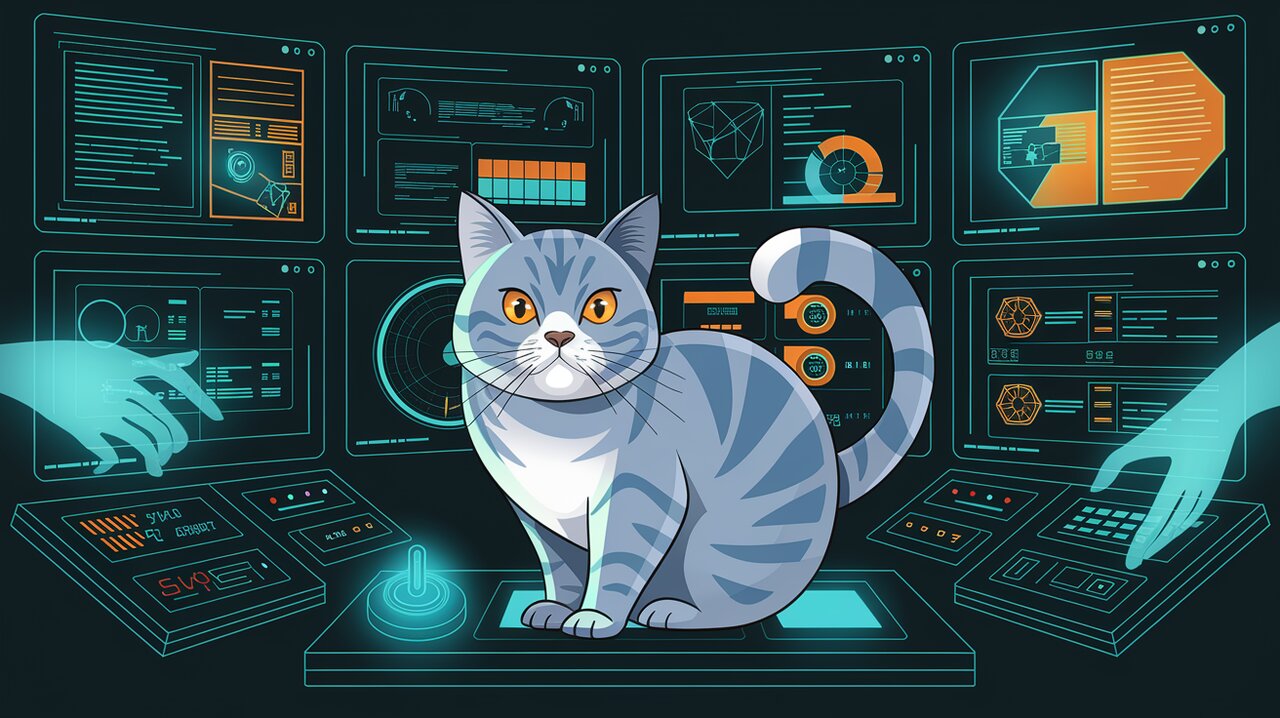
Gridstack.js: Conjuring Dynamic Dashboards with React Magic
Gridstack.js is a powerful library that brings the magic of dynamic, draggable, and resizable grid layouts to your React applications. Whether you’re building complex dashboards or flexible content management systems, Gridstack.js offers the tools you need to create responsive and interactive user interfaces with ease.
Unleashing the Power of Gridstack.js
Gridstack.js empowers developers to create grid-based layouts where elements can be easily dragged, dropped, and resized. This flexibility is particularly useful for dashboard applications where users need to customize their view of data and widgets.
Key Features
- Drag and Drop: Effortlessly rearrange grid items with intuitive drag-and-drop functionality.
- Resizable Widgets: Allow users to resize widgets to their preferred dimensions.
- Responsive Design: Automatically adapt layouts to different screen sizes.
- Nested Grids: Create complex layouts with grids within grids.
- API Control: Programmatically manipulate the grid for dynamic content management.
Getting Started with Gridstack.js in React
To begin your journey with Gridstack.js in React, you’ll need to install the library and its CSS file. Here’s how to get started:
npm install gridstack
Once installed, import the necessary components in your React file:
import { GridStack } from 'gridstack';
import 'gridstack/dist/gridstack.min.css';
Crafting Your First Grid
Let’s create a basic grid with some draggable and resizable items:
import React, { useEffect } from 'react';
import { GridStack } from 'gridstack';
import 'gridstack/dist/gridstack.min.css';
const DashboardGrid: React.FC = () => {
useEffect(() => {
const grid = GridStack.init({
column: 12,
minRow: 1,
cellHeight: 80,
disableOneColumnMode: true,
float: false,
removable: '.trash',
removeTimeout: 100,
acceptWidgets: true
});
// Add widgets programmatically
grid.addWidget({ w: 2, content: 'Widget 1' });
grid.addWidget({ w: 4, content: 'Widget 2' });
grid.addWidget({ w: 2, h: 2, content: 'Widget 3' });
return () => {
grid.destroy();
};
}, []);
return (
<div className="grid-stack">
{/* Grid items will be added here */}
</div>
);
};
export default DashboardGrid;
This code initializes a Gridstack instance with 12 columns and adds three widgets with different sizes. The grid will automatically handle the dragging and resizing of these widgets.
Advanced Grid Customization
Gridstack.js offers a wealth of options for fine-tuning your grid’s behavior. Let’s explore some advanced features:
Responsive Breakpoints
Adapt your grid to different screen sizes with custom breakpoints:
const grid = GridStack.init({
column: 12,
minRow: 1,
cellHeight: 80,
disableOneColumnMode: true,
columnOpts: {
breakpoints: [
{ w: 1200, c: 12 },
{ w: 992, c: 9 },
{ w: 768, c: 6 },
{ w: 576, c: 3 }
]
}
});
This configuration adjusts the number of columns based on the screen width, ensuring your dashboard looks great on all devices.
Saving and Loading Layouts
Implement persistence by saving and loading grid layouts:
// Save the current layout
const saveLayout = () => {
const serializedData = grid.save();
localStorage.setItem('gridLayout', JSON.stringify(serializedData));
};
// Load a saved layout
const loadLayout = () => {
const savedLayout = localStorage.getItem('gridLayout');
if (savedLayout) {
grid.load(JSON.parse(savedLayout));
}
};
This feature allows users to customize their dashboard and return to their preferred layout on subsequent visits.
Enhancing User Experience with Events
Gridstack.js provides a rich set of events that you can leverage to create a more interactive experience:
useEffect(() => {
const grid = GridStack.init();
grid.on('added', (event, items) => {
console.log('New widgets added:', items);
});
grid.on('change', (event, items) => {
console.log('Items changed:', items);
});
grid.on('removed', (event, items) => {
console.log('Widgets removed:', items);
});
// Cleanup
return () => {
grid.off('added');
grid.off('change');
grid.off('removed');
grid.destroy();
};
}, []);
By listening to these events, you can trigger additional actions, update your application state, or send data to a backend service as users interact with the dashboard.
Conclusion
Gridstack.js brings a new level of interactivity and flexibility to React applications, making it an excellent choice for building dynamic dashboards and content management systems. Its intuitive API, combined with React’s component-based architecture, allows developers to create powerful and responsive user interfaces with ease.
As you continue to explore Gridstack.js, you’ll discover even more ways to enhance your React applications. For more insights into React libraries that can complement your dashboard creation, check out our articles on React-Beautiful-DND for drag-and-drop functionality and React-Grid-Layout for alternative grid systems.
With Gridstack.js in your toolkit, you’re well-equipped to conjure dashboards that are not just functional, but truly magical in their flexibility and user experience. Happy coding, and may your dashboards be ever dynamic and delightful!