates a custom dark theme with modified success and error colors. The GeistProvider
is configured to use this theme, transforming the appearance of all Geist UI components within its realm.
Conjuring Dynamic Tables
For displaying data in a structured format, Geist UI’s Table component is a powerful tool:
import React from 'react'
import { Table } from '@geist-ui/core'
const data = [
{ name: 'Griffindor', house: 'Lion', points: 500 },
{ name: 'Hufflepuff', house: 'Badger', points: 350 },
{ name: 'Ravenclaw', house: 'Eagle', points: 450 },
{ name: 'Slytherin', house: 'Snake', points: 400 },
]
const TableExample: React.FC = () => {
return (
<Table data={data}>
<Table.Column prop="name" label="Name" />
<Table.Column prop="house" label="House" />
<Table.Column prop="points" label="Points" />
</Table>
)
}
This incantation summons a table that displays information about Hogwarts houses. The Table component automatically handles sorting and pagination, making it easy to present large datasets in a user-friendly manner.
Concluding Our Magical Journey
As we conclude our exploration of Geist UI React, it’s clear that this minimalist UI library offers a powerful set of tools for creating modern, sleek web applications. From basic components to advanced theming and layout options, Geist UI provides everything you need to bring your design visions to life with minimal effort and maximum style.
Whether you’re crafting a personal project or building the next big web application, Geist UI’s focus on simplicity, customization, and performance makes it an excellent choice for developers who value clean design and efficient workflows. As you continue your journey with Geist UI, remember that the true magic lies not just in the components themselves, but in how you combine and customize them to create unique and engaging user experiences.
So go forth, intrepid developer, and may your Geist UI creations be as enchanting as they are functional!