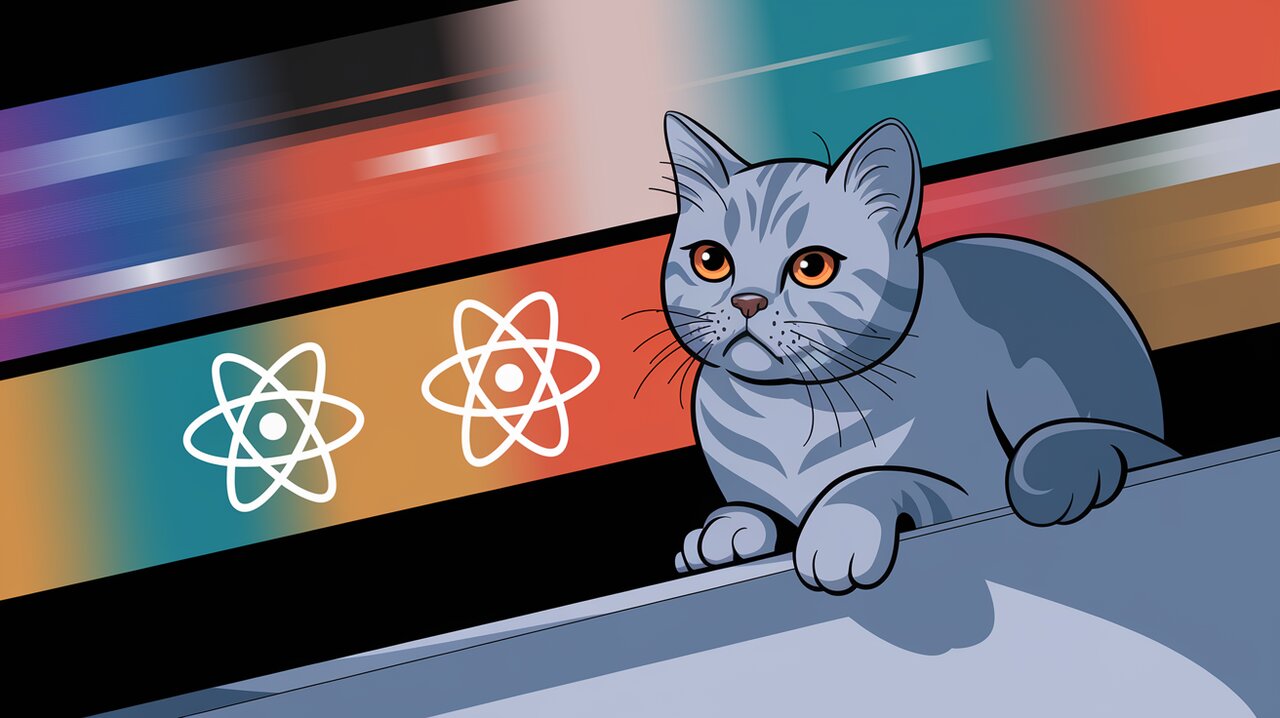
React developers are constantly on the lookout for efficient and elegant ways to showcase content. Enter react-glider, a powerful React wrapper for the popular Glider.js library that brings smooth, responsive carousels to your applications with minimal effort.
Sliding into react-glider
react-glider offers a seamless way to implement carousels in your React projects. It combines the flexibility of React with the robust functionality of Glider.js, resulting in a component that’s both easy to use and highly customizable.
Key Features
- Responsive Design: Adapts to various screen sizes effortlessly.
- Touch-enabled: Smooth swiping on touch devices.
- Customizable Controls: Easy implementation of arrows and dots for navigation.
- Dynamic Slide Count: Adjust the number of visible slides based on viewport.
- Smooth Animations: Glide through slides with buttery-smooth transitions.
Getting Started
Installation
Begin your journey with react-glider by installing it via npm:
npm install react-glider
Don’t forget to include the CSS file for proper styling:
import 'glider-js/glider.min.css';
Basic Usage
Here’s a simple example to get you started:
import React from 'react';
import Glider from 'react-glider';
import 'glider-js/glider.min.css';
function MyCarousel() {
return (
<Glider
draggable
hasArrows
hasDots
slidesToShow={2}
slidesToScroll={1}
>
<div>Slide 1</div>
<div>Slide 2</div>
<div>Slide 3</div>
<div>Slide 4</div>
</Glider>
);
}
This code creates a basic carousel with navigation arrows, dot indicators, and the ability to drag slides on touch devices.
Customization Magic
Responsive Breakpoints
react-glider shines when it comes to responsiveness. You can define different settings for various screen sizes:
<Glider
responsive={[
{
breakpoint: 768,
settings: {
slidesToShow: 2,
slidesToScroll: 1,
},
},
{
breakpoint: 1024,
settings: {
slidesToShow: 3,
slidesToScroll: 1,
},
},
]}
>
{/* Your slides here */}
</Glider>
Custom Navigation
Want to use your own navigation elements? No problem:
function CustomNavigation() {
const prevRef = React.useRef(null);
const nextRef = React.useRef(null);
return (
<>
<Glider
arrows={{
prev: prevRef.current,
next: nextRef.current,
}}
>
{/* Your slides here */}
</Glider>
<button ref={prevRef}>Previous</button>
<button ref={nextRef}>Next</button>
</>
);
}
Advanced Techniques
Accessing Glider Methods
react-glider exposes the underlying Glider instance, allowing you to call its methods directly:
function AdvancedCarousel() {
const gliderRef = React.useRef(null);
const scrollToRandom = () => {
const randomIndex = Math.floor(Math.random() * 5);
gliderRef.current?.scrollItem(randomIndex);
};
return (
<>
<Glider ref={gliderRef}>
{/* Your slides here */}
</Glider>
<button onClick={scrollToRandom}>Go to Random Slide</button>
</>
);
}
Event Handling
react-glider provides various events you can hook into:
<Glider
onSlideVisible={(e) => console.log('Slide visible:', e)}
onAnimated={() => console.log('Animation complete')}
>
{/* Your slides here */}
</Glider>
Performance Considerations
While react-glider is highly performant, keep these tips in mind for optimal results:
- Lazy Loading: For image-heavy carousels, consider implementing lazy loading to improve initial load times.
- Slide Count: Be mindful of the number of slides. Too many can impact performance, especially on mobile devices.
- Animation Duration: Adjust the
duration
prop for smoother animations on slower devices.
Conclusion
react-glider brings the power of Glider.js to the React ecosystem, offering a flexible and feature-rich solution for implementing carousels. Its ease of use, coupled with extensive customization options, makes it a top choice for developers looking to add smooth, responsive sliders to their React applications.
Whether you’re building a product showcase, a testimonial slider, or an image gallery, react-glider provides the tools you need to create engaging, interactive carousels that will delight your users.
Ready to take your React carousels to the next level? Dive into react-glider and start gliding through your content with style and ease!
For more React UI component insights, check out our articles on React Carousel Chronicles and Embla Carousel React Smooth Sliding Symphony.