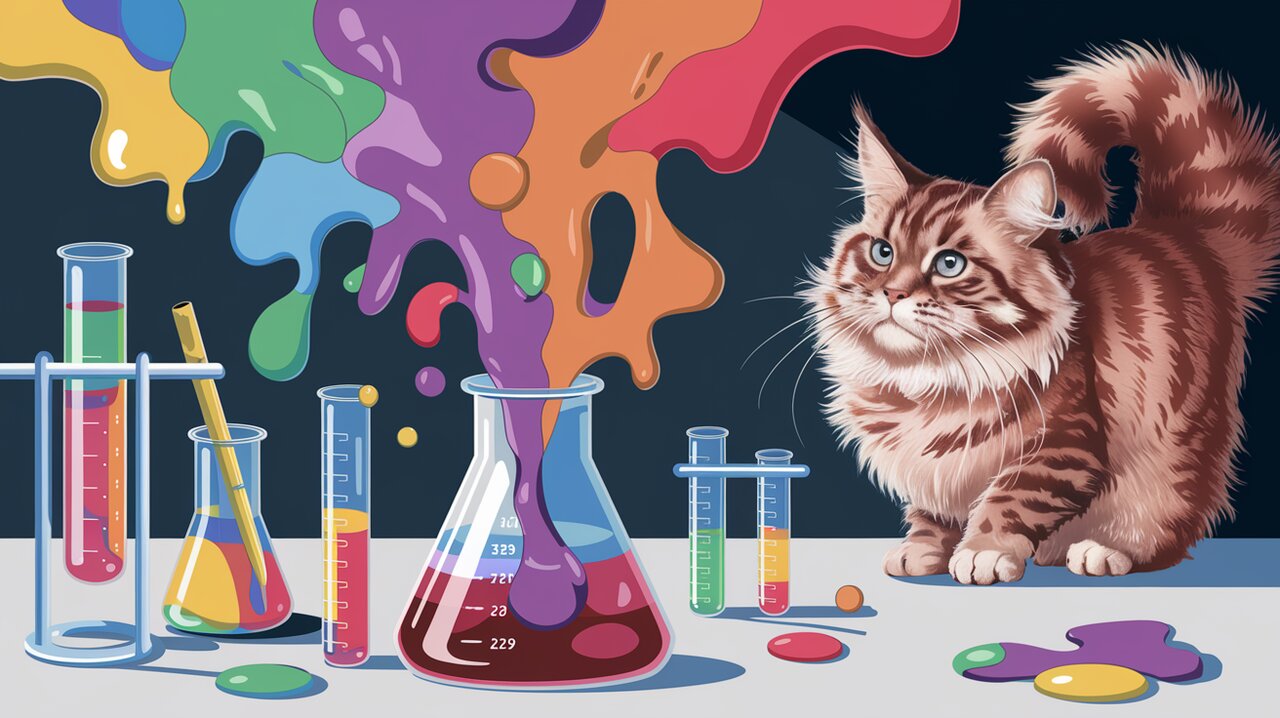
Have you ever wanted to add a touch of fluid, blobby magic to your React applications? Look no further than gooey-react, a tiny yet powerful library that brings the mesmerizing ‘gooey effect’ to your user interfaces. This effect, which has gained popularity through various blog posts and demos, is now easily accessible in React, thanks to this optimized and browser-friendly implementation.
Unleashing the Goo: Features and Benefits
gooey-react isn’t just another animation library. It’s a specialized tool designed to create smooth, blob-like transitions and interactions. Here’s what makes it stand out:
- Crisp and Sharp: Unlike many existing implementations, gooey-react is optimized for clarity, ensuring your gooey effects are as crisp as possible.
- Safari Support: Notorious for its compatibility issues, Safari is fully supported, making your gooey effects accessible to a wider audience.
- Lightweight: At just about 0.5 KB, it won’t bloat your project.
- Flexible: Works with both SVG and regular HTML elements, giving you the freedom to choose your preferred approach.
- Easy Integration: Designed to seamlessly fit into your React workflow.
Getting Started: Installation
To start creating blobby wonders, you first need to install gooey-react. You can do this using npm or yarn:
npm install gooey-react
Or if you prefer yarn:
yarn add gooey-react
Basic Usage: Your First Gooey Effect
Let’s dive into creating your first gooey effect. The basic usage is straightforward and requires minimal setup.
Simple Circles Example
import React from 'react';
import Goo from 'gooey-react';
const GooeyCircles: React.FC = () => (
<Goo>
<svg width="192" height="192">
<circle cx="37%" cy="37%" fill="orchid" r="32" />
<circle cx="63%" cy="63%" fill="mediumorchid" r="32" />
</svg>
</Goo>
);
export default GooeyCircles;
In this example, we’re creating two circles within an SVG, wrapped by the Goo
component. The circles are positioned close enough to demonstrate the gooey effect when they overlap. The cx
and cy
attributes control the position of the circles, while r
sets their radius.
Adding Animation
To make things more interesting, let’s add some simple animation:
import React from 'react';
import Goo from 'gooey-react';
const AnimatedGoo: React.FC = () => (
<Goo>
<svg width="192" height="192">
<g style={{ animation: 'left 4s linear infinite' }}>
<circle
cx="37%"
cy="37%"
fill="orchid"
r="32"
style={{ animation: 'right 1s linear infinite' }}
/>
<circle cx="63%" cy="63%" fill="mediumorchid" r="32" />
</g>
</svg>
</Goo>
);
export default AnimatedGoo;
Here, we’ve added CSS animations to create movement. The outer g
element rotates the entire group, while the first circle has its own rotation. This creates a mesmerizing, fluid motion that showcases the gooey effect beautifully.
Advanced Techniques: Customizing Your Goo
gooey-react offers various ways to customize and fine-tune your gooey effects. Let’s explore some advanced techniques.
Adjusting Intensity
You can control the strength of the gooey effect using the intensity
prop:
import React from 'react';
import Goo from 'gooey-react';
const IntenseGoo: React.FC = () => (
<Goo intensity="strong">
{/* Your SVG content here */}
</Goo>
);
export default IntenseGoo;
The intensity
prop accepts values like “weak”, “medium”, or “strong”, allowing you to fine-tune the blobbing effect to your liking.
Using with HTML Elements
While SVG is recommended for better browser support, you can also use gooey-react with regular HTML elements:
import React from 'react';
import Goo from 'gooey-react';
const HTMLGoo: React.FC = () => (
<Goo style={{ height: '12rem', position: 'relative', width: '12rem' }}>
<div style={{
background: 'sandybrown',
borderRadius: '50%',
height: '4rem',
width: '4rem',
left: '2.5rem',
top: '5.5rem',
position: 'absolute',
}} />
<div style={{
background: 'palevioletred',
borderRadius: '50%',
height: '4rem',
width: '4rem',
left: '5.5rem',
top: '2.5rem',
position: 'absolute',
}} />
</Goo>
);
export default HTMLGoo;
This example creates two overlapping circles using div
elements, demonstrating that the gooey effect works just as well with HTML as it does with SVG.
Combining with Other Animation Libraries
For more complex animations, you can combine gooey-react with other animation libraries. Here’s an example using React Spring:
import React from 'react';
import Goo from 'gooey-react';
import { useSpring, animated } from 'react-spring';
const SpringyGoo: React.FC = () => {
const props = useSpring({
from: { x: 0 },
to: { x: 100 },
config: { duration: 2000 },
loop: true,
});
return (
<Goo>
<svg width="200" height="200">
<animated.circle
cx={props.x}
cy="100"
r="30"
fill="coral"
/>
<circle cx="100" cy="100" r="30" fill="lightblue" />
</svg>
</Goo>
);
};
export default SpringyGoo;
This example uses React Spring to animate the position of one circle, creating a smooth back-and-forth motion that interacts with the static circle, all enhanced by the gooey effect.
Wrapping Up: The Magic of Gooey React
gooey-react opens up a world of creative possibilities for React developers. Whether you’re looking to add subtle, fluid interactions or create eye-catching, blobby animations, this library provides an easy and efficient way to implement the gooey effect in your projects.
By leveraging SVG or HTML elements, customizing the intensity, and combining with other animation techniques, you can create unique and engaging user interfaces that stand out from the crowd. The library’s small size and broad browser support, including Safari, make it an excellent choice for both small projects and large-scale applications.
So why not give your React apps a touch of blobby brilliance? With gooey-react, you’re just a few lines of code away from creating fluid, mesmerizing UI effects that will captivate your users and add that extra layer of polish to your web applications.