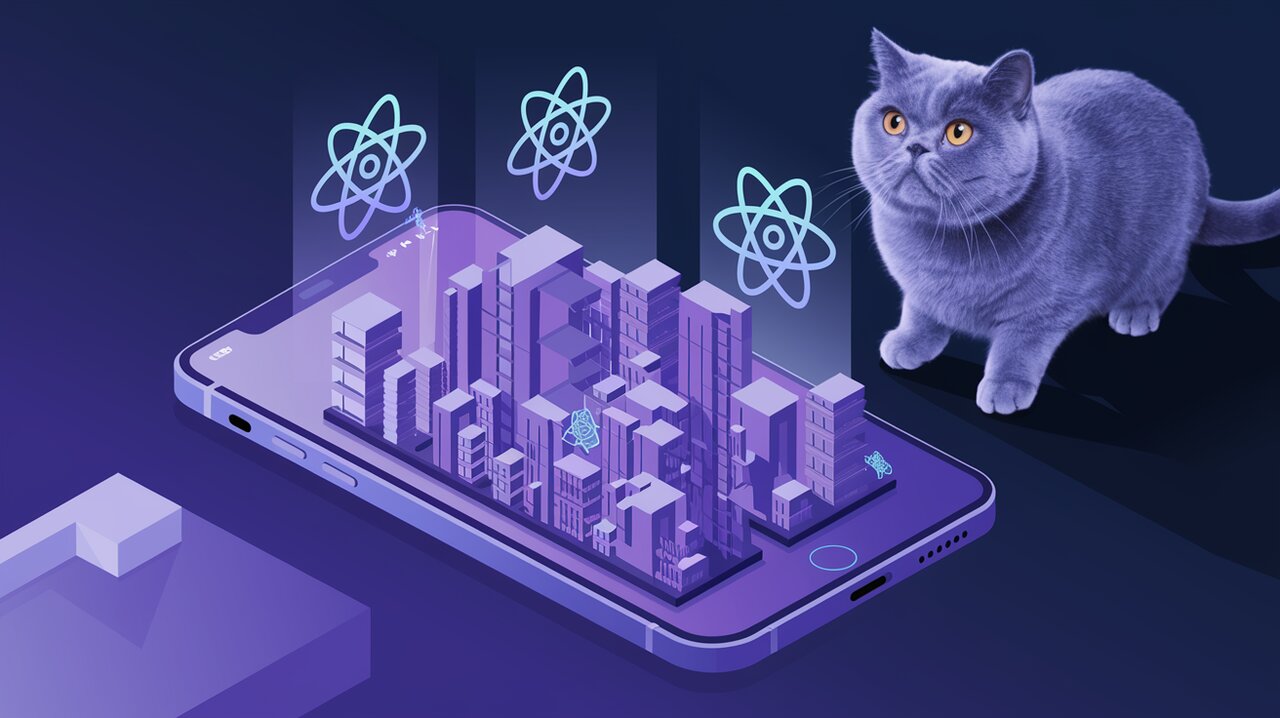
Unleash 3D Magic in React Native with react-native-godot
React Native developers, prepare to embark on a journey into the third dimension! The react-native-godot
library opens up a world of possibilities by bringing the power of Godot’s 3D engine to your React Native applications. Whether you’re looking to create immersive 3D experiences or develop interactive games, this library provides the tools you need to elevate your mobile apps to new heights.
Bridging Two Worlds
react-native-godot
serves as a bridge between React Native and Godot, allowing developers to harness the strengths of both frameworks. React Native provides a familiar and efficient way to build cross-platform mobile applications, while Godot offers a robust 3D engine with powerful graphics capabilities. By combining these technologies, you can create visually stunning and interactive 3D experiences within your React Native apps.
Features That Shine
The react-native-godot
library comes packed with features that make 3D development in React Native a breeze:
- Native Performance: Utilizing C++ JSI, the library ensures high-performance integration between React Native and Godot.
- GPU Acceleration: Harness the power of Metal on iOS and OpenGL/Vulkan on Android for smooth, hardware-accelerated graphics.
- Cross-Architecture Support: Works seamlessly with both old and new React Native architectures.
- Godot Variants in React Native: Access and manipulate Godot’s data types directly in your React Native code.
- GDScript Integration: Call GDScript methods from your React Native components, bridging the gap between the two environments.
- Easy Project Import: Seamlessly import your existing Godot projects into your React Native application.
Getting Started
To begin your 3D adventure with react-native-godot
, you’ll need to have Godot 4.3 installed on your development machine. Once you have Godot set up, you can install the library in your React Native project:
npm install react-native-godot
# or
yarn add react-native-godot
Bringing Godot to Life in React Native
Let’s dive into how you can use react-native-godot
to add 3D elements to your React Native app. Here’s a basic example of how to set up a Godot view and interact with it:
import React, { useRef, useEffect } from 'react';
import { Godot, GodotView, GodotProvider, useGodot } from 'react-native-godot';
const GodotScene = () => {
const godotRef = useRef<GodotView>(null);
const { Vector3 } = useGodot();
useEffect(() => {
if (godotRef.current?.isReady) {
// Send a message to Godot
godotRef.current.emitMessage({
message: 'Hello from React Native!',
position: Vector3(1, 2, 3),
});
// Access a node in the Godot scene
const node = godotRef.current.getRoot()?.getNode('MyNode');
if (node) {
node.hello_world();
}
}
}, [godotRef.current?.isReady]);
return (
<Godot
ref={godotRef}
style={{ flex: 1 }}
source={require('./assets/game.pck')}
scene="res://main.tscn"
onMessage={(message) => console.log('Godot message:', message)}
/>
);
};
const App = () => (
<GodotProvider>
<GodotScene />
</GodotProvider>
);
export default App;
In this example, we’re setting up a Godot view, sending messages to the Godot environment, and interacting with nodes in the Godot scene. The GodotProvider
component is crucial for initializing Godot properly and should be placed at the root of your app.
Bridging the Gap: React Native and Godot Communication
One of the most powerful features of react-native-godot
is the ability to communicate between React Native and Godot. This two-way communication allows you to create dynamic and interactive 3D experiences that respond to user input and app state changes.
Sending Messages from React Native to Godot
You can send messages from React Native to Godot using the emitMessage
method:
godotRef.current?.emitMessage({
message: 'Update player position',
position: Vector3(10, 0, 5),
});
Receiving Messages from Godot in React Native
To receive messages from Godot, use the onMessage
prop of the Godot
component:
<Godot
// ... other props
onMessage={(message) => {
console.log('Received from Godot:', message);
// Handle the message, update React Native state, etc.
}}
/>
Godot Script Integration
On the Godot side, you’ll need to set up your GDScript to handle messages from React Native and send messages back. Here’s a basic example of how your Godot script might look:
extends Node
func _ready():
if Engine.has_singleton("ReactNative"):
Engine.get_singleton("ReactNative").on_message(self, "_on_message")
func _on_message(message):
print("Received message from React Native:", message)
# Handle the message, update game state, etc.
func send_to_react_native():
if Engine.has_singleton("ReactNative"):
Engine.get_singleton("ReactNative").emit_message({
"event": "score_update",
"score": 100
})
This script sets up a message handler in Godot and provides a method to send messages back to React Native.
Advanced Usage: Godot Variants in React Native
react-native-godot
allows you to use Godot’s data types (Variants) directly in your React Native code. This includes types like Vector3
, Vector2
, Color
, and more. Here’s an example of how you might use these in your React Native component:
const { Vector3, Color } = useGodot();
// Create a new Vector3
const position = Vector3(1, 2, 3);
// Use Vector3 methods
console.log('Length:', position.length());
// Create a Color
const color = Color(1, 0, 0, 1); // Red
// Access color components
console.log('Red component:', color.r);
This tight integration between React Native and Godot data types allows for seamless manipulation of 3D objects and properties.
Conclusion: A New Dimension for React Native
react-native-godot
opens up a world of possibilities for React Native developers looking to incorporate 3D graphics and game-like experiences into their mobile apps. By bridging the gap between React Native’s component-based architecture and Godot’s powerful 3D engine, this library enables the creation of rich, interactive 3D applications that were previously challenging to implement in React Native alone.
As you explore the capabilities of react-native-godot
, you’ll find that it not only enhances your app’s visual appeal but also expands the types of applications you can create with React Native. From interactive product visualizations to full-fledged mobile games, the possibilities are limited only by your imagination.
For more insights into enhancing your React Native applications, check out our articles on react-native-skia for 2D graphics and react-native-reanimated for fluid animations. These libraries, combined with react-native-godot
, form a powerful toolkit for creating visually stunning and highly interactive mobile experiences.
Embrace the third dimension in your React Native projects with react-native-godot
, and watch as your apps come to life with immersive 3D graphics and interactivity!