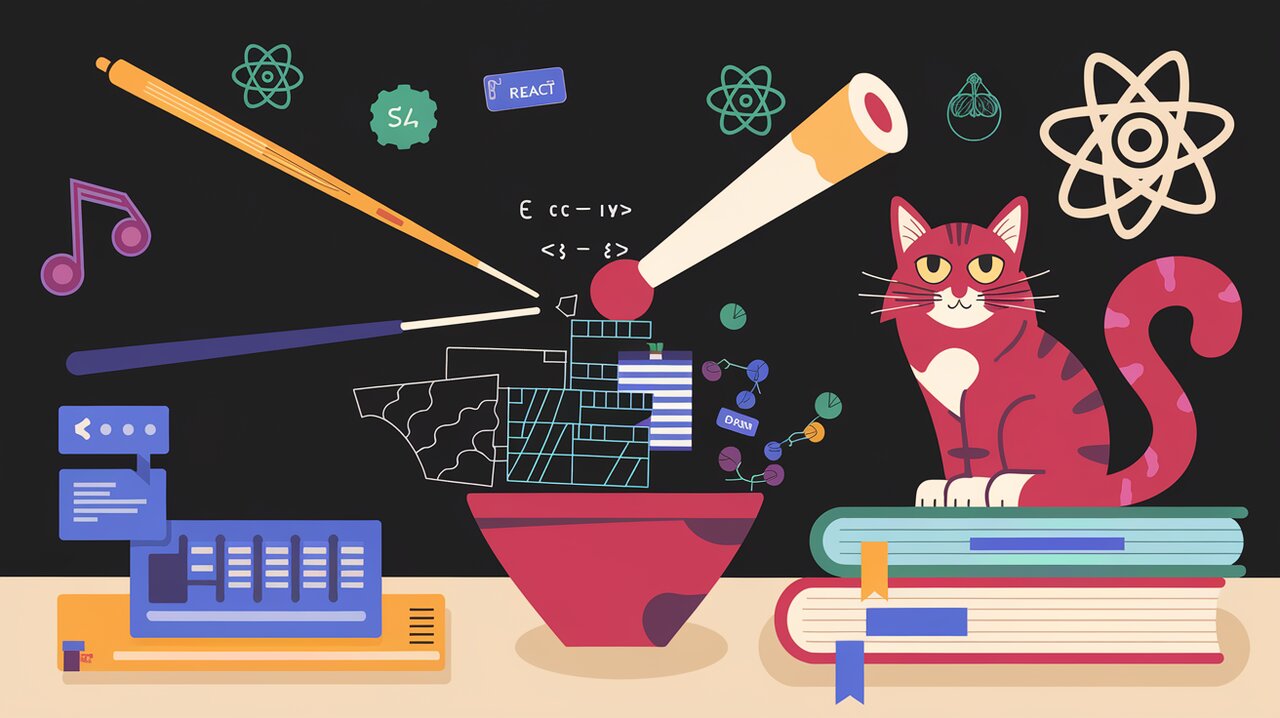
Orchestrating React Symphonies with generator-reactapp
In the ever-evolving world of web development, setting up a new React project can be a complex symphony of tools, configurations, and dependencies. Enter generator-reactapp
, a Yeoman generator that conducts this intricate process with the finesse of a seasoned maestro. This powerful tool harmonizes the setup of a comprehensive React application, allowing developers to focus on composing their code rather than orchestrating the project structure.
The Overture: What is generator-reactapp?
generator-reactapp is a Yeoman generator designed to quickly scaffold a React application with a rich ecosystem of popular libraries and tools. It’s like having a skilled conductor who knows exactly which instruments to bring together to create a masterpiece. This generator sets up not just the basic React structure, but also integrates routing, state management, styling solutions, and testing frameworks, among other essential components.
Features: A Symphony of Tools
The generator-reactapp
brings together a harmonious ensemble of features:
- React and its Ecosystem: Incorporates React, React Router, and React CSS Modules for a solid foundation.
- State Management: Includes both MobX and Redux, giving you the flexibility to choose your preferred state management library.
- API Handling: Integrates RESTful.js, fetch, and request for smooth API interactions.
- Data Normalization: Utilizes normalizr to keep your application state normalized.
- Testing Suite: Sets up a comprehensive testing environment with Mocha, Chai, Sinon, and Enzyme.
- Build Tools: Configures Webpack and Gulp for efficient building and bundling.
- Code Quality: Implements ESLint with Airbnb’s style guide for consistent code quality.
- CSS Processing: Includes PostCSS with Autoprefixer and PreCSS for advanced CSS capabilities.
Installation: Setting the Stage
Before we begin our performance, let’s set the stage by installing Yeoman and generator-reactapp
. Open your terminal and run:
npm install -g yo
npm install -g generator-reactapp
With these global installations, you’re ready to generate your React application.
Basic Usage: Composing Your First Movement
To create a new React project, simply run:
yo reactapp
This command will prompt you with a series of questions to customize your project setup. Once completed, it will orchestrate the creation of your project structure, install dependencies, and set up configuration files.
The Project Structure
After running the generator, you’ll find yourself with a well-organized project structure:
my-react-app/
├── src/
│ ├── components/
│ ├── containers/
│ ├── actions/
│ ├── reducers/
│ ├── sagas/
│ ├── styles/
│ ├── index.jsx
│ └── config.js
├── test/
├── .babelrc
├── .eslintrc
├── webpack.config.js
├── gulpfile.js
└── package.json
This structure separates concerns and follows best practices for React application development.
Advanced Usage: Crafting Complex Compositions
Generating Features
generator-reactapp
allows you to add new features to your existing project. Use the sub-generator to create new feature components:
yo reactapp:feature
This will prompt you for the feature name and create the necessary files, including component, container, actions, and reducers if you’re using Redux.
Customizing the Build Process
The generated webpack.config.js
and gulpfile.js
are set up with sensible defaults, but you can customize them to fit your project’s specific needs. For example, to add a new loader to Webpack:
// webpack.config.js
module.exports = {
// ... existing config
module: {
rules: [
// ... existing rules
{
test: /\.csv$/,
use: 'csv-loader'
}
]
}
};
Implementing State Management
Depending on your choice during setup, you’ll have either Redux or MobX configured. Here’s a quick example of setting up a Redux store:
// src/store/configureStore.ts
import { createStore, applyMiddleware } from 'redux';
import createSagaMiddleware from 'redux-saga';
import rootReducer from '../reducers';
import rootSaga from '../sagas';
const sagaMiddleware = createSagaMiddleware();
export default function configureStore(initialState = {}) {
const store = createStore(
rootReducer,
initialState,
applyMiddleware(sagaMiddleware)
);
sagaMiddleware.run(rootSaga);
return store;
}
Testing: Ensuring Harmonic Performance
The generator sets up a testing environment using Mocha, Chai, and Enzyme. Here’s an example of a simple component test:
// test/components/MyComponent.test.tsx
import React from 'react';
import { expect } from 'chai';
import { shallow } from 'enzyme';
import MyComponent from '../../src/components/MyComponent';
describe('<MyComponent />', () => {
it('renders without crashing', () => {
const wrapper = shallow(<MyComponent />);
expect(wrapper.exists()).to.be.true;
});
});
Run your tests using the npm script:
npm test
Conclusion: The Final Applause
generator-reactapp
orchestrates a harmonious setup for React applications, bringing together a symphony of tools and best practices. By automating the initial setup and providing a solid foundation, it allows developers to focus on creating beautiful and functional user interfaces without getting bogged down in configuration details.
As you continue to develop your React skills, you might find interest in exploring other React libraries and tools. For instance, you could enhance your project’s UI with the “Chakra UI React Symphony” or dive deeper into state management with the “Redux Rhapsody: Orchestrating React State”. These articles, available on our site, can provide further insights into expanding your React application’s capabilities.
Remember, like any good symphony, your React application can always be refined and expanded. generator-reactapp
gives you the perfect starting point – now it’s up to you to compose your masterpiece. Happy coding!