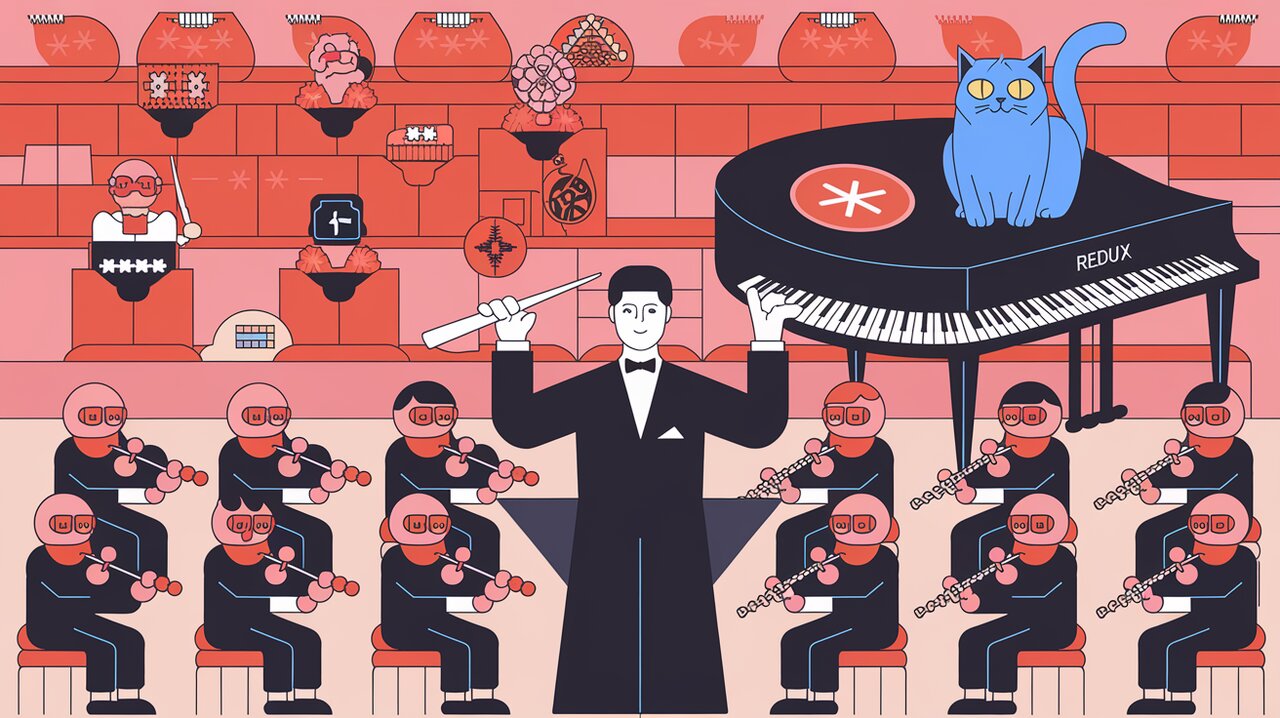
Orchestrating Redux Side Effects with redux-gen's Generator Symphony
In the world of React and Redux, managing side effects can often feel like conducting a complex symphony. Enter redux-gen, a powerful middleware that brings the elegance of generator functions to your Redux orchestra. This library allows you to compose your action creators as generators, effectively pushing all side effects to the edges of your application and creating a more harmonious Redux experience.
Composing Your Redux Symphony
redux-gen offers a unique approach to handling side effects in Redux applications. By leveraging generator functions, it provides several key features that can simplify your Redux workflow:
- Generator-based action creators for cleaner async logic
- Simplified side effect management
- Improved testability of action creators
- Better separation of concerns in your Redux architecture
Let’s dive into how you can start using redux-gen to orchestrate your Redux symphony.
Setting Up Your Redux Orchestra
To begin using redux-gen in your project, you’ll need to install it alongside your existing Redux setup. You can do this using npm or yarn:
npm install redux-gen
# or
yarn add redux-gen
Once installed, you’re ready to integrate redux-gen into your Redux store configuration.
Basic Usage: Your First Redux-Gen Composition
Configuring the Middleware
To use redux-gen, you’ll need to apply it as middleware when creating your Redux store. Here’s how you can set it up:
import { createStore, applyMiddleware } from 'redux'
import gen from 'redux-gen'
import rootReducer from './reducers'
const store = createStore(
rootReducer,
applyMiddleware(gen())
)
This setup applies the redux-gen middleware to your store, allowing you to use generator-based action creators.
Writing Generator Action Creators
Now that we have redux-gen set up, let’s look at how we can write action creators using generator functions:
function* fetchUsers() {
yield { type: 'FETCH_USERS_REQUEST' }
try {
const users = yield { type: 'FETCH', url: '/api/users' }
yield { type: 'FETCH_USERS_SUCCESS', payload: users }
} catch (error) {
yield { type: 'FETCH_USERS_FAILURE', error }
}
}
In this example, we’ve created a fetchUsers
generator function that yields multiple actions. The FETCH
action is intercepted by our custom middleware to perform the actual API call.
Advanced Usage: Orchestrating Complex Flows
Composing Multiple Generators
One of the strengths of redux-gen is its ability to compose multiple generator functions. This allows for complex workflows to be broken down into smaller, more manageable pieces:
function* fetchUserDetails(userId) {
const user = yield { type: 'FETCH', url: `/api/users/${userId}` }
const posts = yield { type: 'FETCH', url: `/api/users/${userId}/posts` }
return { user, posts }
}
function* fetchAllUserDetails() {
const userIds = yield { type: 'FETCH', url: '/api/users' }
const userDetails = yield userIds.map(fetchUserDetails)
yield { type: 'SET_USER_DETAILS', payload: userDetails }
}
This composition allows for a clear separation of concerns and makes your code more readable and maintainable.
Custom Middleware Integration
redux-gen shines when combined with custom middleware for handling specific side effects. Here’s an example of how you might integrate a custom fetch middleware:
const fetchMiddleware = ({ dispatch, getState }) => next => action => {
if (action.type !== 'FETCH') return next(action)
return fetch(action.url)
.then(response => response.json())
.catch(error => ({ error }))
}
const store = createStore(
rootReducer,
applyMiddleware(gen(), fetchMiddleware)
)
This setup allows your generator action creators to yield FETCH
actions that are automatically handled by the fetchMiddleware
.
Conclusion: A Harmonious Redux Experience
redux-gen brings a new level of clarity and organization to Redux applications by leveraging the power of generator functions. By pushing side effects to the edges of your application and allowing for more declarative action creators, it helps create a more maintainable and testable Redux architecture.
As you incorporate redux-gen into your projects, you’ll find that managing complex asynchronous flows becomes more intuitive and your code becomes more expressive. Whether you’re building a small React application or orchestrating a large-scale Redux symphony, redux-gen provides the tools you need to create harmonious and efficient state management solutions.
So why not give redux-gen a try in your next Redux project? You might just find that it’s the missing piece in your Redux orchestra, helping you create beautiful, well-structured applications with ease.