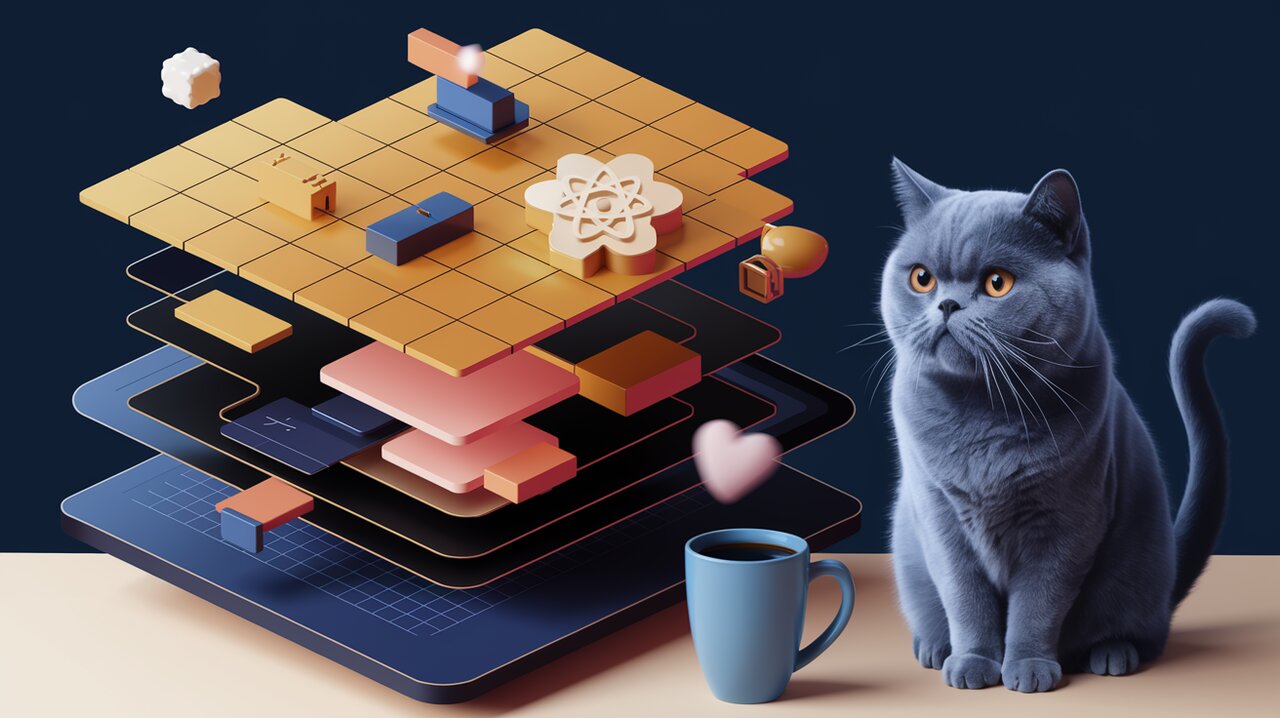
Golden Layout is a powerful JavaScript layout manager that brings a new level of flexibility and interactivity to React applications. It allows developers to create dynamic, draggable, and resizable layouts that users can customize to their liking. With its rich feature set and seamless integration with React, Golden Layout opens up a world of possibilities for creating complex, user-friendly interfaces.
Harmonizing Your UI with Golden Layout’s Features
Golden Layout comes packed with an impressive array of features that make it a standout choice for React developers:
- Native Popup Windows: Easily create detachable components that users can pop out into separate browser windows.
- Touch Support: Ensure your layouts work smoothly on touch-enabled devices.
- Framework Compatibility: Seamlessly integrate with popular frameworks like React, Angular, and Vue.
- Virtual Components: Utilize Golden Layout’s virtual component system for efficient rendering and management.
- Comprehensive API: Access a rich set of methods and events for fine-grained control over your layout.
- Persistence: Save and load layouts to provide a consistent experience across sessions.
- Focus Management: Implement intuitive focus handling for keyboard navigation.
- Theming: Customize the look and feel to match your application’s design.
- Browser Support: Works reliably in modern browsers like Firefox and Chrome.
- Responsive Design: Create layouts that adapt to different screen sizes and orientations.
Installing the Golden Layout Symphony
To begin orchestrating your React layouts with Golden Layout, you’ll need to install the library. You can do this using either npm or yarn:
# Using npm
npm install golden-layout
# Using yarn
yarn add golden-layout
Once installed, you’re ready to start composing your layout masterpiece.
Composing Your First Golden Layout
Let’s start by creating a basic layout with Golden Layout in a React application. First, we’ll set up the necessary imports and create a simple component structure.
Setting the Stage
import React, { useRef, useEffect } from 'react';
import { GoldenLayout, LayoutConfig } from 'golden-layout';
import 'golden-layout/dist/css/goldenlayout-base.css';
import 'golden-layout/dist/css/themes/goldenlayout-dark-theme.css';
const MyLayout: React.FC = () => {
const layoutRef = useRef<GoldenLayout | null>(null);
const containerRef = useRef<HTMLDivElement>(null);
useEffect(() => {
if (containerRef.current) {
const layout = new GoldenLayout(containerRef.current);
layoutRef.current = layout;
// We'll add our configuration here
layout.init();
}
}, []);
return <div ref={containerRef} style={{ width: '100%', height: '600px' }} />;
};
export default MyLayout;
In this setup, we’re creating a container for our layout and initializing Golden Layout within a useEffect
hook. The containerRef
is used to provide Golden Layout with the DOM element it should render into.
Arranging the Components
Now, let’s add some components to our layout. We’ll create a configuration that splits the screen into two columns, with the right column further divided into two rows.
const config: LayoutConfig = {
root: {
type: 'row',
content: [
{
type: 'component',
componentName: 'example',
componentState: { label: 'A' },
title: 'Component A',
width: 30
},
{
type: 'column',
content: [
{
type: 'component',
componentName: 'example',
componentState: { label: 'B' },
title: 'Component B'
},
{
type: 'component',
componentName: 'example',
componentState: { label: 'C' },
title: 'Component C'
}
]
}
]
}
};
layout.loadLayout(config);
This configuration creates a layout with three components: A, B, and C. Component A takes up 30% of the width on the left, while B and C are stacked vertically on the right.
Bringing Components to Life
To make our layout functional, we need to register the components we’ve defined. Let’s create a simple example component and register it with Golden Layout.
const ExampleComponent: React.FC<{ label: string }> = ({ label }) => {
return <div>{`I am component ${label}`}</div>;
};
layout.registerComponent('example', ExampleComponent);
By registering the component, we’re telling Golden Layout how to render our example
components when they’re referenced in the configuration.
Advanced Compositions with Golden Layout
Now that we’ve got the basics down, let’s explore some more advanced features of Golden Layout that can add depth and interactivity to your React applications.
Conducting Responsive Layouts
Golden Layout can adapt to different screen sizes, making it perfect for responsive designs. Let’s modify our layout to be responsive:
const responsiveConfig: LayoutConfig = {
settings: {
responsiveMode: 'always'
},
dimensions: {
minItemWidth: 200,
minItemHeight: 200
},
content: [
{
type: 'row',
content: [
{
type: 'component',
componentName: 'example',
componentState: { label: 'Responsive A' },
title: 'Responsive Component A'
},
{
type: 'component',
componentName: 'example',
componentState: { label: 'Responsive B' },
title: 'Responsive Component B'
}
]
}
]
};
layout.loadLayout(responsiveConfig);
This configuration enables responsive mode and sets minimum dimensions for items. The layout will automatically adjust as the screen size changes.
Orchestrating Popout Windows
One of Golden Layout’s standout features is the ability to create popout windows. Let’s add this functionality to a component:
const PopoutComponent: React.FC<{ glContainer: any }> = ({ glContainer }) => {
const handlePopout = () => {
glContainer.parent.popout();
};
return (
<div>
<button onClick={handlePopout}>Popout</button>
<p>Click to open in a new window!</p>
</div>
);
};
layout.registerComponent('popout', PopoutComponent);
Now you can add this component to your layout configuration, and users will be able to pop it out into a separate window.
Composing with Virtual Components
Virtual components are particularly useful when working with frameworks like React. They allow you to create components that Golden Layout manages without directly manipulating the DOM.
const VirtualComponent: React.FC = () => {
return <div>I'm a virtual component!</div>;
};
layout.createComponentCreator(VirtualComponent, 'virtual');
const virtualConfig: LayoutConfig = {
root: {
type: 'component',
componentName: 'virtual',
title: 'Virtual Component'
}
};
layout.loadLayout(virtualConfig);
This approach gives you more control over how your React components are rendered within the Golden Layout structure.
Finale: Bringing It All Together
Golden Layout provides a powerful set of tools for creating flexible, interactive layouts in React applications. By leveraging its features such as responsive design, popout windows, and virtual components, you can create rich user interfaces that adapt to user needs and preferences.
As you continue to explore Golden Layout, you’ll discover even more ways to enhance your React applications. From saving and loading layouts to implementing complex drag-and-drop interactions, Golden Layout offers a comprehensive solution for managing complex UI arrangements.
Remember to consult the Golden Layout documentation for the most up-to-date information and advanced usage scenarios. With practice and experimentation, you’ll be composing intricate, user-friendly layouts that will elevate your React applications to new heights of interactivity and flexibility.
Whether you’re building data-heavy dashboards, complex editing interfaces, or customizable workspaces, Golden Layout provides the foundation for creating truly dynamic and responsive React applications. So start experimenting, and let your creativity flow as you orchestrate beautiful, functional layouts with Golden Layout and React!